parent
6de8e2df0a
commit
2b1299b408
@ -0,0 +1,7 @@
|
||||
<?php
|
||||
|
||||
// autoload.php @generated by Composer
|
||||
|
||||
require_once __DIR__ . '/composer/autoload_real.php';
|
||||
|
||||
return ComposerAutoloaderInitc184f419bfb1b5c35adb1bc36e45b15e::getLoader();
|
@ -0,0 +1,14 @@
|
||||
#!/usr/bin/env sh
|
||||
|
||||
dir=$(cd "${0%[/\\]*}" > /dev/null; cd "../phpunit/phpunit" && pwd)
|
||||
|
||||
if [ -d /proc/cygdrive ]; then
|
||||
case $(which php) in
|
||||
$(readlink -n /proc/cygdrive)/*)
|
||||
# We are in Cygwin using Windows php, so the path must be translated
|
||||
dir=$(cygpath -m "$dir");
|
||||
;;
|
||||
esac
|
||||
fi
|
||||
|
||||
"${dir}/phpunit" "$@"
|
@ -0,0 +1,4 @@
|
||||
@ECHO OFF
|
||||
setlocal DISABLEDELAYEDEXPANSION
|
||||
SET BIN_TARGET=%~dp0/../phpunit/phpunit/phpunit
|
||||
php "%BIN_TARGET%" %*
|
@ -0,0 +1,479 @@
|
||||
<?php
|
||||
|
||||
/*
|
||||
* This file is part of Composer.
|
||||
*
|
||||
* (c) Nils Adermann <naderman@naderman.de>
|
||||
* Jordi Boggiano <j.boggiano@seld.be>
|
||||
*
|
||||
* For the full copyright and license information, please view the LICENSE
|
||||
* file that was distributed with this source code.
|
||||
*/
|
||||
|
||||
namespace Composer\Autoload;
|
||||
|
||||
/**
|
||||
* ClassLoader implements a PSR-0, PSR-4 and classmap class loader.
|
||||
*
|
||||
* $loader = new \Composer\Autoload\ClassLoader();
|
||||
*
|
||||
* // register classes with namespaces
|
||||
* $loader->add('Symfony\Component', __DIR__.'/component');
|
||||
* $loader->add('Symfony', __DIR__.'/framework');
|
||||
*
|
||||
* // activate the autoloader
|
||||
* $loader->register();
|
||||
*
|
||||
* // to enable searching the include path (eg. for PEAR packages)
|
||||
* $loader->setUseIncludePath(true);
|
||||
*
|
||||
* In this example, if you try to use a class in the Symfony\Component
|
||||
* namespace or one of its children (Symfony\Component\Console for instance),
|
||||
* the autoloader will first look for the class under the component/
|
||||
* directory, and it will then fallback to the framework/ directory if not
|
||||
* found before giving up.
|
||||
*
|
||||
* This class is loosely based on the Symfony UniversalClassLoader.
|
||||
*
|
||||
* @author Fabien Potencier <fabien@symfony.com>
|
||||
* @author Jordi Boggiano <j.boggiano@seld.be>
|
||||
* @see https://www.php-fig.org/psr/psr-0/
|
||||
* @see https://www.php-fig.org/psr/psr-4/
|
||||
*/
|
||||
class ClassLoader
|
||||
{
|
||||
private $vendorDir;
|
||||
|
||||
// PSR-4
|
||||
private $prefixLengthsPsr4 = array();
|
||||
private $prefixDirsPsr4 = array();
|
||||
private $fallbackDirsPsr4 = array();
|
||||
|
||||
// PSR-0
|
||||
private $prefixesPsr0 = array();
|
||||
private $fallbackDirsPsr0 = array();
|
||||
|
||||
private $useIncludePath = false;
|
||||
private $classMap = array();
|
||||
private $classMapAuthoritative = false;
|
||||
private $missingClasses = array();
|
||||
private $apcuPrefix;
|
||||
|
||||
private static $registeredLoaders = array();
|
||||
|
||||
public function __construct($vendorDir = null)
|
||||
{
|
||||
$this->vendorDir = $vendorDir;
|
||||
}
|
||||
|
||||
public function getPrefixes()
|
||||
{
|
||||
if (!empty($this->prefixesPsr0)) {
|
||||
return call_user_func_array('array_merge', array_values($this->prefixesPsr0));
|
||||
}
|
||||
|
||||
return array();
|
||||
}
|
||||
|
||||
public function getPrefixesPsr4()
|
||||
{
|
||||
return $this->prefixDirsPsr4;
|
||||
}
|
||||
|
||||
public function getFallbackDirs()
|
||||
{
|
||||
return $this->fallbackDirsPsr0;
|
||||
}
|
||||
|
||||
public function getFallbackDirsPsr4()
|
||||
{
|
||||
return $this->fallbackDirsPsr4;
|
||||
}
|
||||
|
||||
public function getClassMap()
|
||||
{
|
||||
return $this->classMap;
|
||||
}
|
||||
|
||||
/**
|
||||
* @param array $classMap Class to filename map
|
||||
*/
|
||||
public function addClassMap(array $classMap)
|
||||
{
|
||||
if ($this->classMap) {
|
||||
$this->classMap = array_merge($this->classMap, $classMap);
|
||||
} else {
|
||||
$this->classMap = $classMap;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Registers a set of PSR-0 directories for a given prefix, either
|
||||
* appending or prepending to the ones previously set for this prefix.
|
||||
*
|
||||
* @param string $prefix The prefix
|
||||
* @param array|string $paths The PSR-0 root directories
|
||||
* @param bool $prepend Whether to prepend the directories
|
||||
*/
|
||||
public function add($prefix, $paths, $prepend = false)
|
||||
{
|
||||
if (!$prefix) {
|
||||
if ($prepend) {
|
||||
$this->fallbackDirsPsr0 = array_merge(
|
||||
(array) $paths,
|
||||
$this->fallbackDirsPsr0
|
||||
);
|
||||
} else {
|
||||
$this->fallbackDirsPsr0 = array_merge(
|
||||
$this->fallbackDirsPsr0,
|
||||
(array) $paths
|
||||
);
|
||||
}
|
||||
|
||||
return;
|
||||
}
|
||||
|
||||
$first = $prefix[0];
|
||||
if (!isset($this->prefixesPsr0[$first][$prefix])) {
|
||||
$this->prefixesPsr0[$first][$prefix] = (array) $paths;
|
||||
|
||||
return;
|
||||
}
|
||||
if ($prepend) {
|
||||
$this->prefixesPsr0[$first][$prefix] = array_merge(
|
||||
(array) $paths,
|
||||
$this->prefixesPsr0[$first][$prefix]
|
||||
);
|
||||
} else {
|
||||
$this->prefixesPsr0[$first][$prefix] = array_merge(
|
||||
$this->prefixesPsr0[$first][$prefix],
|
||||
(array) $paths
|
||||
);
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Registers a set of PSR-4 directories for a given namespace, either
|
||||
* appending or prepending to the ones previously set for this namespace.
|
||||
*
|
||||
* @param string $prefix The prefix/namespace, with trailing '\\'
|
||||
* @param array|string $paths The PSR-4 base directories
|
||||
* @param bool $prepend Whether to prepend the directories
|
||||
*
|
||||
* @throws \InvalidArgumentException
|
||||
*/
|
||||
public function addPsr4($prefix, $paths, $prepend = false)
|
||||
{
|
||||
if (!$prefix) {
|
||||
// Register directories for the root namespace.
|
||||
if ($prepend) {
|
||||
$this->fallbackDirsPsr4 = array_merge(
|
||||
(array) $paths,
|
||||
$this->fallbackDirsPsr4
|
||||
);
|
||||
} else {
|
||||
$this->fallbackDirsPsr4 = array_merge(
|
||||
$this->fallbackDirsPsr4,
|
||||
(array) $paths
|
||||
);
|
||||
}
|
||||
} elseif (!isset($this->prefixDirsPsr4[$prefix])) {
|
||||
// Register directories for a new namespace.
|
||||
$length = strlen($prefix);
|
||||
if ('\\' !== $prefix[$length - 1]) {
|
||||
throw new \InvalidArgumentException("A non-empty PSR-4 prefix must end with a namespace separator.");
|
||||
}
|
||||
$this->prefixLengthsPsr4[$prefix[0]][$prefix] = $length;
|
||||
$this->prefixDirsPsr4[$prefix] = (array) $paths;
|
||||
} elseif ($prepend) {
|
||||
// Prepend directories for an already registered namespace.
|
||||
$this->prefixDirsPsr4[$prefix] = array_merge(
|
||||
(array) $paths,
|
||||
$this->prefixDirsPsr4[$prefix]
|
||||
);
|
||||
} else {
|
||||
// Append directories for an already registered namespace.
|
||||
$this->prefixDirsPsr4[$prefix] = array_merge(
|
||||
$this->prefixDirsPsr4[$prefix],
|
||||
(array) $paths
|
||||
);
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Registers a set of PSR-0 directories for a given prefix,
|
||||
* replacing any others previously set for this prefix.
|
||||
*
|
||||
* @param string $prefix The prefix
|
||||
* @param array|string $paths The PSR-0 base directories
|
||||
*/
|
||||
public function set($prefix, $paths)
|
||||
{
|
||||
if (!$prefix) {
|
||||
$this->fallbackDirsPsr0 = (array) $paths;
|
||||
} else {
|
||||
$this->prefixesPsr0[$prefix[0]][$prefix] = (array) $paths;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Registers a set of PSR-4 directories for a given namespace,
|
||||
* replacing any others previously set for this namespace.
|
||||
*
|
||||
* @param string $prefix The prefix/namespace, with trailing '\\'
|
||||
* @param array|string $paths The PSR-4 base directories
|
||||
*
|
||||
* @throws \InvalidArgumentException
|
||||
*/
|
||||
public function setPsr4($prefix, $paths)
|
||||
{
|
||||
if (!$prefix) {
|
||||
$this->fallbackDirsPsr4 = (array) $paths;
|
||||
} else {
|
||||
$length = strlen($prefix);
|
||||
if ('\\' !== $prefix[$length - 1]) {
|
||||
throw new \InvalidArgumentException("A non-empty PSR-4 prefix must end with a namespace separator.");
|
||||
}
|
||||
$this->prefixLengthsPsr4[$prefix[0]][$prefix] = $length;
|
||||
$this->prefixDirsPsr4[$prefix] = (array) $paths;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Turns on searching the include path for class files.
|
||||
*
|
||||
* @param bool $useIncludePath
|
||||
*/
|
||||
public function setUseIncludePath($useIncludePath)
|
||||
{
|
||||
$this->useIncludePath = $useIncludePath;
|
||||
}
|
||||
|
||||
/**
|
||||
* Can be used to check if the autoloader uses the include path to check
|
||||
* for classes.
|
||||
*
|
||||
* @return bool
|
||||
*/
|
||||
public function getUseIncludePath()
|
||||
{
|
||||
return $this->useIncludePath;
|
||||
}
|
||||
|
||||
/**
|
||||
* Turns off searching the prefix and fallback directories for classes
|
||||
* that have not been registered with the class map.
|
||||
*
|
||||
* @param bool $classMapAuthoritative
|
||||
*/
|
||||
public function setClassMapAuthoritative($classMapAuthoritative)
|
||||
{
|
||||
$this->classMapAuthoritative = $classMapAuthoritative;
|
||||
}
|
||||
|
||||
/**
|
||||
* Should class lookup fail if not found in the current class map?
|
||||
*
|
||||
* @return bool
|
||||
*/
|
||||
public function isClassMapAuthoritative()
|
||||
{
|
||||
return $this->classMapAuthoritative;
|
||||
}
|
||||
|
||||
/**
|
||||
* APCu prefix to use to cache found/not-found classes, if the extension is enabled.
|
||||
*
|
||||
* @param string|null $apcuPrefix
|
||||
*/
|
||||
public function setApcuPrefix($apcuPrefix)
|
||||
{
|
||||
$this->apcuPrefix = function_exists('apcu_fetch') && filter_var(ini_get('apc.enabled'), FILTER_VALIDATE_BOOLEAN) ? $apcuPrefix : null;
|
||||
}
|
||||
|
||||
/**
|
||||
* The APCu prefix in use, or null if APCu caching is not enabled.
|
||||
*
|
||||
* @return string|null
|
||||
*/
|
||||
public function getApcuPrefix()
|
||||
{
|
||||
return $this->apcuPrefix;
|
||||
}
|
||||
|
||||
/**
|
||||
* Registers this instance as an autoloader.
|
||||
*
|
||||
* @param bool $prepend Whether to prepend the autoloader or not
|
||||
*/
|
||||
public function register($prepend = false)
|
||||
{
|
||||
spl_autoload_register(array($this, 'loadClass'), true, $prepend);
|
||||
|
||||
if (null === $this->vendorDir) {
|
||||
return;
|
||||
}
|
||||
|
||||
if ($prepend) {
|
||||
self::$registeredLoaders = array($this->vendorDir => $this) + self::$registeredLoaders;
|
||||
} else {
|
||||
unset(self::$registeredLoaders[$this->vendorDir]);
|
||||
self::$registeredLoaders[$this->vendorDir] = $this;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Unregisters this instance as an autoloader.
|
||||
*/
|
||||
public function unregister()
|
||||
{
|
||||
spl_autoload_unregister(array($this, 'loadClass'));
|
||||
|
||||
if (null !== $this->vendorDir) {
|
||||
unset(self::$registeredLoaders[$this->vendorDir]);
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Loads the given class or interface.
|
||||
*
|
||||
* @param string $class The name of the class
|
||||
* @return bool|null True if loaded, null otherwise
|
||||
*/
|
||||
public function loadClass($class)
|
||||
{
|
||||
if ($file = $this->findFile($class)) {
|
||||
includeFile($file);
|
||||
|
||||
return true;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Finds the path to the file where the class is defined.
|
||||
*
|
||||
* @param string $class The name of the class
|
||||
*
|
||||
* @return string|false The path if found, false otherwise
|
||||
*/
|
||||
public function findFile($class)
|
||||
{
|
||||
// class map lookup
|
||||
if (isset($this->classMap[$class])) {
|
||||
return $this->classMap[$class];
|
||||
}
|
||||
if ($this->classMapAuthoritative || isset($this->missingClasses[$class])) {
|
||||
return false;
|
||||
}
|
||||
if (null !== $this->apcuPrefix) {
|
||||
$file = apcu_fetch($this->apcuPrefix.$class, $hit);
|
||||
if ($hit) {
|
||||
return $file;
|
||||
}
|
||||
}
|
||||
|
||||
$file = $this->findFileWithExtension($class, '.php');
|
||||
|
||||
// Search for Hack files if we are running on HHVM
|
||||
if (false === $file && defined('HHVM_VERSION')) {
|
||||
$file = $this->findFileWithExtension($class, '.hh');
|
||||
}
|
||||
|
||||
if (null !== $this->apcuPrefix) {
|
||||
apcu_add($this->apcuPrefix.$class, $file);
|
||||
}
|
||||
|
||||
if (false === $file) {
|
||||
// Remember that this class does not exist.
|
||||
$this->missingClasses[$class] = true;
|
||||
}
|
||||
|
||||
return $file;
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns the currently registered loaders indexed by their corresponding vendor directories.
|
||||
*
|
||||
* @return self[]
|
||||
*/
|
||||
public static function getRegisteredLoaders()
|
||||
{
|
||||
return self::$registeredLoaders;
|
||||
}
|
||||
|
||||
private function findFileWithExtension($class, $ext)
|
||||
{
|
||||
// PSR-4 lookup
|
||||
$logicalPathPsr4 = strtr($class, '\\', DIRECTORY_SEPARATOR) . $ext;
|
||||
|
||||
$first = $class[0];
|
||||
if (isset($this->prefixLengthsPsr4[$first])) {
|
||||
$subPath = $class;
|
||||
while (false !== $lastPos = strrpos($subPath, '\\')) {
|
||||
$subPath = substr($subPath, 0, $lastPos);
|
||||
$search = $subPath . '\\';
|
||||
if (isset($this->prefixDirsPsr4[$search])) {
|
||||
$pathEnd = DIRECTORY_SEPARATOR . substr($logicalPathPsr4, $lastPos + 1);
|
||||
foreach ($this->prefixDirsPsr4[$search] as $dir) {
|
||||
if (file_exists($file = $dir . $pathEnd)) {
|
||||
return $file;
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
// PSR-4 fallback dirs
|
||||
foreach ($this->fallbackDirsPsr4 as $dir) {
|
||||
if (file_exists($file = $dir . DIRECTORY_SEPARATOR . $logicalPathPsr4)) {
|
||||
return $file;
|
||||
}
|
||||
}
|
||||
|
||||
// PSR-0 lookup
|
||||
if (false !== $pos = strrpos($class, '\\')) {
|
||||
// namespaced class name
|
||||
$logicalPathPsr0 = substr($logicalPathPsr4, 0, $pos + 1)
|
||||
. strtr(substr($logicalPathPsr4, $pos + 1), '_', DIRECTORY_SEPARATOR);
|
||||
} else {
|
||||
// PEAR-like class name
|
||||
$logicalPathPsr0 = strtr($class, '_', DIRECTORY_SEPARATOR) . $ext;
|
||||
}
|
||||
|
||||
if (isset($this->prefixesPsr0[$first])) {
|
||||
foreach ($this->prefixesPsr0[$first] as $prefix => $dirs) {
|
||||
if (0 === strpos($class, $prefix)) {
|
||||
foreach ($dirs as $dir) {
|
||||
if (file_exists($file = $dir . DIRECTORY_SEPARATOR . $logicalPathPsr0)) {
|
||||
return $file;
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
// PSR-0 fallback dirs
|
||||
foreach ($this->fallbackDirsPsr0 as $dir) {
|
||||
if (file_exists($file = $dir . DIRECTORY_SEPARATOR . $logicalPathPsr0)) {
|
||||
return $file;
|
||||
}
|
||||
}
|
||||
|
||||
// PSR-0 include paths.
|
||||
if ($this->useIncludePath && $file = stream_resolve_include_path($logicalPathPsr0)) {
|
||||
return $file;
|
||||
}
|
||||
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Scope isolated include.
|
||||
*
|
||||
* Prevents access to $this/self from included files.
|
||||
*/
|
||||
function includeFile($file)
|
||||
{
|
||||
include $file;
|
||||
}
|
@ -0,0 +1,679 @@
|
||||
<?php
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
namespace Composer;
|
||||
|
||||
use Composer\Autoload\ClassLoader;
|
||||
use Composer\Semver\VersionParser;
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
class InstalledVersions
|
||||
{
|
||||
private static $installed = array (
|
||||
'root' =>
|
||||
array (
|
||||
'pretty_version' => 'dev-master',
|
||||
'version' => 'dev-master',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '7088f600d8002ab34d9457e48bfdaf2050cebf68',
|
||||
'name' => 'topthink/think',
|
||||
),
|
||||
'versions' =>
|
||||
array (
|
||||
'doctrine/instantiator' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.0',
|
||||
'version' => '1.4.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'd56bf6102915de5702778fe20f2de3b2fe570b5b',
|
||||
),
|
||||
'markbaker/complex' =>
|
||||
array (
|
||||
'pretty_version' => '1.5.0',
|
||||
'version' => '1.5.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'c3131244e29c08d44fefb49e0dd35021e9e39dd2',
|
||||
),
|
||||
'markbaker/matrix' =>
|
||||
array (
|
||||
'pretty_version' => '1.2.3',
|
||||
'version' => '1.2.3.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '44bb1ab01811116f01fe216ab37d921dccc6c10d',
|
||||
),
|
||||
'pclzip/pclzip' =>
|
||||
array (
|
||||
'pretty_version' => '2.8.2',
|
||||
'version' => '2.8.2.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '19dd1de9d3f5fc4d7d70175b4c344dee329f45fd',
|
||||
),
|
||||
'phpdocumentor/reflection-common' =>
|
||||
array (
|
||||
'pretty_version' => '2.2.0',
|
||||
'version' => '2.2.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '1d01c49d4ed62f25aa84a747ad35d5a16924662b',
|
||||
),
|
||||
'phpdocumentor/reflection-docblock' =>
|
||||
array (
|
||||
'pretty_version' => '5.2.2',
|
||||
'version' => '5.2.2.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '069a785b2141f5bcf49f3e353548dc1cce6df556',
|
||||
),
|
||||
'phpdocumentor/type-resolver' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.0',
|
||||
'version' => '1.4.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '6a467b8989322d92aa1c8bf2bebcc6e5c2ba55c0',
|
||||
),
|
||||
'phpoffice/common' =>
|
||||
array (
|
||||
'pretty_version' => '0.2.9',
|
||||
'version' => '0.2.9.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'edb5d32b1e3400a35a5c91e2539ed6f6ce925e4d',
|
||||
),
|
||||
'phpoffice/phpexcel' =>
|
||||
array (
|
||||
'pretty_version' => '1.8.0',
|
||||
'version' => '1.8.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'e69a5e4d0ffa7fb6f171859e0a04346e580df30b',
|
||||
),
|
||||
'phpoffice/phpspreadsheet' =>
|
||||
array (
|
||||
'pretty_version' => '1.8.0',
|
||||
'version' => '1.8.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '0e6238c69e863b58aeece61e48ea032696c6dccd',
|
||||
),
|
||||
'phpoffice/phpword' =>
|
||||
array (
|
||||
'pretty_version' => '0.17.0',
|
||||
'version' => '0.17.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'b8346af548d399acd9e30fc76ab0c55c2fec03a5',
|
||||
),
|
||||
'phpspec/prophecy' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.10.3',
|
||||
'version' => '1.10.3.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '451c3cd1418cf640de218914901e51b064abb093',
|
||||
),
|
||||
'phpunit/php-code-coverage' =>
|
||||
array (
|
||||
'pretty_version' => '2.2.4',
|
||||
'version' => '2.2.4.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'eabf68b476ac7d0f73793aada060f1c1a9bf8979',
|
||||
),
|
||||
'phpunit/php-file-iterator' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.5',
|
||||
'version' => '1.4.5.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '730b01bc3e867237eaac355e06a36b85dd93a8b4',
|
||||
),
|
||||
'phpunit/php-text-template' =>
|
||||
array (
|
||||
'pretty_version' => '1.2.1',
|
||||
'version' => '1.2.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '31f8b717e51d9a2afca6c9f046f5d69fc27c8686',
|
||||
),
|
||||
'phpunit/php-timer' =>
|
||||
array (
|
||||
'pretty_version' => '1.0.9',
|
||||
'version' => '1.0.9.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '3dcf38ca72b158baf0bc245e9184d3fdffa9c46f',
|
||||
),
|
||||
'phpunit/php-token-stream' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.12',
|
||||
'version' => '1.4.12.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '1ce90ba27c42e4e44e6d8458241466380b51fa16',
|
||||
),
|
||||
'phpunit/phpunit' =>
|
||||
array (
|
||||
'pretty_version' => '4.8.36',
|
||||
'version' => '4.8.36.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '46023de9a91eec7dfb06cc56cb4e260017298517',
|
||||
),
|
||||
'phpunit/phpunit-mock-objects' =>
|
||||
array (
|
||||
'pretty_version' => '2.3.8',
|
||||
'version' => '2.3.8.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'ac8e7a3db35738d56ee9a76e78a4e03d97628983',
|
||||
),
|
||||
'psr/simple-cache' =>
|
||||
array (
|
||||
'pretty_version' => '1.0.1',
|
||||
'version' => '1.0.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '408d5eafb83c57f6365a3ca330ff23aa4a5fa39b',
|
||||
),
|
||||
'sebastian/comparator' =>
|
||||
array (
|
||||
'pretty_version' => '1.2.4',
|
||||
'version' => '1.2.4.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '2b7424b55f5047b47ac6e5ccb20b2aea4011d9be',
|
||||
),
|
||||
'sebastian/diff' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.3',
|
||||
'version' => '1.4.3.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '7f066a26a962dbe58ddea9f72a4e82874a3975a4',
|
||||
),
|
||||
'sebastian/environment' =>
|
||||
array (
|
||||
'pretty_version' => '1.3.8',
|
||||
'version' => '1.3.8.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'be2c607e43ce4c89ecd60e75c6a85c126e754aea',
|
||||
),
|
||||
'sebastian/exporter' =>
|
||||
array (
|
||||
'pretty_version' => '1.2.2',
|
||||
'version' => '1.2.2.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '42c4c2eec485ee3e159ec9884f95b431287edde4',
|
||||
),
|
||||
'sebastian/global-state' =>
|
||||
array (
|
||||
'pretty_version' => '1.1.1',
|
||||
'version' => '1.1.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'bc37d50fea7d017d3d340f230811c9f1d7280af4',
|
||||
),
|
||||
'sebastian/recursion-context' =>
|
||||
array (
|
||||
'pretty_version' => '1.0.5',
|
||||
'version' => '1.0.5.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'b19cc3298482a335a95f3016d2f8a6950f0fbcd7',
|
||||
),
|
||||
'sebastian/version' =>
|
||||
array (
|
||||
'pretty_version' => '1.0.6',
|
||||
'version' => '1.0.6.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '58b3a85e7999757d6ad81c787a1fbf5ff6c628c6',
|
||||
),
|
||||
'symfony/dom-crawler' =>
|
||||
array (
|
||||
'pretty_version' => 'v2.8.52',
|
||||
'version' => '2.8.52.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '2cdc7d3909eea6f982a6298d2e9ab7db01b6403c',
|
||||
),
|
||||
'symfony/polyfill-ctype' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.22.1',
|
||||
'version' => '1.22.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'c6c942b1ac76c82448322025e084cadc56048b4e',
|
||||
),
|
||||
'symfony/polyfill-mbstring' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.22.1',
|
||||
'version' => '1.22.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '5232de97ee3b75b0360528dae24e73db49566ab1',
|
||||
),
|
||||
'symfony/yaml' =>
|
||||
array (
|
||||
'pretty_version' => 'v3.4.47',
|
||||
'version' => '3.4.47.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '88289caa3c166321883f67fe5130188ebbb47094',
|
||||
),
|
||||
'topthink/framework' =>
|
||||
array (
|
||||
'pretty_version' => 'v5.0.24',
|
||||
'version' => '5.0.24.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'c255c22b2f5fa30f320ecf6c1d29f7740eb3e8be',
|
||||
),
|
||||
'topthink/think' =>
|
||||
array (
|
||||
'pretty_version' => 'dev-master',
|
||||
'version' => 'dev-master',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '7088f600d8002ab34d9457e48bfdaf2050cebf68',
|
||||
),
|
||||
'topthink/think-helper' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.7',
|
||||
'version' => '1.0.7.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '5f92178606c8ce131d36b37a57c58eb71e55f019',
|
||||
),
|
||||
'topthink/think-image' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.7',
|
||||
'version' => '1.0.7.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '8586cf47f117481c6d415b20f7dedf62e79d5512',
|
||||
),
|
||||
'topthink/think-installer' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.14',
|
||||
'version' => '1.0.14.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'eae1740ac264a55c06134b6685dfb9f837d004d1',
|
||||
),
|
||||
'topthink/think-migration' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.1.1',
|
||||
'version' => '1.1.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '8e489f8d38a39876690c0e00fcf9a54ae92c4d3d',
|
||||
),
|
||||
'topthink/think-mongo' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.8.5',
|
||||
'version' => '1.8.5.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '657cc79bd5f090a58b0cc83776073dd69c83a3d1',
|
||||
),
|
||||
'topthink/think-queue' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.1.6',
|
||||
'version' => '1.1.6.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '250650eb0e8ea5af4cfdc7ae46f3f4e0a24ac245',
|
||||
),
|
||||
'topthink/think-sae' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.1',
|
||||
'version' => '1.1.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'e31ee4ec073c0ffc5dbc7292f8268661e5265091',
|
||||
),
|
||||
'topthink/think-testing' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.6',
|
||||
'version' => '1.0.6.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'e89794e5c58aa5587f7b08038e9468150870b185',
|
||||
),
|
||||
'topthink/think-worker' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.0',
|
||||
'version' => '1.0.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'f48ca8e5d8324aace15cdbf0f3de751397ef803f',
|
||||
),
|
||||
'webmozart/assert' =>
|
||||
array (
|
||||
'pretty_version' => '1.10.0',
|
||||
'version' => '1.10.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '6964c76c7804814a842473e0c8fd15bab0f18e25',
|
||||
),
|
||||
'workerman/workerman' =>
|
||||
array (
|
||||
'pretty_version' => 'v3.5.31',
|
||||
'version' => '3.5.31.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'b73ddc45b3c7299f330923a2bde23ca6e974fd96',
|
||||
),
|
||||
'zendframework/zend-escaper' =>
|
||||
array (
|
||||
'pretty_version' => '2.6.1',
|
||||
'version' => '2.6.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '3801caa21b0ca6aca57fa1c42b08d35c395ebd5f',
|
||||
),
|
||||
),
|
||||
);
|
||||
private static $canGetVendors;
|
||||
private static $installedByVendor = array();
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function getInstalledPackages()
|
||||
{
|
||||
$packages = array();
|
||||
foreach (self::getInstalled() as $installed) {
|
||||
$packages[] = array_keys($installed['versions']);
|
||||
}
|
||||
|
||||
|
||||
if (1 === \count($packages)) {
|
||||
return $packages[0];
|
||||
}
|
||||
|
||||
return array_keys(array_flip(\call_user_func_array('array_merge', $packages)));
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function isInstalled($packageName)
|
||||
{
|
||||
foreach (self::getInstalled() as $installed) {
|
||||
if (isset($installed['versions'][$packageName])) {
|
||||
return true;
|
||||
}
|
||||
}
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function satisfies(VersionParser $parser, $packageName, $constraint)
|
||||
{
|
||||
$constraint = $parser->parseConstraints($constraint);
|
||||
$provided = $parser->parseConstraints(self::getVersionRanges($packageName));
|
||||
|
||||
return $provided->matches($constraint);
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function getVersionRanges($packageName)
|
||||
{
|
||||
foreach (self::getInstalled() as $installed) {
|
||||
if (!isset($installed['versions'][$packageName])) {
|
||||
continue;
|
||||
}
|
||||
|
||||
$ranges = array();
|
||||
if (isset($installed['versions'][$packageName]['pretty_version'])) {
|
||||
$ranges[] = $installed['versions'][$packageName]['pretty_version'];
|
||||
}
|
||||
if (array_key_exists('aliases', $installed['versions'][$packageName])) {
|
||||
$ranges = array_merge($ranges, $installed['versions'][$packageName]['aliases']);
|
||||
}
|
||||
if (array_key_exists('replaced', $installed['versions'][$packageName])) {
|
||||
$ranges = array_merge($ranges, $installed['versions'][$packageName]['replaced']);
|
||||
}
|
||||
if (array_key_exists('provided', $installed['versions'][$packageName])) {
|
||||
$ranges = array_merge($ranges, $installed['versions'][$packageName]['provided']);
|
||||
}
|
||||
|
||||
return implode(' || ', $ranges);
|
||||
}
|
||||
|
||||
throw new \OutOfBoundsException('Package "' . $packageName . '" is not installed');
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function getVersion($packageName)
|
||||
{
|
||||
foreach (self::getInstalled() as $installed) {
|
||||
if (!isset($installed['versions'][$packageName])) {
|
||||
continue;
|
||||
}
|
||||
|
||||
if (!isset($installed['versions'][$packageName]['version'])) {
|
||||
return null;
|
||||
}
|
||||
|
||||
return $installed['versions'][$packageName]['version'];
|
||||
}
|
||||
|
||||
throw new \OutOfBoundsException('Package "' . $packageName . '" is not installed');
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function getPrettyVersion($packageName)
|
||||
{
|
||||
foreach (self::getInstalled() as $installed) {
|
||||
if (!isset($installed['versions'][$packageName])) {
|
||||
continue;
|
||||
}
|
||||
|
||||
if (!isset($installed['versions'][$packageName]['pretty_version'])) {
|
||||
return null;
|
||||
}
|
||||
|
||||
return $installed['versions'][$packageName]['pretty_version'];
|
||||
}
|
||||
|
||||
throw new \OutOfBoundsException('Package "' . $packageName . '" is not installed');
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function getReference($packageName)
|
||||
{
|
||||
foreach (self::getInstalled() as $installed) {
|
||||
if (!isset($installed['versions'][$packageName])) {
|
||||
continue;
|
||||
}
|
||||
|
||||
if (!isset($installed['versions'][$packageName]['reference'])) {
|
||||
return null;
|
||||
}
|
||||
|
||||
return $installed['versions'][$packageName]['reference'];
|
||||
}
|
||||
|
||||
throw new \OutOfBoundsException('Package "' . $packageName . '" is not installed');
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function getRootPackage()
|
||||
{
|
||||
$installed = self::getInstalled();
|
||||
|
||||
return $installed[0]['root'];
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function getRawData()
|
||||
{
|
||||
return self::$installed;
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
public static function reload($data)
|
||||
{
|
||||
self::$installed = $data;
|
||||
self::$installedByVendor = array();
|
||||
}
|
||||
|
||||
|
||||
|
||||
|
||||
private static function getInstalled()
|
||||
{
|
||||
if (null === self::$canGetVendors) {
|
||||
self::$canGetVendors = method_exists('Composer\Autoload\ClassLoader', 'getRegisteredLoaders');
|
||||
}
|
||||
|
||||
$installed = array();
|
||||
|
||||
if (self::$canGetVendors) {
|
||||
foreach (ClassLoader::getRegisteredLoaders() as $vendorDir => $loader) {
|
||||
if (isset(self::$installedByVendor[$vendorDir])) {
|
||||
$installed[] = self::$installedByVendor[$vendorDir];
|
||||
} elseif (is_file($vendorDir.'/composer/installed.php')) {
|
||||
$installed[] = self::$installedByVendor[$vendorDir] = require $vendorDir.'/composer/installed.php';
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
$installed[] = self::$installed;
|
||||
|
||||
return $installed;
|
||||
}
|
||||
}
|
@ -0,0 +1,21 @@
|
||||
|
||||
Copyright (c) Nils Adermann, Jordi Boggiano
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy
|
||||
of this software and associated documentation files (the "Software"), to deal
|
||||
in the Software without restriction, including without limitation the rights
|
||||
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the Software is furnished
|
||||
to do so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
||||
THE SOFTWARE.
|
||||
|
@ -0,0 +1,456 @@
|
||||
<?php
|
||||
|
||||
// autoload_classmap.php @generated by Composer
|
||||
|
||||
$vendorDir = dirname(dirname(__FILE__));
|
||||
$baseDir = dirname($vendorDir);
|
||||
|
||||
return array(
|
||||
'Composer\\InstalledVersions' => $vendorDir . '/composer/InstalledVersions.php',
|
||||
'File_Iterator' => $vendorDir . '/phpunit/php-file-iterator/src/Iterator.php',
|
||||
'File_Iterator_Facade' => $vendorDir . '/phpunit/php-file-iterator/src/Facade.php',
|
||||
'File_Iterator_Factory' => $vendorDir . '/phpunit/php-file-iterator/src/Factory.php',
|
||||
'PHPUnit\\Framework\\Assert' => $vendorDir . '/phpunit/phpunit/src/ForwardCompatibility/Assert.php',
|
||||
'PHPUnit\\Framework\\AssertionFailedError' => $vendorDir . '/phpunit/phpunit/src/ForwardCompatibility/AssertionFailedError.php',
|
||||
'PHPUnit\\Framework\\BaseTestListener' => $vendorDir . '/phpunit/phpunit/src/ForwardCompatibility/BaseTestListener.php',
|
||||
'PHPUnit\\Framework\\Test' => $vendorDir . '/phpunit/phpunit/src/ForwardCompatibility/Test.php',
|
||||
'PHPUnit\\Framework\\TestCase' => $vendorDir . '/phpunit/phpunit/src/ForwardCompatibility/TestCase.php',
|
||||
'PHPUnit\\Framework\\TestListener' => $vendorDir . '/phpunit/phpunit/src/ForwardCompatibility/TestListener.php',
|
||||
'PHPUnit\\Framework\\TestSuite' => $vendorDir . '/phpunit/phpunit/src/ForwardCompatibility/TestSuite.php',
|
||||
'PHPUnit_Exception' => $vendorDir . '/phpunit/phpunit/src/Exception.php',
|
||||
'PHPUnit_Extensions_GroupTestSuite' => $vendorDir . '/phpunit/phpunit/src/Extensions/GroupTestSuite.php',
|
||||
'PHPUnit_Extensions_PhptTestCase' => $vendorDir . '/phpunit/phpunit/src/Extensions/PhptTestCase.php',
|
||||
'PHPUnit_Extensions_PhptTestSuite' => $vendorDir . '/phpunit/phpunit/src/Extensions/PhptTestSuite.php',
|
||||
'PHPUnit_Extensions_RepeatedTest' => $vendorDir . '/phpunit/phpunit/src/Extensions/RepeatedTest.php',
|
||||
'PHPUnit_Extensions_TestDecorator' => $vendorDir . '/phpunit/phpunit/src/Extensions/TestDecorator.php',
|
||||
'PHPUnit_Extensions_TicketListener' => $vendorDir . '/phpunit/phpunit/src/Extensions/TicketListener.php',
|
||||
'PHPUnit_Framework_Assert' => $vendorDir . '/phpunit/phpunit/src/Framework/Assert.php',
|
||||
'PHPUnit_Framework_AssertionFailedError' => $vendorDir . '/phpunit/phpunit/src/Framework/AssertionFailedError.php',
|
||||
'PHPUnit_Framework_BaseTestListener' => $vendorDir . '/phpunit/phpunit/src/Framework/BaseTestListener.php',
|
||||
'PHPUnit_Framework_CodeCoverageException' => $vendorDir . '/phpunit/phpunit/src/Framework/CodeCoverageException.php',
|
||||
'PHPUnit_Framework_Constraint' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint.php',
|
||||
'PHPUnit_Framework_Constraint_And' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/And.php',
|
||||
'PHPUnit_Framework_Constraint_ArrayHasKey' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/ArrayHasKey.php',
|
||||
'PHPUnit_Framework_Constraint_ArraySubset' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/ArraySubset.php',
|
||||
'PHPUnit_Framework_Constraint_Attribute' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/Attribute.php',
|
||||
'PHPUnit_Framework_Constraint_Callback' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/Callback.php',
|
||||
'PHPUnit_Framework_Constraint_ClassHasAttribute' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/ClassHasAttribute.php',
|
||||
'PHPUnit_Framework_Constraint_ClassHasStaticAttribute' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/ClassHasStaticAttribute.php',
|
||||
'PHPUnit_Framework_Constraint_Composite' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/Composite.php',
|
||||
'PHPUnit_Framework_Constraint_Count' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/Count.php',
|
||||
'PHPUnit_Framework_Constraint_Exception' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/Exception.php',
|
||||
'PHPUnit_Framework_Constraint_ExceptionCode' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/ExceptionCode.php',
|
||||
'PHPUnit_Framework_Constraint_ExceptionMessage' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/ExceptionMessage.php',
|
||||
'PHPUnit_Framework_Constraint_ExceptionMessageRegExp' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/ExceptionMessageRegExp.php',
|
||||
'PHPUnit_Framework_Constraint_FileExists' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/FileExists.php',
|
||||
'PHPUnit_Framework_Constraint_GreaterThan' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/GreaterThan.php',
|
||||
'PHPUnit_Framework_Constraint_IsAnything' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsAnything.php',
|
||||
'PHPUnit_Framework_Constraint_IsEmpty' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsEmpty.php',
|
||||
'PHPUnit_Framework_Constraint_IsEqual' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsEqual.php',
|
||||
'PHPUnit_Framework_Constraint_IsFalse' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsFalse.php',
|
||||
'PHPUnit_Framework_Constraint_IsIdentical' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsIdentical.php',
|
||||
'PHPUnit_Framework_Constraint_IsInstanceOf' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsInstanceOf.php',
|
||||
'PHPUnit_Framework_Constraint_IsJson' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsJson.php',
|
||||
'PHPUnit_Framework_Constraint_IsNull' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsNull.php',
|
||||
'PHPUnit_Framework_Constraint_IsTrue' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsTrue.php',
|
||||
'PHPUnit_Framework_Constraint_IsType' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/IsType.php',
|
||||
'PHPUnit_Framework_Constraint_JsonMatches' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/JsonMatches.php',
|
||||
'PHPUnit_Framework_Constraint_JsonMatches_ErrorMessageProvider' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/JsonMatches/ErrorMessageProvider.php',
|
||||
'PHPUnit_Framework_Constraint_LessThan' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/LessThan.php',
|
||||
'PHPUnit_Framework_Constraint_Not' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/Not.php',
|
||||
'PHPUnit_Framework_Constraint_ObjectHasAttribute' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/ObjectHasAttribute.php',
|
||||
'PHPUnit_Framework_Constraint_Or' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/Or.php',
|
||||
'PHPUnit_Framework_Constraint_PCREMatch' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/PCREMatch.php',
|
||||
'PHPUnit_Framework_Constraint_SameSize' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/SameSize.php',
|
||||
'PHPUnit_Framework_Constraint_StringContains' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/StringContains.php',
|
||||
'PHPUnit_Framework_Constraint_StringEndsWith' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/StringEndsWith.php',
|
||||
'PHPUnit_Framework_Constraint_StringMatches' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/StringMatches.php',
|
||||
'PHPUnit_Framework_Constraint_StringStartsWith' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/StringStartsWith.php',
|
||||
'PHPUnit_Framework_Constraint_TraversableContains' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/TraversableContains.php',
|
||||
'PHPUnit_Framework_Constraint_TraversableContainsOnly' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/TraversableContainsOnly.php',
|
||||
'PHPUnit_Framework_Constraint_Xor' => $vendorDir . '/phpunit/phpunit/src/Framework/Constraint/Xor.php',
|
||||
'PHPUnit_Framework_Error' => $vendorDir . '/phpunit/phpunit/src/Framework/Error.php',
|
||||
'PHPUnit_Framework_Error_Deprecated' => $vendorDir . '/phpunit/phpunit/src/Framework/Error/Deprecated.php',
|
||||
'PHPUnit_Framework_Error_Notice' => $vendorDir . '/phpunit/phpunit/src/Framework/Error/Notice.php',
|
||||
'PHPUnit_Framework_Error_Warning' => $vendorDir . '/phpunit/phpunit/src/Framework/Error/Warning.php',
|
||||
'PHPUnit_Framework_Exception' => $vendorDir . '/phpunit/phpunit/src/Framework/Exception.php',
|
||||
'PHPUnit_Framework_ExceptionWrapper' => $vendorDir . '/phpunit/phpunit/src/Framework/ExceptionWrapper.php',
|
||||
'PHPUnit_Framework_ExpectationFailedException' => $vendorDir . '/phpunit/phpunit/src/Framework/ExpectationFailedException.php',
|
||||
'PHPUnit_Framework_IncompleteTest' => $vendorDir . '/phpunit/phpunit/src/Framework/IncompleteTest.php',
|
||||
'PHPUnit_Framework_IncompleteTestCase' => $vendorDir . '/phpunit/phpunit/src/Framework/IncompleteTestCase.php',
|
||||
'PHPUnit_Framework_IncompleteTestError' => $vendorDir . '/phpunit/phpunit/src/Framework/IncompleteTestError.php',
|
||||
'PHPUnit_Framework_InvalidCoversTargetError' => $vendorDir . '/phpunit/phpunit/src/Framework/InvalidCoversTargetError.php',
|
||||
'PHPUnit_Framework_InvalidCoversTargetException' => $vendorDir . '/phpunit/phpunit/src/Framework/InvalidCoversTargetException.php',
|
||||
'PHPUnit_Framework_MockObject_BadMethodCallException' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Exception/BadMethodCallException.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_Identity' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/Identity.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_InvocationMocker' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/InvocationMocker.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_Match' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/Match.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_MethodNameMatch' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/MethodNameMatch.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_Namespace' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/Namespace.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_ParametersMatch' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/ParametersMatch.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_Stub' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/Stub.php',
|
||||
'PHPUnit_Framework_MockObject_Exception' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Exception/Exception.php',
|
||||
'PHPUnit_Framework_MockObject_Generator' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Generator.php',
|
||||
'PHPUnit_Framework_MockObject_Invocation' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Invocation.php',
|
||||
'PHPUnit_Framework_MockObject_InvocationMocker' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/InvocationMocker.php',
|
||||
'PHPUnit_Framework_MockObject_Invocation_Object' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Invocation/Object.php',
|
||||
'PHPUnit_Framework_MockObject_Invocation_Static' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Invocation/Static.php',
|
||||
'PHPUnit_Framework_MockObject_Invokable' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Invokable.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_AnyInvokedCount' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/AnyInvokedCount.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_AnyParameters' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/AnyParameters.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_ConsecutiveParameters' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/ConsecutiveParameters.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_Invocation' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/Invocation.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedAtIndex' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedAtIndex.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedAtLeastCount' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedAtLeastCount.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedAtLeastOnce' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedAtLeastOnce.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedAtMostCount' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedAtMostCount.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedCount' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedCount.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedRecorder' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedRecorder.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_MethodName' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/MethodName.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_Parameters' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/Parameters.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_StatelessInvocation' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/StatelessInvocation.php',
|
||||
'PHPUnit_Framework_MockObject_MockBuilder' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/MockBuilder.php',
|
||||
'PHPUnit_Framework_MockObject_MockObject' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/MockObject.php',
|
||||
'PHPUnit_Framework_MockObject_RuntimeException' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Exception/RuntimeException.php',
|
||||
'PHPUnit_Framework_MockObject_Stub' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ConsecutiveCalls' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ConsecutiveCalls.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_Exception' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/Exception.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_MatcherCollection' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/MatcherCollection.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_Return' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/Return.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ReturnArgument' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ReturnArgument.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ReturnCallback' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ReturnCallback.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ReturnSelf' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ReturnSelf.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ReturnValueMap' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ReturnValueMap.php',
|
||||
'PHPUnit_Framework_MockObject_Verifiable' => $vendorDir . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Verifiable.php',
|
||||
'PHPUnit_Framework_OutputError' => $vendorDir . '/phpunit/phpunit/src/Framework/OutputError.php',
|
||||
'PHPUnit_Framework_RiskyTest' => $vendorDir . '/phpunit/phpunit/src/Framework/RiskyTest.php',
|
||||
'PHPUnit_Framework_RiskyTestError' => $vendorDir . '/phpunit/phpunit/src/Framework/RiskyTestError.php',
|
||||
'PHPUnit_Framework_SelfDescribing' => $vendorDir . '/phpunit/phpunit/src/Framework/SelfDescribing.php',
|
||||
'PHPUnit_Framework_SkippedTest' => $vendorDir . '/phpunit/phpunit/src/Framework/SkippedTest.php',
|
||||
'PHPUnit_Framework_SkippedTestCase' => $vendorDir . '/phpunit/phpunit/src/Framework/SkippedTestCase.php',
|
||||
'PHPUnit_Framework_SkippedTestError' => $vendorDir . '/phpunit/phpunit/src/Framework/SkippedTestError.php',
|
||||
'PHPUnit_Framework_SkippedTestSuiteError' => $vendorDir . '/phpunit/phpunit/src/Framework/SkippedTestSuiteError.php',
|
||||
'PHPUnit_Framework_SyntheticError' => $vendorDir . '/phpunit/phpunit/src/Framework/SyntheticError.php',
|
||||
'PHPUnit_Framework_Test' => $vendorDir . '/phpunit/phpunit/src/Framework/Test.php',
|
||||
'PHPUnit_Framework_TestCase' => $vendorDir . '/phpunit/phpunit/src/Framework/TestCase.php',
|
||||
'PHPUnit_Framework_TestFailure' => $vendorDir . '/phpunit/phpunit/src/Framework/TestFailure.php',
|
||||
'PHPUnit_Framework_TestListener' => $vendorDir . '/phpunit/phpunit/src/Framework/TestListener.php',
|
||||
'PHPUnit_Framework_TestResult' => $vendorDir . '/phpunit/phpunit/src/Framework/TestResult.php',
|
||||
'PHPUnit_Framework_TestSuite' => $vendorDir . '/phpunit/phpunit/src/Framework/TestSuite.php',
|
||||
'PHPUnit_Framework_TestSuite_DataProvider' => $vendorDir . '/phpunit/phpunit/src/Framework/TestSuite/DataProvider.php',
|
||||
'PHPUnit_Framework_UnintentionallyCoveredCodeError' => $vendorDir . '/phpunit/phpunit/src/Framework/UnintentionallyCoveredCodeError.php',
|
||||
'PHPUnit_Framework_Warning' => $vendorDir . '/phpunit/phpunit/src/Framework/Warning.php',
|
||||
'PHPUnit_Runner_BaseTestRunner' => $vendorDir . '/phpunit/phpunit/src/Runner/BaseTestRunner.php',
|
||||
'PHPUnit_Runner_Exception' => $vendorDir . '/phpunit/phpunit/src/Runner/Exception.php',
|
||||
'PHPUnit_Runner_Filter_Factory' => $vendorDir . '/phpunit/phpunit/src/Runner/Filter/Factory.php',
|
||||
'PHPUnit_Runner_Filter_GroupFilterIterator' => $vendorDir . '/phpunit/phpunit/src/Runner/Filter/Group.php',
|
||||
'PHPUnit_Runner_Filter_Group_Exclude' => $vendorDir . '/phpunit/phpunit/src/Runner/Filter/Group/Exclude.php',
|
||||
'PHPUnit_Runner_Filter_Group_Include' => $vendorDir . '/phpunit/phpunit/src/Runner/Filter/Group/Include.php',
|
||||
'PHPUnit_Runner_Filter_Test' => $vendorDir . '/phpunit/phpunit/src/Runner/Filter/Test.php',
|
||||
'PHPUnit_Runner_StandardTestSuiteLoader' => $vendorDir . '/phpunit/phpunit/src/Runner/StandardTestSuiteLoader.php',
|
||||
'PHPUnit_Runner_TestSuiteLoader' => $vendorDir . '/phpunit/phpunit/src/Runner/TestSuiteLoader.php',
|
||||
'PHPUnit_Runner_Version' => $vendorDir . '/phpunit/phpunit/src/Runner/Version.php',
|
||||
'PHPUnit_TextUI_Command' => $vendorDir . '/phpunit/phpunit/src/TextUI/Command.php',
|
||||
'PHPUnit_TextUI_ResultPrinter' => $vendorDir . '/phpunit/phpunit/src/TextUI/ResultPrinter.php',
|
||||
'PHPUnit_TextUI_TestRunner' => $vendorDir . '/phpunit/phpunit/src/TextUI/TestRunner.php',
|
||||
'PHPUnit_Util_Blacklist' => $vendorDir . '/phpunit/phpunit/src/Util/Blacklist.php',
|
||||
'PHPUnit_Util_Configuration' => $vendorDir . '/phpunit/phpunit/src/Util/Configuration.php',
|
||||
'PHPUnit_Util_ErrorHandler' => $vendorDir . '/phpunit/phpunit/src/Util/ErrorHandler.php',
|
||||
'PHPUnit_Util_Fileloader' => $vendorDir . '/phpunit/phpunit/src/Util/Fileloader.php',
|
||||
'PHPUnit_Util_Filesystem' => $vendorDir . '/phpunit/phpunit/src/Util/Filesystem.php',
|
||||
'PHPUnit_Util_Filter' => $vendorDir . '/phpunit/phpunit/src/Util/Filter.php',
|
||||
'PHPUnit_Util_Getopt' => $vendorDir . '/phpunit/phpunit/src/Util/Getopt.php',
|
||||
'PHPUnit_Util_GlobalState' => $vendorDir . '/phpunit/phpunit/src/Util/GlobalState.php',
|
||||
'PHPUnit_Util_InvalidArgumentHelper' => $vendorDir . '/phpunit/phpunit/src/Util/InvalidArgumentHelper.php',
|
||||
'PHPUnit_Util_Log_JSON' => $vendorDir . '/phpunit/phpunit/src/Util/Log/JSON.php',
|
||||
'PHPUnit_Util_Log_JUnit' => $vendorDir . '/phpunit/phpunit/src/Util/Log/JUnit.php',
|
||||
'PHPUnit_Util_Log_TAP' => $vendorDir . '/phpunit/phpunit/src/Util/Log/TAP.php',
|
||||
'PHPUnit_Util_PHP' => $vendorDir . '/phpunit/phpunit/src/Util/PHP.php',
|
||||
'PHPUnit_Util_PHP_Default' => $vendorDir . '/phpunit/phpunit/src/Util/PHP/Default.php',
|
||||
'PHPUnit_Util_PHP_Windows' => $vendorDir . '/phpunit/phpunit/src/Util/PHP/Windows.php',
|
||||
'PHPUnit_Util_Printer' => $vendorDir . '/phpunit/phpunit/src/Util/Printer.php',
|
||||
'PHPUnit_Util_Regex' => $vendorDir . '/phpunit/phpunit/src/Util/Regex.php',
|
||||
'PHPUnit_Util_String' => $vendorDir . '/phpunit/phpunit/src/Util/String.php',
|
||||
'PHPUnit_Util_Test' => $vendorDir . '/phpunit/phpunit/src/Util/Test.php',
|
||||
'PHPUnit_Util_TestDox_NamePrettifier' => $vendorDir . '/phpunit/phpunit/src/Util/TestDox/NamePrettifier.php',
|
||||
'PHPUnit_Util_TestDox_ResultPrinter' => $vendorDir . '/phpunit/phpunit/src/Util/TestDox/ResultPrinter.php',
|
||||
'PHPUnit_Util_TestDox_ResultPrinter_HTML' => $vendorDir . '/phpunit/phpunit/src/Util/TestDox/ResultPrinter/HTML.php',
|
||||
'PHPUnit_Util_TestDox_ResultPrinter_Text' => $vendorDir . '/phpunit/phpunit/src/Util/TestDox/ResultPrinter/Text.php',
|
||||
'PHPUnit_Util_TestSuiteIterator' => $vendorDir . '/phpunit/phpunit/src/Util/TestSuiteIterator.php',
|
||||
'PHPUnit_Util_Type' => $vendorDir . '/phpunit/phpunit/src/Util/Type.php',
|
||||
'PHPUnit_Util_XML' => $vendorDir . '/phpunit/phpunit/src/Util/XML.php',
|
||||
'PHP_CodeCoverage' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage.php',
|
||||
'PHP_CodeCoverage_Driver' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Driver.php',
|
||||
'PHP_CodeCoverage_Driver_HHVM' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Driver/HHVM.php',
|
||||
'PHP_CodeCoverage_Driver_PHPDBG' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Driver/PHPDBG.php',
|
||||
'PHP_CodeCoverage_Driver_Xdebug' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Driver/Xdebug.php',
|
||||
'PHP_CodeCoverage_Exception' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Exception.php',
|
||||
'PHP_CodeCoverage_Exception_UnintentionallyCoveredCode' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Exception/UnintentionallyCoveredCode.php',
|
||||
'PHP_CodeCoverage_Filter' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Filter.php',
|
||||
'PHP_CodeCoverage_Report_Clover' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Clover.php',
|
||||
'PHP_CodeCoverage_Report_Crap4j' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Crap4j.php',
|
||||
'PHP_CodeCoverage_Report_Factory' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Factory.php',
|
||||
'PHP_CodeCoverage_Report_HTML' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML.php',
|
||||
'PHP_CodeCoverage_Report_HTML_Renderer' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML/Renderer.php',
|
||||
'PHP_CodeCoverage_Report_HTML_Renderer_Dashboard' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML/Renderer/Dashboard.php',
|
||||
'PHP_CodeCoverage_Report_HTML_Renderer_Directory' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML/Renderer/Directory.php',
|
||||
'PHP_CodeCoverage_Report_HTML_Renderer_File' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML/Renderer/File.php',
|
||||
'PHP_CodeCoverage_Report_Node' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Node.php',
|
||||
'PHP_CodeCoverage_Report_Node_Directory' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Node/Directory.php',
|
||||
'PHP_CodeCoverage_Report_Node_File' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Node/File.php',
|
||||
'PHP_CodeCoverage_Report_Node_Iterator' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Node/Iterator.php',
|
||||
'PHP_CodeCoverage_Report_PHP' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/PHP.php',
|
||||
'PHP_CodeCoverage_Report_Text' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Text.php',
|
||||
'PHP_CodeCoverage_Report_XML' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML.php',
|
||||
'PHP_CodeCoverage_Report_XML_Directory' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Directory.php',
|
||||
'PHP_CodeCoverage_Report_XML_File' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File.php',
|
||||
'PHP_CodeCoverage_Report_XML_File_Coverage' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File/Coverage.php',
|
||||
'PHP_CodeCoverage_Report_XML_File_Method' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File/Method.php',
|
||||
'PHP_CodeCoverage_Report_XML_File_Report' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File/Report.php',
|
||||
'PHP_CodeCoverage_Report_XML_File_Unit' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File/Unit.php',
|
||||
'PHP_CodeCoverage_Report_XML_Node' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Node.php',
|
||||
'PHP_CodeCoverage_Report_XML_Project' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Project.php',
|
||||
'PHP_CodeCoverage_Report_XML_Tests' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Tests.php',
|
||||
'PHP_CodeCoverage_Report_XML_Totals' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Totals.php',
|
||||
'PHP_CodeCoverage_Util' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Util.php',
|
||||
'PHP_CodeCoverage_Util_InvalidArgumentHelper' => $vendorDir . '/phpunit/php-code-coverage/src/CodeCoverage/Util/InvalidArgumentHelper.php',
|
||||
'PHP_Timer' => $vendorDir . '/phpunit/php-timer/src/Timer.php',
|
||||
'PHP_Token' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_TokenWithScope' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_TokenWithScopeAndVisibility' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ABSTRACT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AMPERSAND' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AND_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ARRAY' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ARRAY_CAST' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AS' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ASYNC' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AWAIT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BACKTICK' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BAD_CHARACTER' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BOOLEAN_AND' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BOOLEAN_OR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BOOL_CAST' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BREAK' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CALLABLE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CARET' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CASE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CATCH' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CHARACTER' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLASS' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLASS_C' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLASS_NAME_CONSTANT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLONE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLOSE_BRACKET' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLOSE_CURLY' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLOSE_SQUARE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLOSE_TAG' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COALESCE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COLON' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COMMA' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COMMENT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COMPILER_HALT_OFFSET' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CONCAT_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CONST' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CONSTANT_ENCAPSED_STRING' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CONTINUE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CURLY_OPEN' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DEC' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DECLARE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DEFAULT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DIR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DIV' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DIV_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DNUMBER' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DO' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOC_COMMENT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOLLAR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOLLAR_OPEN_CURLY_BRACES' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOUBLE_ARROW' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOUBLE_CAST' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOUBLE_COLON' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOUBLE_QUOTES' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ECHO' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ELLIPSIS' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ELSE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ELSEIF' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EMPTY' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENCAPSED_AND_WHITESPACE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDDECLARE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDFOR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDFOREACH' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDIF' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDSWITCH' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDWHILE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_END_HEREDOC' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENUM' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EQUALS' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EVAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EXCLAMATION_MARK' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EXIT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EXTENDS' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FILE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FINAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FINALLY' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FOR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FOREACH' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FUNCTION' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FUNC_C' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_GLOBAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_GOTO' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_GT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_HALT_COMPILER' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IF' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IMPLEMENTS' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IN' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INC' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INCLUDE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INCLUDE_ONCE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INLINE_HTML' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INSTANCEOF' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INSTEADOF' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INTERFACE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INT_CAST' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ISSET' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_GREATER_OR_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_IDENTICAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_NOT_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_NOT_IDENTICAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_SMALLER_OR_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_Includes' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_JOIN' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LAMBDA_ARROW' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LAMBDA_CP' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LAMBDA_OP' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LINE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LIST' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LNUMBER' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LOGICAL_AND' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LOGICAL_OR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LOGICAL_XOR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_METHOD_C' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MINUS' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MINUS_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MOD_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MULT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MUL_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NAMESPACE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NEW' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NS_C' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NS_SEPARATOR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NULLSAFE_OBJECT_OPERATOR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NUM_STRING' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OBJECT_CAST' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OBJECT_OPERATOR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ONUMBER' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_BRACKET' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_CURLY' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_SQUARE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_TAG' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_TAG_WITH_ECHO' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OR_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PAAMAYIM_NEKUDOTAYIM' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PERCENT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PIPE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PLUS' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PLUS_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_POW' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_POW_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PRINT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PRIVATE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PROTECTED' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PUBLIC' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_QUESTION_MARK' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_REQUIRE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_REQUIRE_ONCE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_RETURN' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SEMICOLON' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SHAPE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SL_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SPACESHIP' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SR_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_START_HEREDOC' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_STATIC' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_STRING' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_STRING_CAST' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_STRING_VARNAME' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SUPER' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SWITCH' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_Stream' => $vendorDir . '/phpunit/php-token-stream/src/Token/Stream.php',
|
||||
'PHP_Token_Stream_CachingFactory' => $vendorDir . '/phpunit/php-token-stream/src/Token/Stream/CachingFactory.php',
|
||||
'PHP_Token_THROW' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TILDE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TRAIT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TRAIT_C' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TRY' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TYPE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TYPELIST_GT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TYPELIST_LT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_UNSET' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_UNSET_CAST' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_USE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_USE_FUNCTION' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_VAR' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_VARIABLE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_WHERE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_WHILE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_WHITESPACE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_ATTRIBUTE' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_CATEGORY' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_CATEGORY_LABEL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_CHILDREN' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_LABEL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_REQUIRED' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_TAG_GT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_TAG_LT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_TEXT' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XOR_EQUAL' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_YIELD' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_YIELD_FROM' => $vendorDir . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PclZip' => $vendorDir . '/pclzip/pclzip/pclzip.lib.php',
|
||||
'SebastianBergmann\\Comparator\\ArrayComparator' => $vendorDir . '/sebastian/comparator/src/ArrayComparator.php',
|
||||
'SebastianBergmann\\Comparator\\Comparator' => $vendorDir . '/sebastian/comparator/src/Comparator.php',
|
||||
'SebastianBergmann\\Comparator\\ComparisonFailure' => $vendorDir . '/sebastian/comparator/src/ComparisonFailure.php',
|
||||
'SebastianBergmann\\Comparator\\DOMNodeComparator' => $vendorDir . '/sebastian/comparator/src/DOMNodeComparator.php',
|
||||
'SebastianBergmann\\Comparator\\DateTimeComparator' => $vendorDir . '/sebastian/comparator/src/DateTimeComparator.php',
|
||||
'SebastianBergmann\\Comparator\\DoubleComparator' => $vendorDir . '/sebastian/comparator/src/DoubleComparator.php',
|
||||
'SebastianBergmann\\Comparator\\ExceptionComparator' => $vendorDir . '/sebastian/comparator/src/ExceptionComparator.php',
|
||||
'SebastianBergmann\\Comparator\\Factory' => $vendorDir . '/sebastian/comparator/src/Factory.php',
|
||||
'SebastianBergmann\\Comparator\\MockObjectComparator' => $vendorDir . '/sebastian/comparator/src/MockObjectComparator.php',
|
||||
'SebastianBergmann\\Comparator\\NumericComparator' => $vendorDir . '/sebastian/comparator/src/NumericComparator.php',
|
||||
'SebastianBergmann\\Comparator\\ObjectComparator' => $vendorDir . '/sebastian/comparator/src/ObjectComparator.php',
|
||||
'SebastianBergmann\\Comparator\\ResourceComparator' => $vendorDir . '/sebastian/comparator/src/ResourceComparator.php',
|
||||
'SebastianBergmann\\Comparator\\ScalarComparator' => $vendorDir . '/sebastian/comparator/src/ScalarComparator.php',
|
||||
'SebastianBergmann\\Comparator\\SplObjectStorageComparator' => $vendorDir . '/sebastian/comparator/src/SplObjectStorageComparator.php',
|
||||
'SebastianBergmann\\Comparator\\TypeComparator' => $vendorDir . '/sebastian/comparator/src/TypeComparator.php',
|
||||
'SebastianBergmann\\Diff\\Chunk' => $vendorDir . '/sebastian/diff/src/Chunk.php',
|
||||
'SebastianBergmann\\Diff\\Diff' => $vendorDir . '/sebastian/diff/src/Diff.php',
|
||||
'SebastianBergmann\\Diff\\Differ' => $vendorDir . '/sebastian/diff/src/Differ.php',
|
||||
'SebastianBergmann\\Diff\\LCS\\LongestCommonSubsequence' => $vendorDir . '/sebastian/diff/src/LCS/LongestCommonSubsequence.php',
|
||||
'SebastianBergmann\\Diff\\LCS\\MemoryEfficientImplementation' => $vendorDir . '/sebastian/diff/src/LCS/MemoryEfficientLongestCommonSubsequenceImplementation.php',
|
||||
'SebastianBergmann\\Diff\\LCS\\TimeEfficientImplementation' => $vendorDir . '/sebastian/diff/src/LCS/TimeEfficientLongestCommonSubsequenceImplementation.php',
|
||||
'SebastianBergmann\\Diff\\Line' => $vendorDir . '/sebastian/diff/src/Line.php',
|
||||
'SebastianBergmann\\Diff\\Parser' => $vendorDir . '/sebastian/diff/src/Parser.php',
|
||||
'SebastianBergmann\\Environment\\Console' => $vendorDir . '/sebastian/environment/src/Console.php',
|
||||
'SebastianBergmann\\Environment\\Runtime' => $vendorDir . '/sebastian/environment/src/Runtime.php',
|
||||
'SebastianBergmann\\Exporter\\Exporter' => $vendorDir . '/sebastian/exporter/src/Exporter.php',
|
||||
'SebastianBergmann\\GlobalState\\Blacklist' => $vendorDir . '/sebastian/global-state/src/Blacklist.php',
|
||||
'SebastianBergmann\\GlobalState\\CodeExporter' => $vendorDir . '/sebastian/global-state/src/CodeExporter.php',
|
||||
'SebastianBergmann\\GlobalState\\Exception' => $vendorDir . '/sebastian/global-state/src/Exception.php',
|
||||
'SebastianBergmann\\GlobalState\\Restorer' => $vendorDir . '/sebastian/global-state/src/Restorer.php',
|
||||
'SebastianBergmann\\GlobalState\\RuntimeException' => $vendorDir . '/sebastian/global-state/src/RuntimeException.php',
|
||||
'SebastianBergmann\\GlobalState\\Snapshot' => $vendorDir . '/sebastian/global-state/src/Snapshot.php',
|
||||
'SebastianBergmann\\RecursionContext\\Context' => $vendorDir . '/sebastian/recursion-context/src/Context.php',
|
||||
'SebastianBergmann\\RecursionContext\\Exception' => $vendorDir . '/sebastian/recursion-context/src/Exception.php',
|
||||
'SebastianBergmann\\RecursionContext\\InvalidArgumentException' => $vendorDir . '/sebastian/recursion-context/src/InvalidArgumentException.php',
|
||||
'SebastianBergmann\\Version' => $vendorDir . '/sebastian/version/src/Version.php',
|
||||
'Text_Template' => $vendorDir . '/phpunit/php-text-template/src/Template.php',
|
||||
);
|
@ -0,0 +1,73 @@
|
||||
<?php
|
||||
|
||||
// autoload_files.php @generated by Composer
|
||||
|
||||
$vendorDir = dirname(dirname(__FILE__));
|
||||
$baseDir = dirname($vendorDir);
|
||||
|
||||
return array(
|
||||
'320cde22f66dd4f5d3fd621d3e88b98f' => $vendorDir . '/symfony/polyfill-ctype/bootstrap.php',
|
||||
'0e6d7bf4a5811bfa5cf40c5ccd6fae6a' => $vendorDir . '/symfony/polyfill-mbstring/bootstrap.php',
|
||||
'9b552a3cc426e3287cc811caefa3cf53' => $vendorDir . '/topthink/think-helper/src/helper.php',
|
||||
'abede361264e2ae69ec1eee813a101af' => $vendorDir . '/markbaker/complex/classes/src/functions/abs.php',
|
||||
'21a5860fbef5be28db5ddfbc3cca67c4' => $vendorDir . '/markbaker/complex/classes/src/functions/acos.php',
|
||||
'1546e3f9d127f2a9bb2d1b6c31c26ef1' => $vendorDir . '/markbaker/complex/classes/src/functions/acosh.php',
|
||||
'd2516f7f4fba5ea5905f494b4a8262e0' => $vendorDir . '/markbaker/complex/classes/src/functions/acot.php',
|
||||
'4511163d560956219b96882c0980b65e' => $vendorDir . '/markbaker/complex/classes/src/functions/acoth.php',
|
||||
'c361f5616dc2a8da4fa3e137077cd4ea' => $vendorDir . '/markbaker/complex/classes/src/functions/acsc.php',
|
||||
'02d68920fc98da71991ce569c91df0f6' => $vendorDir . '/markbaker/complex/classes/src/functions/acsch.php',
|
||||
'88e19525eae308b4a6aa3419364875d3' => $vendorDir . '/markbaker/complex/classes/src/functions/argument.php',
|
||||
'60e8e2d0827b58bfc904f13957e51849' => $vendorDir . '/markbaker/complex/classes/src/functions/asec.php',
|
||||
'13d2f040713999eab66c359b4d79871d' => $vendorDir . '/markbaker/complex/classes/src/functions/asech.php',
|
||||
'838ab38beb32c68a79d3cd2c007d5a04' => $vendorDir . '/markbaker/complex/classes/src/functions/asin.php',
|
||||
'bb28eccd0f8f008333a1b3c163d604ac' => $vendorDir . '/markbaker/complex/classes/src/functions/asinh.php',
|
||||
'9e483de83558c98f7d3feaa402c78cb3' => $vendorDir . '/markbaker/complex/classes/src/functions/atan.php',
|
||||
'36b74b5b765ded91ee58c8ee3c0e85e3' => $vendorDir . '/markbaker/complex/classes/src/functions/atanh.php',
|
||||
'05c15ee9510da7fd6bf6136f436500c0' => $vendorDir . '/markbaker/complex/classes/src/functions/conjugate.php',
|
||||
'd3208dfbce2505e370788f9f22f6785f' => $vendorDir . '/markbaker/complex/classes/src/functions/cos.php',
|
||||
'141cf1fb3a3046f8b64534b0ebab33ca' => $vendorDir . '/markbaker/complex/classes/src/functions/cosh.php',
|
||||
'be660df75fd0dbe7fa7c03b7434b3294' => $vendorDir . '/markbaker/complex/classes/src/functions/cot.php',
|
||||
'01e31ea298a51bc9e91517e3ce6b9e76' => $vendorDir . '/markbaker/complex/classes/src/functions/coth.php',
|
||||
'803ddd97f7b1da68982a7b087c3476f6' => $vendorDir . '/markbaker/complex/classes/src/functions/csc.php',
|
||||
'3001cdfd101ec3c32da34ee43c2e149b' => $vendorDir . '/markbaker/complex/classes/src/functions/csch.php',
|
||||
'77b2d7629ef2a93fabb8c56754a91051' => $vendorDir . '/markbaker/complex/classes/src/functions/exp.php',
|
||||
'4a4471296dec796c21d4f4b6552396a9' => $vendorDir . '/markbaker/complex/classes/src/functions/inverse.php',
|
||||
'c3e9897e1744b88deb56fcdc39d34d85' => $vendorDir . '/markbaker/complex/classes/src/functions/ln.php',
|
||||
'a83cacf2de942cff288de15a83afd26d' => $vendorDir . '/markbaker/complex/classes/src/functions/log2.php',
|
||||
'6a861dacc9ee2f3061241d4c7772fa21' => $vendorDir . '/markbaker/complex/classes/src/functions/log10.php',
|
||||
'4d2522d968c8ba78d6c13548a1b4200e' => $vendorDir . '/markbaker/complex/classes/src/functions/negative.php',
|
||||
'fd587ca933fc0447fa5ab4843bdd97f7' => $vendorDir . '/markbaker/complex/classes/src/functions/pow.php',
|
||||
'383ef01c62028fc78cd4388082fce3c2' => $vendorDir . '/markbaker/complex/classes/src/functions/rho.php',
|
||||
'150fbd1b95029dc47292da97ecab9375' => $vendorDir . '/markbaker/complex/classes/src/functions/sec.php',
|
||||
'549abd9bae174286d660bdaa07407c68' => $vendorDir . '/markbaker/complex/classes/src/functions/sech.php',
|
||||
'6bfbf5eaea6b17a0ed85cb21ba80370c' => $vendorDir . '/markbaker/complex/classes/src/functions/sin.php',
|
||||
'22efe13f1a497b8e199540ae2d9dc59c' => $vendorDir . '/markbaker/complex/classes/src/functions/sinh.php',
|
||||
'e90135ab8e787795a509ed7147de207d' => $vendorDir . '/markbaker/complex/classes/src/functions/sqrt.php',
|
||||
'bb0a7923ffc6a90919cd64ec54ff06bc' => $vendorDir . '/markbaker/complex/classes/src/functions/tan.php',
|
||||
'2d302f32ce0fd4e433dd91c5bb404a28' => $vendorDir . '/markbaker/complex/classes/src/functions/tanh.php',
|
||||
'24dd4658a952171a4ee79218c4f9fd06' => $vendorDir . '/markbaker/complex/classes/src/functions/theta.php',
|
||||
'e49b7876281d6f5bc39536dde96d1f4a' => $vendorDir . '/markbaker/complex/classes/src/operations/add.php',
|
||||
'47596e02b43cd6da7700134fd08f88cf' => $vendorDir . '/markbaker/complex/classes/src/operations/subtract.php',
|
||||
'883af48563631547925fa4c3b48ead07' => $vendorDir . '/markbaker/complex/classes/src/operations/multiply.php',
|
||||
'f190e3308e6ca23234a2875edc985c03' => $vendorDir . '/markbaker/complex/classes/src/operations/divideby.php',
|
||||
'ac9e33ce6841aa5bf5d16d465a2f03a7' => $vendorDir . '/markbaker/complex/classes/src/operations/divideinto.php',
|
||||
'3af723442581d6c310bf44543f9f5c60' => $vendorDir . '/markbaker/matrix/classes/src/Functions/adjoint.php',
|
||||
'd803221834c8b57fec95debb5406a797' => $vendorDir . '/markbaker/matrix/classes/src/Functions/antidiagonal.php',
|
||||
'4714cafbd3be4c72c274a25eae9396bb' => $vendorDir . '/markbaker/matrix/classes/src/Functions/cofactors.php',
|
||||
'89719dc7c77436609d1c1c31f0797b8f' => $vendorDir . '/markbaker/matrix/classes/src/Functions/determinant.php',
|
||||
'c28af79ec7730859d83f2d4310b8dd0b' => $vendorDir . '/markbaker/matrix/classes/src/Functions/diagonal.php',
|
||||
'c5d82bf1ac485e445f911e55789ab4e6' => $vendorDir . '/markbaker/matrix/classes/src/Functions/identity.php',
|
||||
'0d2d594de24a247f7a33499e933aa21e' => $vendorDir . '/markbaker/matrix/classes/src/Functions/inverse.php',
|
||||
'f37c25880804a014ef40c8bffbab1b10' => $vendorDir . '/markbaker/matrix/classes/src/Functions/minors.php',
|
||||
'd6e4e42171df0dbea253b3067fefda38' => $vendorDir . '/markbaker/matrix/classes/src/Functions/trace.php',
|
||||
'2c9b19fa954fd3e6fcc7e7a1383caddd' => $vendorDir . '/markbaker/matrix/classes/src/Functions/transpose.php',
|
||||
'0a538fc9b897450ec362480ebbebe94f' => $vendorDir . '/markbaker/matrix/classes/src/Operations/add.php',
|
||||
'f0843f7f4089ec2343c7445544356385' => $vendorDir . '/markbaker/matrix/classes/src/Operations/directsum.php',
|
||||
'ad3e8c29aa16d134661a414265677b61' => $vendorDir . '/markbaker/matrix/classes/src/Operations/subtract.php',
|
||||
'8d37dad4703fab45bfec9dd0bbf3278e' => $vendorDir . '/markbaker/matrix/classes/src/Operations/multiply.php',
|
||||
'4888a6f58c08148ebe17682f9ce9b2a8' => $vendorDir . '/markbaker/matrix/classes/src/Operations/divideby.php',
|
||||
'eef6fa3879d3efa347cd24d5eb348f85' => $vendorDir . '/markbaker/matrix/classes/src/Operations/divideinto.php',
|
||||
'ddc3cd2a04224f9638c5d0de6a69c7e3' => $vendorDir . '/topthink/think-migration/src/config.php',
|
||||
'cc56288302d9df745d97c934d6a6e5f0' => $vendorDir . '/topthink/think-queue/src/common.php',
|
||||
'72c97b53391125cae04082a81029f42d' => $vendorDir . '/topthink/think-testing/src/config.php',
|
||||
);
|
@ -0,0 +1,10 @@
|
||||
<?php
|
||||
|
||||
// autoload_namespaces.php @generated by Composer
|
||||
|
||||
$vendorDir = dirname(dirname(__FILE__));
|
||||
$baseDir = dirname($vendorDir);
|
||||
|
||||
return array(
|
||||
'PHPExcel' => array($vendorDir . '/phpoffice/phpexcel/Classes'),
|
||||
);
|
@ -0,0 +1,34 @@
|
||||
<?php
|
||||
|
||||
// autoload_psr4.php @generated by Composer
|
||||
|
||||
$vendorDir = dirname(dirname(__FILE__));
|
||||
$baseDir = dirname($vendorDir);
|
||||
|
||||
return array(
|
||||
'think\\worker\\' => array($vendorDir . '/topthink/think-worker/src'),
|
||||
'think\\testing\\' => array($vendorDir . '/topthink/think-testing/src'),
|
||||
'think\\sae\\' => array($vendorDir . '/topthink/think-sae/src'),
|
||||
'think\\mongo\\' => array($vendorDir . '/topthink/think-mongo/src'),
|
||||
'think\\migration\\' => array($vendorDir . '/topthink/think-migration/src'),
|
||||
'think\\helper\\' => array($vendorDir . '/topthink/think-helper/src'),
|
||||
'think\\composer\\' => array($vendorDir . '/topthink/think-installer/src'),
|
||||
'think\\' => array($baseDir . '/thinkphp/library/think', $vendorDir . '/topthink/think-image/src', $vendorDir . '/topthink/think-queue/src'),
|
||||
'phpDocumentor\\Reflection\\' => array($vendorDir . '/phpdocumentor/reflection-common/src', $vendorDir . '/phpdocumentor/reflection-docblock/src', $vendorDir . '/phpdocumentor/type-resolver/src'),
|
||||
'Zend\\Escaper\\' => array($vendorDir . '/zendframework/zend-escaper/src'),
|
||||
'Workerman\\' => array($vendorDir . '/workerman/workerman'),
|
||||
'Webmozart\\Assert\\' => array($vendorDir . '/webmozart/assert/src'),
|
||||
'Symfony\\Polyfill\\Mbstring\\' => array($vendorDir . '/symfony/polyfill-mbstring'),
|
||||
'Symfony\\Polyfill\\Ctype\\' => array($vendorDir . '/symfony/polyfill-ctype'),
|
||||
'Symfony\\Component\\Yaml\\' => array($vendorDir . '/symfony/yaml'),
|
||||
'Symfony\\Component\\DomCrawler\\' => array($vendorDir . '/symfony/dom-crawler'),
|
||||
'Psr\\SimpleCache\\' => array($vendorDir . '/psr/simple-cache/src'),
|
||||
'Prophecy\\' => array($vendorDir . '/phpspec/prophecy/src/Prophecy'),
|
||||
'PhpOffice\\PhpWord\\' => array($vendorDir . '/phpoffice/phpword/src/PhpWord'),
|
||||
'PhpOffice\\PhpSpreadsheet\\' => array($vendorDir . '/phpoffice/phpspreadsheet/src/PhpSpreadsheet'),
|
||||
'PhpOffice\\Common\\' => array($vendorDir . '/phpoffice/common/src/Common'),
|
||||
'Phinx\\' => array($vendorDir . '/topthink/think-migration/phinx/src/Phinx'),
|
||||
'Matrix\\' => array($vendorDir . '/markbaker/matrix/classes/src'),
|
||||
'Doctrine\\Instantiator\\' => array($vendorDir . '/doctrine/instantiator/src/Doctrine/Instantiator'),
|
||||
'Complex\\' => array($vendorDir . '/markbaker/complex/classes/src'),
|
||||
);
|
@ -0,0 +1,75 @@
|
||||
<?php
|
||||
|
||||
// autoload_real.php @generated by Composer
|
||||
|
||||
class ComposerAutoloaderInitc184f419bfb1b5c35adb1bc36e45b15e
|
||||
{
|
||||
private static $loader;
|
||||
|
||||
public static function loadClassLoader($class)
|
||||
{
|
||||
if ('Composer\Autoload\ClassLoader' === $class) {
|
||||
require __DIR__ . '/ClassLoader.php';
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* @return \Composer\Autoload\ClassLoader
|
||||
*/
|
||||
public static function getLoader()
|
||||
{
|
||||
if (null !== self::$loader) {
|
||||
return self::$loader;
|
||||
}
|
||||
|
||||
require __DIR__ . '/platform_check.php';
|
||||
|
||||
spl_autoload_register(array('ComposerAutoloaderInitc184f419bfb1b5c35adb1bc36e45b15e', 'loadClassLoader'), true, true);
|
||||
self::$loader = $loader = new \Composer\Autoload\ClassLoader(\dirname(\dirname(__FILE__)));
|
||||
spl_autoload_unregister(array('ComposerAutoloaderInitc184f419bfb1b5c35adb1bc36e45b15e', 'loadClassLoader'));
|
||||
|
||||
$useStaticLoader = PHP_VERSION_ID >= 50600 && !defined('HHVM_VERSION') && (!function_exists('zend_loader_file_encoded') || !zend_loader_file_encoded());
|
||||
if ($useStaticLoader) {
|
||||
require __DIR__ . '/autoload_static.php';
|
||||
|
||||
call_user_func(\Composer\Autoload\ComposerStaticInitc184f419bfb1b5c35adb1bc36e45b15e::getInitializer($loader));
|
||||
} else {
|
||||
$map = require __DIR__ . '/autoload_namespaces.php';
|
||||
foreach ($map as $namespace => $path) {
|
||||
$loader->set($namespace, $path);
|
||||
}
|
||||
|
||||
$map = require __DIR__ . '/autoload_psr4.php';
|
||||
foreach ($map as $namespace => $path) {
|
||||
$loader->setPsr4($namespace, $path);
|
||||
}
|
||||
|
||||
$classMap = require __DIR__ . '/autoload_classmap.php';
|
||||
if ($classMap) {
|
||||
$loader->addClassMap($classMap);
|
||||
}
|
||||
}
|
||||
|
||||
$loader->register(true);
|
||||
|
||||
if ($useStaticLoader) {
|
||||
$includeFiles = Composer\Autoload\ComposerStaticInitc184f419bfb1b5c35adb1bc36e45b15e::$files;
|
||||
} else {
|
||||
$includeFiles = require __DIR__ . '/autoload_files.php';
|
||||
}
|
||||
foreach ($includeFiles as $fileIdentifier => $file) {
|
||||
composerRequirec184f419bfb1b5c35adb1bc36e45b15e($fileIdentifier, $file);
|
||||
}
|
||||
|
||||
return $loader;
|
||||
}
|
||||
}
|
||||
|
||||
function composerRequirec184f419bfb1b5c35adb1bc36e45b15e($fileIdentifier, $file)
|
||||
{
|
||||
if (empty($GLOBALS['__composer_autoload_files'][$fileIdentifier])) {
|
||||
require $file;
|
||||
|
||||
$GLOBALS['__composer_autoload_files'][$fileIdentifier] = true;
|
||||
}
|
||||
}
|
@ -0,0 +1,708 @@
|
||||
<?php
|
||||
|
||||
// autoload_static.php @generated by Composer
|
||||
|
||||
namespace Composer\Autoload;
|
||||
|
||||
class ComposerStaticInitc184f419bfb1b5c35adb1bc36e45b15e
|
||||
{
|
||||
public static $files = array (
|
||||
'320cde22f66dd4f5d3fd621d3e88b98f' => __DIR__ . '/..' . '/symfony/polyfill-ctype/bootstrap.php',
|
||||
'0e6d7bf4a5811bfa5cf40c5ccd6fae6a' => __DIR__ . '/..' . '/symfony/polyfill-mbstring/bootstrap.php',
|
||||
'9b552a3cc426e3287cc811caefa3cf53' => __DIR__ . '/..' . '/topthink/think-helper/src/helper.php',
|
||||
'abede361264e2ae69ec1eee813a101af' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/abs.php',
|
||||
'21a5860fbef5be28db5ddfbc3cca67c4' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/acos.php',
|
||||
'1546e3f9d127f2a9bb2d1b6c31c26ef1' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/acosh.php',
|
||||
'd2516f7f4fba5ea5905f494b4a8262e0' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/acot.php',
|
||||
'4511163d560956219b96882c0980b65e' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/acoth.php',
|
||||
'c361f5616dc2a8da4fa3e137077cd4ea' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/acsc.php',
|
||||
'02d68920fc98da71991ce569c91df0f6' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/acsch.php',
|
||||
'88e19525eae308b4a6aa3419364875d3' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/argument.php',
|
||||
'60e8e2d0827b58bfc904f13957e51849' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/asec.php',
|
||||
'13d2f040713999eab66c359b4d79871d' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/asech.php',
|
||||
'838ab38beb32c68a79d3cd2c007d5a04' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/asin.php',
|
||||
'bb28eccd0f8f008333a1b3c163d604ac' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/asinh.php',
|
||||
'9e483de83558c98f7d3feaa402c78cb3' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/atan.php',
|
||||
'36b74b5b765ded91ee58c8ee3c0e85e3' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/atanh.php',
|
||||
'05c15ee9510da7fd6bf6136f436500c0' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/conjugate.php',
|
||||
'd3208dfbce2505e370788f9f22f6785f' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/cos.php',
|
||||
'141cf1fb3a3046f8b64534b0ebab33ca' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/cosh.php',
|
||||
'be660df75fd0dbe7fa7c03b7434b3294' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/cot.php',
|
||||
'01e31ea298a51bc9e91517e3ce6b9e76' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/coth.php',
|
||||
'803ddd97f7b1da68982a7b087c3476f6' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/csc.php',
|
||||
'3001cdfd101ec3c32da34ee43c2e149b' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/csch.php',
|
||||
'77b2d7629ef2a93fabb8c56754a91051' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/exp.php',
|
||||
'4a4471296dec796c21d4f4b6552396a9' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/inverse.php',
|
||||
'c3e9897e1744b88deb56fcdc39d34d85' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/ln.php',
|
||||
'a83cacf2de942cff288de15a83afd26d' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/log2.php',
|
||||
'6a861dacc9ee2f3061241d4c7772fa21' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/log10.php',
|
||||
'4d2522d968c8ba78d6c13548a1b4200e' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/negative.php',
|
||||
'fd587ca933fc0447fa5ab4843bdd97f7' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/pow.php',
|
||||
'383ef01c62028fc78cd4388082fce3c2' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/rho.php',
|
||||
'150fbd1b95029dc47292da97ecab9375' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/sec.php',
|
||||
'549abd9bae174286d660bdaa07407c68' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/sech.php',
|
||||
'6bfbf5eaea6b17a0ed85cb21ba80370c' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/sin.php',
|
||||
'22efe13f1a497b8e199540ae2d9dc59c' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/sinh.php',
|
||||
'e90135ab8e787795a509ed7147de207d' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/sqrt.php',
|
||||
'bb0a7923ffc6a90919cd64ec54ff06bc' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/tan.php',
|
||||
'2d302f32ce0fd4e433dd91c5bb404a28' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/tanh.php',
|
||||
'24dd4658a952171a4ee79218c4f9fd06' => __DIR__ . '/..' . '/markbaker/complex/classes/src/functions/theta.php',
|
||||
'e49b7876281d6f5bc39536dde96d1f4a' => __DIR__ . '/..' . '/markbaker/complex/classes/src/operations/add.php',
|
||||
'47596e02b43cd6da7700134fd08f88cf' => __DIR__ . '/..' . '/markbaker/complex/classes/src/operations/subtract.php',
|
||||
'883af48563631547925fa4c3b48ead07' => __DIR__ . '/..' . '/markbaker/complex/classes/src/operations/multiply.php',
|
||||
'f190e3308e6ca23234a2875edc985c03' => __DIR__ . '/..' . '/markbaker/complex/classes/src/operations/divideby.php',
|
||||
'ac9e33ce6841aa5bf5d16d465a2f03a7' => __DIR__ . '/..' . '/markbaker/complex/classes/src/operations/divideinto.php',
|
||||
'3af723442581d6c310bf44543f9f5c60' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/adjoint.php',
|
||||
'd803221834c8b57fec95debb5406a797' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/antidiagonal.php',
|
||||
'4714cafbd3be4c72c274a25eae9396bb' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/cofactors.php',
|
||||
'89719dc7c77436609d1c1c31f0797b8f' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/determinant.php',
|
||||
'c28af79ec7730859d83f2d4310b8dd0b' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/diagonal.php',
|
||||
'c5d82bf1ac485e445f911e55789ab4e6' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/identity.php',
|
||||
'0d2d594de24a247f7a33499e933aa21e' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/inverse.php',
|
||||
'f37c25880804a014ef40c8bffbab1b10' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/minors.php',
|
||||
'd6e4e42171df0dbea253b3067fefda38' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/trace.php',
|
||||
'2c9b19fa954fd3e6fcc7e7a1383caddd' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Functions/transpose.php',
|
||||
'0a538fc9b897450ec362480ebbebe94f' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Operations/add.php',
|
||||
'f0843f7f4089ec2343c7445544356385' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Operations/directsum.php',
|
||||
'ad3e8c29aa16d134661a414265677b61' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Operations/subtract.php',
|
||||
'8d37dad4703fab45bfec9dd0bbf3278e' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Operations/multiply.php',
|
||||
'4888a6f58c08148ebe17682f9ce9b2a8' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Operations/divideby.php',
|
||||
'eef6fa3879d3efa347cd24d5eb348f85' => __DIR__ . '/..' . '/markbaker/matrix/classes/src/Operations/divideinto.php',
|
||||
'ddc3cd2a04224f9638c5d0de6a69c7e3' => __DIR__ . '/..' . '/topthink/think-migration/src/config.php',
|
||||
'cc56288302d9df745d97c934d6a6e5f0' => __DIR__ . '/..' . '/topthink/think-queue/src/common.php',
|
||||
'72c97b53391125cae04082a81029f42d' => __DIR__ . '/..' . '/topthink/think-testing/src/config.php',
|
||||
);
|
||||
|
||||
public static $prefixLengthsPsr4 = array (
|
||||
't' =>
|
||||
array (
|
||||
'think\\worker\\' => 13,
|
||||
'think\\testing\\' => 14,
|
||||
'think\\sae\\' => 10,
|
||||
'think\\mongo\\' => 12,
|
||||
'think\\migration\\' => 16,
|
||||
'think\\helper\\' => 13,
|
||||
'think\\composer\\' => 15,
|
||||
'think\\' => 6,
|
||||
),
|
||||
'p' =>
|
||||
array (
|
||||
'phpDocumentor\\Reflection\\' => 25,
|
||||
),
|
||||
'Z' =>
|
||||
array (
|
||||
'Zend\\Escaper\\' => 13,
|
||||
),
|
||||
'W' =>
|
||||
array (
|
||||
'Workerman\\' => 10,
|
||||
'Webmozart\\Assert\\' => 17,
|
||||
),
|
||||
'S' =>
|
||||
array (
|
||||
'Symfony\\Polyfill\\Mbstring\\' => 26,
|
||||
'Symfony\\Polyfill\\Ctype\\' => 23,
|
||||
'Symfony\\Component\\Yaml\\' => 23,
|
||||
'Symfony\\Component\\DomCrawler\\' => 29,
|
||||
),
|
||||
'P' =>
|
||||
array (
|
||||
'Psr\\SimpleCache\\' => 16,
|
||||
'Prophecy\\' => 9,
|
||||
'PhpOffice\\PhpWord\\' => 18,
|
||||
'PhpOffice\\PhpSpreadsheet\\' => 25,
|
||||
'PhpOffice\\Common\\' => 17,
|
||||
'Phinx\\' => 6,
|
||||
),
|
||||
'M' =>
|
||||
array (
|
||||
'Matrix\\' => 7,
|
||||
),
|
||||
'D' =>
|
||||
array (
|
||||
'Doctrine\\Instantiator\\' => 22,
|
||||
),
|
||||
'C' =>
|
||||
array (
|
||||
'Complex\\' => 8,
|
||||
),
|
||||
);
|
||||
|
||||
public static $prefixDirsPsr4 = array (
|
||||
'think\\worker\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/topthink/think-worker/src',
|
||||
),
|
||||
'think\\testing\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/topthink/think-testing/src',
|
||||
),
|
||||
'think\\sae\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/topthink/think-sae/src',
|
||||
),
|
||||
'think\\mongo\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/topthink/think-mongo/src',
|
||||
),
|
||||
'think\\migration\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/topthink/think-migration/src',
|
||||
),
|
||||
'think\\helper\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/topthink/think-helper/src',
|
||||
),
|
||||
'think\\composer\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/topthink/think-installer/src',
|
||||
),
|
||||
'think\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/../..' . '/thinkphp/library/think',
|
||||
1 => __DIR__ . '/..' . '/topthink/think-image/src',
|
||||
2 => __DIR__ . '/..' . '/topthink/think-queue/src',
|
||||
),
|
||||
'phpDocumentor\\Reflection\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/phpdocumentor/reflection-common/src',
|
||||
1 => __DIR__ . '/..' . '/phpdocumentor/reflection-docblock/src',
|
||||
2 => __DIR__ . '/..' . '/phpdocumentor/type-resolver/src',
|
||||
),
|
||||
'Zend\\Escaper\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/zendframework/zend-escaper/src',
|
||||
),
|
||||
'Workerman\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/workerman/workerman',
|
||||
),
|
||||
'Webmozart\\Assert\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/webmozart/assert/src',
|
||||
),
|
||||
'Symfony\\Polyfill\\Mbstring\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/symfony/polyfill-mbstring',
|
||||
),
|
||||
'Symfony\\Polyfill\\Ctype\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/symfony/polyfill-ctype',
|
||||
),
|
||||
'Symfony\\Component\\Yaml\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/symfony/yaml',
|
||||
),
|
||||
'Symfony\\Component\\DomCrawler\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/symfony/dom-crawler',
|
||||
),
|
||||
'Psr\\SimpleCache\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/psr/simple-cache/src',
|
||||
),
|
||||
'Prophecy\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/phpspec/prophecy/src/Prophecy',
|
||||
),
|
||||
'PhpOffice\\PhpWord\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/phpoffice/phpword/src/PhpWord',
|
||||
),
|
||||
'PhpOffice\\PhpSpreadsheet\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/phpoffice/phpspreadsheet/src/PhpSpreadsheet',
|
||||
),
|
||||
'PhpOffice\\Common\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/phpoffice/common/src/Common',
|
||||
),
|
||||
'Phinx\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/topthink/think-migration/phinx/src/Phinx',
|
||||
),
|
||||
'Matrix\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/markbaker/matrix/classes/src',
|
||||
),
|
||||
'Doctrine\\Instantiator\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/doctrine/instantiator/src/Doctrine/Instantiator',
|
||||
),
|
||||
'Complex\\' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/markbaker/complex/classes/src',
|
||||
),
|
||||
);
|
||||
|
||||
public static $prefixesPsr0 = array (
|
||||
'P' =>
|
||||
array (
|
||||
'PHPExcel' =>
|
||||
array (
|
||||
0 => __DIR__ . '/..' . '/phpoffice/phpexcel/Classes',
|
||||
),
|
||||
),
|
||||
);
|
||||
|
||||
public static $classMap = array (
|
||||
'Composer\\InstalledVersions' => __DIR__ . '/..' . '/composer/InstalledVersions.php',
|
||||
'File_Iterator' => __DIR__ . '/..' . '/phpunit/php-file-iterator/src/Iterator.php',
|
||||
'File_Iterator_Facade' => __DIR__ . '/..' . '/phpunit/php-file-iterator/src/Facade.php',
|
||||
'File_Iterator_Factory' => __DIR__ . '/..' . '/phpunit/php-file-iterator/src/Factory.php',
|
||||
'PHPUnit\\Framework\\Assert' => __DIR__ . '/..' . '/phpunit/phpunit/src/ForwardCompatibility/Assert.php',
|
||||
'PHPUnit\\Framework\\AssertionFailedError' => __DIR__ . '/..' . '/phpunit/phpunit/src/ForwardCompatibility/AssertionFailedError.php',
|
||||
'PHPUnit\\Framework\\BaseTestListener' => __DIR__ . '/..' . '/phpunit/phpunit/src/ForwardCompatibility/BaseTestListener.php',
|
||||
'PHPUnit\\Framework\\Test' => __DIR__ . '/..' . '/phpunit/phpunit/src/ForwardCompatibility/Test.php',
|
||||
'PHPUnit\\Framework\\TestCase' => __DIR__ . '/..' . '/phpunit/phpunit/src/ForwardCompatibility/TestCase.php',
|
||||
'PHPUnit\\Framework\\TestListener' => __DIR__ . '/..' . '/phpunit/phpunit/src/ForwardCompatibility/TestListener.php',
|
||||
'PHPUnit\\Framework\\TestSuite' => __DIR__ . '/..' . '/phpunit/phpunit/src/ForwardCompatibility/TestSuite.php',
|
||||
'PHPUnit_Exception' => __DIR__ . '/..' . '/phpunit/phpunit/src/Exception.php',
|
||||
'PHPUnit_Extensions_GroupTestSuite' => __DIR__ . '/..' . '/phpunit/phpunit/src/Extensions/GroupTestSuite.php',
|
||||
'PHPUnit_Extensions_PhptTestCase' => __DIR__ . '/..' . '/phpunit/phpunit/src/Extensions/PhptTestCase.php',
|
||||
'PHPUnit_Extensions_PhptTestSuite' => __DIR__ . '/..' . '/phpunit/phpunit/src/Extensions/PhptTestSuite.php',
|
||||
'PHPUnit_Extensions_RepeatedTest' => __DIR__ . '/..' . '/phpunit/phpunit/src/Extensions/RepeatedTest.php',
|
||||
'PHPUnit_Extensions_TestDecorator' => __DIR__ . '/..' . '/phpunit/phpunit/src/Extensions/TestDecorator.php',
|
||||
'PHPUnit_Extensions_TicketListener' => __DIR__ . '/..' . '/phpunit/phpunit/src/Extensions/TicketListener.php',
|
||||
'PHPUnit_Framework_Assert' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Assert.php',
|
||||
'PHPUnit_Framework_AssertionFailedError' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/AssertionFailedError.php',
|
||||
'PHPUnit_Framework_BaseTestListener' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/BaseTestListener.php',
|
||||
'PHPUnit_Framework_CodeCoverageException' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/CodeCoverageException.php',
|
||||
'PHPUnit_Framework_Constraint' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint.php',
|
||||
'PHPUnit_Framework_Constraint_And' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/And.php',
|
||||
'PHPUnit_Framework_Constraint_ArrayHasKey' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/ArrayHasKey.php',
|
||||
'PHPUnit_Framework_Constraint_ArraySubset' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/ArraySubset.php',
|
||||
'PHPUnit_Framework_Constraint_Attribute' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/Attribute.php',
|
||||
'PHPUnit_Framework_Constraint_Callback' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/Callback.php',
|
||||
'PHPUnit_Framework_Constraint_ClassHasAttribute' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/ClassHasAttribute.php',
|
||||
'PHPUnit_Framework_Constraint_ClassHasStaticAttribute' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/ClassHasStaticAttribute.php',
|
||||
'PHPUnit_Framework_Constraint_Composite' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/Composite.php',
|
||||
'PHPUnit_Framework_Constraint_Count' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/Count.php',
|
||||
'PHPUnit_Framework_Constraint_Exception' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/Exception.php',
|
||||
'PHPUnit_Framework_Constraint_ExceptionCode' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/ExceptionCode.php',
|
||||
'PHPUnit_Framework_Constraint_ExceptionMessage' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/ExceptionMessage.php',
|
||||
'PHPUnit_Framework_Constraint_ExceptionMessageRegExp' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/ExceptionMessageRegExp.php',
|
||||
'PHPUnit_Framework_Constraint_FileExists' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/FileExists.php',
|
||||
'PHPUnit_Framework_Constraint_GreaterThan' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/GreaterThan.php',
|
||||
'PHPUnit_Framework_Constraint_IsAnything' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsAnything.php',
|
||||
'PHPUnit_Framework_Constraint_IsEmpty' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsEmpty.php',
|
||||
'PHPUnit_Framework_Constraint_IsEqual' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsEqual.php',
|
||||
'PHPUnit_Framework_Constraint_IsFalse' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsFalse.php',
|
||||
'PHPUnit_Framework_Constraint_IsIdentical' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsIdentical.php',
|
||||
'PHPUnit_Framework_Constraint_IsInstanceOf' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsInstanceOf.php',
|
||||
'PHPUnit_Framework_Constraint_IsJson' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsJson.php',
|
||||
'PHPUnit_Framework_Constraint_IsNull' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsNull.php',
|
||||
'PHPUnit_Framework_Constraint_IsTrue' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsTrue.php',
|
||||
'PHPUnit_Framework_Constraint_IsType' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/IsType.php',
|
||||
'PHPUnit_Framework_Constraint_JsonMatches' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/JsonMatches.php',
|
||||
'PHPUnit_Framework_Constraint_JsonMatches_ErrorMessageProvider' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/JsonMatches/ErrorMessageProvider.php',
|
||||
'PHPUnit_Framework_Constraint_LessThan' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/LessThan.php',
|
||||
'PHPUnit_Framework_Constraint_Not' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/Not.php',
|
||||
'PHPUnit_Framework_Constraint_ObjectHasAttribute' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/ObjectHasAttribute.php',
|
||||
'PHPUnit_Framework_Constraint_Or' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/Or.php',
|
||||
'PHPUnit_Framework_Constraint_PCREMatch' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/PCREMatch.php',
|
||||
'PHPUnit_Framework_Constraint_SameSize' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/SameSize.php',
|
||||
'PHPUnit_Framework_Constraint_StringContains' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/StringContains.php',
|
||||
'PHPUnit_Framework_Constraint_StringEndsWith' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/StringEndsWith.php',
|
||||
'PHPUnit_Framework_Constraint_StringMatches' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/StringMatches.php',
|
||||
'PHPUnit_Framework_Constraint_StringStartsWith' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/StringStartsWith.php',
|
||||
'PHPUnit_Framework_Constraint_TraversableContains' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/TraversableContains.php',
|
||||
'PHPUnit_Framework_Constraint_TraversableContainsOnly' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/TraversableContainsOnly.php',
|
||||
'PHPUnit_Framework_Constraint_Xor' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Constraint/Xor.php',
|
||||
'PHPUnit_Framework_Error' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Error.php',
|
||||
'PHPUnit_Framework_Error_Deprecated' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Error/Deprecated.php',
|
||||
'PHPUnit_Framework_Error_Notice' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Error/Notice.php',
|
||||
'PHPUnit_Framework_Error_Warning' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Error/Warning.php',
|
||||
'PHPUnit_Framework_Exception' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Exception.php',
|
||||
'PHPUnit_Framework_ExceptionWrapper' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/ExceptionWrapper.php',
|
||||
'PHPUnit_Framework_ExpectationFailedException' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/ExpectationFailedException.php',
|
||||
'PHPUnit_Framework_IncompleteTest' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/IncompleteTest.php',
|
||||
'PHPUnit_Framework_IncompleteTestCase' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/IncompleteTestCase.php',
|
||||
'PHPUnit_Framework_IncompleteTestError' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/IncompleteTestError.php',
|
||||
'PHPUnit_Framework_InvalidCoversTargetError' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/InvalidCoversTargetError.php',
|
||||
'PHPUnit_Framework_InvalidCoversTargetException' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/InvalidCoversTargetException.php',
|
||||
'PHPUnit_Framework_MockObject_BadMethodCallException' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Exception/BadMethodCallException.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_Identity' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/Identity.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_InvocationMocker' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/InvocationMocker.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_Match' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/Match.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_MethodNameMatch' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/MethodNameMatch.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_Namespace' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/Namespace.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_ParametersMatch' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/ParametersMatch.php',
|
||||
'PHPUnit_Framework_MockObject_Builder_Stub' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Builder/Stub.php',
|
||||
'PHPUnit_Framework_MockObject_Exception' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Exception/Exception.php',
|
||||
'PHPUnit_Framework_MockObject_Generator' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Generator.php',
|
||||
'PHPUnit_Framework_MockObject_Invocation' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Invocation.php',
|
||||
'PHPUnit_Framework_MockObject_InvocationMocker' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/InvocationMocker.php',
|
||||
'PHPUnit_Framework_MockObject_Invocation_Object' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Invocation/Object.php',
|
||||
'PHPUnit_Framework_MockObject_Invocation_Static' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Invocation/Static.php',
|
||||
'PHPUnit_Framework_MockObject_Invokable' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Invokable.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_AnyInvokedCount' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/AnyInvokedCount.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_AnyParameters' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/AnyParameters.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_ConsecutiveParameters' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/ConsecutiveParameters.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_Invocation' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/Invocation.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedAtIndex' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedAtIndex.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedAtLeastCount' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedAtLeastCount.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedAtLeastOnce' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedAtLeastOnce.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedAtMostCount' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedAtMostCount.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedCount' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedCount.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_InvokedRecorder' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/InvokedRecorder.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_MethodName' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/MethodName.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_Parameters' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/Parameters.php',
|
||||
'PHPUnit_Framework_MockObject_Matcher_StatelessInvocation' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Matcher/StatelessInvocation.php',
|
||||
'PHPUnit_Framework_MockObject_MockBuilder' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/MockBuilder.php',
|
||||
'PHPUnit_Framework_MockObject_MockObject' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/MockObject.php',
|
||||
'PHPUnit_Framework_MockObject_RuntimeException' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Exception/RuntimeException.php',
|
||||
'PHPUnit_Framework_MockObject_Stub' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ConsecutiveCalls' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ConsecutiveCalls.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_Exception' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/Exception.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_MatcherCollection' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/MatcherCollection.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_Return' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/Return.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ReturnArgument' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ReturnArgument.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ReturnCallback' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ReturnCallback.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ReturnSelf' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ReturnSelf.php',
|
||||
'PHPUnit_Framework_MockObject_Stub_ReturnValueMap' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Stub/ReturnValueMap.php',
|
||||
'PHPUnit_Framework_MockObject_Verifiable' => __DIR__ . '/..' . '/phpunit/phpunit-mock-objects/src/Framework/MockObject/Verifiable.php',
|
||||
'PHPUnit_Framework_OutputError' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/OutputError.php',
|
||||
'PHPUnit_Framework_RiskyTest' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/RiskyTest.php',
|
||||
'PHPUnit_Framework_RiskyTestError' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/RiskyTestError.php',
|
||||
'PHPUnit_Framework_SelfDescribing' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/SelfDescribing.php',
|
||||
'PHPUnit_Framework_SkippedTest' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/SkippedTest.php',
|
||||
'PHPUnit_Framework_SkippedTestCase' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/SkippedTestCase.php',
|
||||
'PHPUnit_Framework_SkippedTestError' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/SkippedTestError.php',
|
||||
'PHPUnit_Framework_SkippedTestSuiteError' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/SkippedTestSuiteError.php',
|
||||
'PHPUnit_Framework_SyntheticError' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/SyntheticError.php',
|
||||
'PHPUnit_Framework_Test' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Test.php',
|
||||
'PHPUnit_Framework_TestCase' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/TestCase.php',
|
||||
'PHPUnit_Framework_TestFailure' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/TestFailure.php',
|
||||
'PHPUnit_Framework_TestListener' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/TestListener.php',
|
||||
'PHPUnit_Framework_TestResult' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/TestResult.php',
|
||||
'PHPUnit_Framework_TestSuite' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/TestSuite.php',
|
||||
'PHPUnit_Framework_TestSuite_DataProvider' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/TestSuite/DataProvider.php',
|
||||
'PHPUnit_Framework_UnintentionallyCoveredCodeError' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/UnintentionallyCoveredCodeError.php',
|
||||
'PHPUnit_Framework_Warning' => __DIR__ . '/..' . '/phpunit/phpunit/src/Framework/Warning.php',
|
||||
'PHPUnit_Runner_BaseTestRunner' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/BaseTestRunner.php',
|
||||
'PHPUnit_Runner_Exception' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/Exception.php',
|
||||
'PHPUnit_Runner_Filter_Factory' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/Filter/Factory.php',
|
||||
'PHPUnit_Runner_Filter_GroupFilterIterator' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/Filter/Group.php',
|
||||
'PHPUnit_Runner_Filter_Group_Exclude' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/Filter/Group/Exclude.php',
|
||||
'PHPUnit_Runner_Filter_Group_Include' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/Filter/Group/Include.php',
|
||||
'PHPUnit_Runner_Filter_Test' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/Filter/Test.php',
|
||||
'PHPUnit_Runner_StandardTestSuiteLoader' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/StandardTestSuiteLoader.php',
|
||||
'PHPUnit_Runner_TestSuiteLoader' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/TestSuiteLoader.php',
|
||||
'PHPUnit_Runner_Version' => __DIR__ . '/..' . '/phpunit/phpunit/src/Runner/Version.php',
|
||||
'PHPUnit_TextUI_Command' => __DIR__ . '/..' . '/phpunit/phpunit/src/TextUI/Command.php',
|
||||
'PHPUnit_TextUI_ResultPrinter' => __DIR__ . '/..' . '/phpunit/phpunit/src/TextUI/ResultPrinter.php',
|
||||
'PHPUnit_TextUI_TestRunner' => __DIR__ . '/..' . '/phpunit/phpunit/src/TextUI/TestRunner.php',
|
||||
'PHPUnit_Util_Blacklist' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Blacklist.php',
|
||||
'PHPUnit_Util_Configuration' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Configuration.php',
|
||||
'PHPUnit_Util_ErrorHandler' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/ErrorHandler.php',
|
||||
'PHPUnit_Util_Fileloader' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Fileloader.php',
|
||||
'PHPUnit_Util_Filesystem' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Filesystem.php',
|
||||
'PHPUnit_Util_Filter' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Filter.php',
|
||||
'PHPUnit_Util_Getopt' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Getopt.php',
|
||||
'PHPUnit_Util_GlobalState' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/GlobalState.php',
|
||||
'PHPUnit_Util_InvalidArgumentHelper' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/InvalidArgumentHelper.php',
|
||||
'PHPUnit_Util_Log_JSON' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Log/JSON.php',
|
||||
'PHPUnit_Util_Log_JUnit' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Log/JUnit.php',
|
||||
'PHPUnit_Util_Log_TAP' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Log/TAP.php',
|
||||
'PHPUnit_Util_PHP' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/PHP.php',
|
||||
'PHPUnit_Util_PHP_Default' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/PHP/Default.php',
|
||||
'PHPUnit_Util_PHP_Windows' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/PHP/Windows.php',
|
||||
'PHPUnit_Util_Printer' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Printer.php',
|
||||
'PHPUnit_Util_Regex' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Regex.php',
|
||||
'PHPUnit_Util_String' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/String.php',
|
||||
'PHPUnit_Util_Test' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Test.php',
|
||||
'PHPUnit_Util_TestDox_NamePrettifier' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/TestDox/NamePrettifier.php',
|
||||
'PHPUnit_Util_TestDox_ResultPrinter' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/TestDox/ResultPrinter.php',
|
||||
'PHPUnit_Util_TestDox_ResultPrinter_HTML' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/TestDox/ResultPrinter/HTML.php',
|
||||
'PHPUnit_Util_TestDox_ResultPrinter_Text' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/TestDox/ResultPrinter/Text.php',
|
||||
'PHPUnit_Util_TestSuiteIterator' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/TestSuiteIterator.php',
|
||||
'PHPUnit_Util_Type' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/Type.php',
|
||||
'PHPUnit_Util_XML' => __DIR__ . '/..' . '/phpunit/phpunit/src/Util/XML.php',
|
||||
'PHP_CodeCoverage' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage.php',
|
||||
'PHP_CodeCoverage_Driver' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Driver.php',
|
||||
'PHP_CodeCoverage_Driver_HHVM' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Driver/HHVM.php',
|
||||
'PHP_CodeCoverage_Driver_PHPDBG' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Driver/PHPDBG.php',
|
||||
'PHP_CodeCoverage_Driver_Xdebug' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Driver/Xdebug.php',
|
||||
'PHP_CodeCoverage_Exception' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Exception.php',
|
||||
'PHP_CodeCoverage_Exception_UnintentionallyCoveredCode' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Exception/UnintentionallyCoveredCode.php',
|
||||
'PHP_CodeCoverage_Filter' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Filter.php',
|
||||
'PHP_CodeCoverage_Report_Clover' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Clover.php',
|
||||
'PHP_CodeCoverage_Report_Crap4j' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Crap4j.php',
|
||||
'PHP_CodeCoverage_Report_Factory' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Factory.php',
|
||||
'PHP_CodeCoverage_Report_HTML' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML.php',
|
||||
'PHP_CodeCoverage_Report_HTML_Renderer' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML/Renderer.php',
|
||||
'PHP_CodeCoverage_Report_HTML_Renderer_Dashboard' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML/Renderer/Dashboard.php',
|
||||
'PHP_CodeCoverage_Report_HTML_Renderer_Directory' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML/Renderer/Directory.php',
|
||||
'PHP_CodeCoverage_Report_HTML_Renderer_File' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/HTML/Renderer/File.php',
|
||||
'PHP_CodeCoverage_Report_Node' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Node.php',
|
||||
'PHP_CodeCoverage_Report_Node_Directory' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Node/Directory.php',
|
||||
'PHP_CodeCoverage_Report_Node_File' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Node/File.php',
|
||||
'PHP_CodeCoverage_Report_Node_Iterator' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Node/Iterator.php',
|
||||
'PHP_CodeCoverage_Report_PHP' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/PHP.php',
|
||||
'PHP_CodeCoverage_Report_Text' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/Text.php',
|
||||
'PHP_CodeCoverage_Report_XML' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML.php',
|
||||
'PHP_CodeCoverage_Report_XML_Directory' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Directory.php',
|
||||
'PHP_CodeCoverage_Report_XML_File' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File.php',
|
||||
'PHP_CodeCoverage_Report_XML_File_Coverage' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File/Coverage.php',
|
||||
'PHP_CodeCoverage_Report_XML_File_Method' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File/Method.php',
|
||||
'PHP_CodeCoverage_Report_XML_File_Report' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File/Report.php',
|
||||
'PHP_CodeCoverage_Report_XML_File_Unit' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/File/Unit.php',
|
||||
'PHP_CodeCoverage_Report_XML_Node' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Node.php',
|
||||
'PHP_CodeCoverage_Report_XML_Project' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Project.php',
|
||||
'PHP_CodeCoverage_Report_XML_Tests' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Tests.php',
|
||||
'PHP_CodeCoverage_Report_XML_Totals' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Report/XML/Totals.php',
|
||||
'PHP_CodeCoverage_Util' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Util.php',
|
||||
'PHP_CodeCoverage_Util_InvalidArgumentHelper' => __DIR__ . '/..' . '/phpunit/php-code-coverage/src/CodeCoverage/Util/InvalidArgumentHelper.php',
|
||||
'PHP_Timer' => __DIR__ . '/..' . '/phpunit/php-timer/src/Timer.php',
|
||||
'PHP_Token' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_TokenWithScope' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_TokenWithScopeAndVisibility' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ABSTRACT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AMPERSAND' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AND_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ARRAY' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ARRAY_CAST' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AS' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ASYNC' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_AWAIT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BACKTICK' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BAD_CHARACTER' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BOOLEAN_AND' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BOOLEAN_OR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BOOL_CAST' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_BREAK' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CALLABLE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CARET' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CASE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CATCH' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CHARACTER' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLASS' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLASS_C' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLASS_NAME_CONSTANT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLONE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLOSE_BRACKET' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLOSE_CURLY' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLOSE_SQUARE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CLOSE_TAG' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COALESCE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COLON' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COMMA' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COMMENT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_COMPILER_HALT_OFFSET' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CONCAT_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CONST' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CONSTANT_ENCAPSED_STRING' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CONTINUE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_CURLY_OPEN' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DEC' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DECLARE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DEFAULT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DIR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DIV' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DIV_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DNUMBER' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DO' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOC_COMMENT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOLLAR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOLLAR_OPEN_CURLY_BRACES' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOUBLE_ARROW' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOUBLE_CAST' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOUBLE_COLON' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_DOUBLE_QUOTES' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ECHO' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ELLIPSIS' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ELSE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ELSEIF' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EMPTY' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENCAPSED_AND_WHITESPACE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDDECLARE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDFOR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDFOREACH' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDIF' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDSWITCH' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENDWHILE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_END_HEREDOC' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ENUM' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EQUALS' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EVAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EXCLAMATION_MARK' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EXIT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_EXTENDS' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FILE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FINAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FINALLY' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FOR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FOREACH' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FUNCTION' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_FUNC_C' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_GLOBAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_GOTO' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_GT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_HALT_COMPILER' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IF' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IMPLEMENTS' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IN' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INC' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INCLUDE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INCLUDE_ONCE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INLINE_HTML' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INSTANCEOF' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INSTEADOF' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INTERFACE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_INT_CAST' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ISSET' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_GREATER_OR_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_IDENTICAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_NOT_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_NOT_IDENTICAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_IS_SMALLER_OR_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_Includes' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_JOIN' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LAMBDA_ARROW' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LAMBDA_CP' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LAMBDA_OP' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LINE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LIST' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LNUMBER' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LOGICAL_AND' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LOGICAL_OR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LOGICAL_XOR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_LT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_METHOD_C' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MINUS' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MINUS_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MOD_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MULT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_MUL_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NAMESPACE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NEW' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NS_C' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NS_SEPARATOR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NULLSAFE_OBJECT_OPERATOR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_NUM_STRING' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OBJECT_CAST' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OBJECT_OPERATOR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_ONUMBER' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_BRACKET' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_CURLY' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_SQUARE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_TAG' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OPEN_TAG_WITH_ECHO' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_OR_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PAAMAYIM_NEKUDOTAYIM' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PERCENT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PIPE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PLUS' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PLUS_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_POW' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_POW_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PRINT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PRIVATE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PROTECTED' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_PUBLIC' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_QUESTION_MARK' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_REQUIRE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_REQUIRE_ONCE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_RETURN' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SEMICOLON' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SHAPE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SL_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SPACESHIP' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SR_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_START_HEREDOC' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_STATIC' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_STRING' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_STRING_CAST' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_STRING_VARNAME' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SUPER' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_SWITCH' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_Stream' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token/Stream.php',
|
||||
'PHP_Token_Stream_CachingFactory' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token/Stream/CachingFactory.php',
|
||||
'PHP_Token_THROW' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TILDE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TRAIT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TRAIT_C' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TRY' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TYPE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TYPELIST_GT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_TYPELIST_LT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_UNSET' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_UNSET_CAST' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_USE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_USE_FUNCTION' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_VAR' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_VARIABLE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_WHERE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_WHILE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_WHITESPACE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_ATTRIBUTE' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_CATEGORY' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_CATEGORY_LABEL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_CHILDREN' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_LABEL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_REQUIRED' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_TAG_GT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_TAG_LT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XHP_TEXT' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_XOR_EQUAL' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_YIELD' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PHP_Token_YIELD_FROM' => __DIR__ . '/..' . '/phpunit/php-token-stream/src/Token.php',
|
||||
'PclZip' => __DIR__ . '/..' . '/pclzip/pclzip/pclzip.lib.php',
|
||||
'SebastianBergmann\\Comparator\\ArrayComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/ArrayComparator.php',
|
||||
'SebastianBergmann\\Comparator\\Comparator' => __DIR__ . '/..' . '/sebastian/comparator/src/Comparator.php',
|
||||
'SebastianBergmann\\Comparator\\ComparisonFailure' => __DIR__ . '/..' . '/sebastian/comparator/src/ComparisonFailure.php',
|
||||
'SebastianBergmann\\Comparator\\DOMNodeComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/DOMNodeComparator.php',
|
||||
'SebastianBergmann\\Comparator\\DateTimeComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/DateTimeComparator.php',
|
||||
'SebastianBergmann\\Comparator\\DoubleComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/DoubleComparator.php',
|
||||
'SebastianBergmann\\Comparator\\ExceptionComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/ExceptionComparator.php',
|
||||
'SebastianBergmann\\Comparator\\Factory' => __DIR__ . '/..' . '/sebastian/comparator/src/Factory.php',
|
||||
'SebastianBergmann\\Comparator\\MockObjectComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/MockObjectComparator.php',
|
||||
'SebastianBergmann\\Comparator\\NumericComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/NumericComparator.php',
|
||||
'SebastianBergmann\\Comparator\\ObjectComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/ObjectComparator.php',
|
||||
'SebastianBergmann\\Comparator\\ResourceComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/ResourceComparator.php',
|
||||
'SebastianBergmann\\Comparator\\ScalarComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/ScalarComparator.php',
|
||||
'SebastianBergmann\\Comparator\\SplObjectStorageComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/SplObjectStorageComparator.php',
|
||||
'SebastianBergmann\\Comparator\\TypeComparator' => __DIR__ . '/..' . '/sebastian/comparator/src/TypeComparator.php',
|
||||
'SebastianBergmann\\Diff\\Chunk' => __DIR__ . '/..' . '/sebastian/diff/src/Chunk.php',
|
||||
'SebastianBergmann\\Diff\\Diff' => __DIR__ . '/..' . '/sebastian/diff/src/Diff.php',
|
||||
'SebastianBergmann\\Diff\\Differ' => __DIR__ . '/..' . '/sebastian/diff/src/Differ.php',
|
||||
'SebastianBergmann\\Diff\\LCS\\LongestCommonSubsequence' => __DIR__ . '/..' . '/sebastian/diff/src/LCS/LongestCommonSubsequence.php',
|
||||
'SebastianBergmann\\Diff\\LCS\\MemoryEfficientImplementation' => __DIR__ . '/..' . '/sebastian/diff/src/LCS/MemoryEfficientLongestCommonSubsequenceImplementation.php',
|
||||
'SebastianBergmann\\Diff\\LCS\\TimeEfficientImplementation' => __DIR__ . '/..' . '/sebastian/diff/src/LCS/TimeEfficientLongestCommonSubsequenceImplementation.php',
|
||||
'SebastianBergmann\\Diff\\Line' => __DIR__ . '/..' . '/sebastian/diff/src/Line.php',
|
||||
'SebastianBergmann\\Diff\\Parser' => __DIR__ . '/..' . '/sebastian/diff/src/Parser.php',
|
||||
'SebastianBergmann\\Environment\\Console' => __DIR__ . '/..' . '/sebastian/environment/src/Console.php',
|
||||
'SebastianBergmann\\Environment\\Runtime' => __DIR__ . '/..' . '/sebastian/environment/src/Runtime.php',
|
||||
'SebastianBergmann\\Exporter\\Exporter' => __DIR__ . '/..' . '/sebastian/exporter/src/Exporter.php',
|
||||
'SebastianBergmann\\GlobalState\\Blacklist' => __DIR__ . '/..' . '/sebastian/global-state/src/Blacklist.php',
|
||||
'SebastianBergmann\\GlobalState\\CodeExporter' => __DIR__ . '/..' . '/sebastian/global-state/src/CodeExporter.php',
|
||||
'SebastianBergmann\\GlobalState\\Exception' => __DIR__ . '/..' . '/sebastian/global-state/src/Exception.php',
|
||||
'SebastianBergmann\\GlobalState\\Restorer' => __DIR__ . '/..' . '/sebastian/global-state/src/Restorer.php',
|
||||
'SebastianBergmann\\GlobalState\\RuntimeException' => __DIR__ . '/..' . '/sebastian/global-state/src/RuntimeException.php',
|
||||
'SebastianBergmann\\GlobalState\\Snapshot' => __DIR__ . '/..' . '/sebastian/global-state/src/Snapshot.php',
|
||||
'SebastianBergmann\\RecursionContext\\Context' => __DIR__ . '/..' . '/sebastian/recursion-context/src/Context.php',
|
||||
'SebastianBergmann\\RecursionContext\\Exception' => __DIR__ . '/..' . '/sebastian/recursion-context/src/Exception.php',
|
||||
'SebastianBergmann\\RecursionContext\\InvalidArgumentException' => __DIR__ . '/..' . '/sebastian/recursion-context/src/InvalidArgumentException.php',
|
||||
'SebastianBergmann\\Version' => __DIR__ . '/..' . '/sebastian/version/src/Version.php',
|
||||
'Text_Template' => __DIR__ . '/..' . '/phpunit/php-text-template/src/Template.php',
|
||||
);
|
||||
|
||||
public static function getInitializer(ClassLoader $loader)
|
||||
{
|
||||
return \Closure::bind(function () use ($loader) {
|
||||
$loader->prefixLengthsPsr4 = ComposerStaticInitc184f419bfb1b5c35adb1bc36e45b15e::$prefixLengthsPsr4;
|
||||
$loader->prefixDirsPsr4 = ComposerStaticInitc184f419bfb1b5c35adb1bc36e45b15e::$prefixDirsPsr4;
|
||||
$loader->prefixesPsr0 = ComposerStaticInitc184f419bfb1b5c35adb1bc36e45b15e::$prefixesPsr0;
|
||||
$loader->classMap = ComposerStaticInitc184f419bfb1b5c35adb1bc36e45b15e::$classMap;
|
||||
|
||||
}, null, ClassLoader::class);
|
||||
}
|
||||
}
|
File diff suppressed because it is too large
Load Diff
@ -0,0 +1,420 @@
|
||||
<?php return array (
|
||||
'root' =>
|
||||
array (
|
||||
'pretty_version' => 'dev-master',
|
||||
'version' => 'dev-master',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '7088f600d8002ab34d9457e48bfdaf2050cebf68',
|
||||
'name' => 'topthink/think',
|
||||
),
|
||||
'versions' =>
|
||||
array (
|
||||
'doctrine/instantiator' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.0',
|
||||
'version' => '1.4.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'd56bf6102915de5702778fe20f2de3b2fe570b5b',
|
||||
),
|
||||
'markbaker/complex' =>
|
||||
array (
|
||||
'pretty_version' => '1.5.0',
|
||||
'version' => '1.5.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'c3131244e29c08d44fefb49e0dd35021e9e39dd2',
|
||||
),
|
||||
'markbaker/matrix' =>
|
||||
array (
|
||||
'pretty_version' => '1.2.3',
|
||||
'version' => '1.2.3.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '44bb1ab01811116f01fe216ab37d921dccc6c10d',
|
||||
),
|
||||
'pclzip/pclzip' =>
|
||||
array (
|
||||
'pretty_version' => '2.8.2',
|
||||
'version' => '2.8.2.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '19dd1de9d3f5fc4d7d70175b4c344dee329f45fd',
|
||||
),
|
||||
'phpdocumentor/reflection-common' =>
|
||||
array (
|
||||
'pretty_version' => '2.2.0',
|
||||
'version' => '2.2.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '1d01c49d4ed62f25aa84a747ad35d5a16924662b',
|
||||
),
|
||||
'phpdocumentor/reflection-docblock' =>
|
||||
array (
|
||||
'pretty_version' => '5.2.2',
|
||||
'version' => '5.2.2.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '069a785b2141f5bcf49f3e353548dc1cce6df556',
|
||||
),
|
||||
'phpdocumentor/type-resolver' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.0',
|
||||
'version' => '1.4.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '6a467b8989322d92aa1c8bf2bebcc6e5c2ba55c0',
|
||||
),
|
||||
'phpoffice/common' =>
|
||||
array (
|
||||
'pretty_version' => '0.2.9',
|
||||
'version' => '0.2.9.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'edb5d32b1e3400a35a5c91e2539ed6f6ce925e4d',
|
||||
),
|
||||
'phpoffice/phpexcel' =>
|
||||
array (
|
||||
'pretty_version' => '1.8.0',
|
||||
'version' => '1.8.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'e69a5e4d0ffa7fb6f171859e0a04346e580df30b',
|
||||
),
|
||||
'phpoffice/phpspreadsheet' =>
|
||||
array (
|
||||
'pretty_version' => '1.8.0',
|
||||
'version' => '1.8.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '0e6238c69e863b58aeece61e48ea032696c6dccd',
|
||||
),
|
||||
'phpoffice/phpword' =>
|
||||
array (
|
||||
'pretty_version' => '0.17.0',
|
||||
'version' => '0.17.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'b8346af548d399acd9e30fc76ab0c55c2fec03a5',
|
||||
),
|
||||
'phpspec/prophecy' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.10.3',
|
||||
'version' => '1.10.3.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '451c3cd1418cf640de218914901e51b064abb093',
|
||||
),
|
||||
'phpunit/php-code-coverage' =>
|
||||
array (
|
||||
'pretty_version' => '2.2.4',
|
||||
'version' => '2.2.4.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'eabf68b476ac7d0f73793aada060f1c1a9bf8979',
|
||||
),
|
||||
'phpunit/php-file-iterator' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.5',
|
||||
'version' => '1.4.5.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '730b01bc3e867237eaac355e06a36b85dd93a8b4',
|
||||
),
|
||||
'phpunit/php-text-template' =>
|
||||
array (
|
||||
'pretty_version' => '1.2.1',
|
||||
'version' => '1.2.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '31f8b717e51d9a2afca6c9f046f5d69fc27c8686',
|
||||
),
|
||||
'phpunit/php-timer' =>
|
||||
array (
|
||||
'pretty_version' => '1.0.9',
|
||||
'version' => '1.0.9.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '3dcf38ca72b158baf0bc245e9184d3fdffa9c46f',
|
||||
),
|
||||
'phpunit/php-token-stream' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.12',
|
||||
'version' => '1.4.12.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '1ce90ba27c42e4e44e6d8458241466380b51fa16',
|
||||
),
|
||||
'phpunit/phpunit' =>
|
||||
array (
|
||||
'pretty_version' => '4.8.36',
|
||||
'version' => '4.8.36.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '46023de9a91eec7dfb06cc56cb4e260017298517',
|
||||
),
|
||||
'phpunit/phpunit-mock-objects' =>
|
||||
array (
|
||||
'pretty_version' => '2.3.8',
|
||||
'version' => '2.3.8.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'ac8e7a3db35738d56ee9a76e78a4e03d97628983',
|
||||
),
|
||||
'psr/simple-cache' =>
|
||||
array (
|
||||
'pretty_version' => '1.0.1',
|
||||
'version' => '1.0.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '408d5eafb83c57f6365a3ca330ff23aa4a5fa39b',
|
||||
),
|
||||
'sebastian/comparator' =>
|
||||
array (
|
||||
'pretty_version' => '1.2.4',
|
||||
'version' => '1.2.4.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '2b7424b55f5047b47ac6e5ccb20b2aea4011d9be',
|
||||
),
|
||||
'sebastian/diff' =>
|
||||
array (
|
||||
'pretty_version' => '1.4.3',
|
||||
'version' => '1.4.3.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '7f066a26a962dbe58ddea9f72a4e82874a3975a4',
|
||||
),
|
||||
'sebastian/environment' =>
|
||||
array (
|
||||
'pretty_version' => '1.3.8',
|
||||
'version' => '1.3.8.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'be2c607e43ce4c89ecd60e75c6a85c126e754aea',
|
||||
),
|
||||
'sebastian/exporter' =>
|
||||
array (
|
||||
'pretty_version' => '1.2.2',
|
||||
'version' => '1.2.2.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '42c4c2eec485ee3e159ec9884f95b431287edde4',
|
||||
),
|
||||
'sebastian/global-state' =>
|
||||
array (
|
||||
'pretty_version' => '1.1.1',
|
||||
'version' => '1.1.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'bc37d50fea7d017d3d340f230811c9f1d7280af4',
|
||||
),
|
||||
'sebastian/recursion-context' =>
|
||||
array (
|
||||
'pretty_version' => '1.0.5',
|
||||
'version' => '1.0.5.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'b19cc3298482a335a95f3016d2f8a6950f0fbcd7',
|
||||
),
|
||||
'sebastian/version' =>
|
||||
array (
|
||||
'pretty_version' => '1.0.6',
|
||||
'version' => '1.0.6.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '58b3a85e7999757d6ad81c787a1fbf5ff6c628c6',
|
||||
),
|
||||
'symfony/dom-crawler' =>
|
||||
array (
|
||||
'pretty_version' => 'v2.8.52',
|
||||
'version' => '2.8.52.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '2cdc7d3909eea6f982a6298d2e9ab7db01b6403c',
|
||||
),
|
||||
'symfony/polyfill-ctype' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.22.1',
|
||||
'version' => '1.22.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'c6c942b1ac76c82448322025e084cadc56048b4e',
|
||||
),
|
||||
'symfony/polyfill-mbstring' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.22.1',
|
||||
'version' => '1.22.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '5232de97ee3b75b0360528dae24e73db49566ab1',
|
||||
),
|
||||
'symfony/yaml' =>
|
||||
array (
|
||||
'pretty_version' => 'v3.4.47',
|
||||
'version' => '3.4.47.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '88289caa3c166321883f67fe5130188ebbb47094',
|
||||
),
|
||||
'topthink/framework' =>
|
||||
array (
|
||||
'pretty_version' => 'v5.0.24',
|
||||
'version' => '5.0.24.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'c255c22b2f5fa30f320ecf6c1d29f7740eb3e8be',
|
||||
),
|
||||
'topthink/think' =>
|
||||
array (
|
||||
'pretty_version' => 'dev-master',
|
||||
'version' => 'dev-master',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '7088f600d8002ab34d9457e48bfdaf2050cebf68',
|
||||
),
|
||||
'topthink/think-helper' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.7',
|
||||
'version' => '1.0.7.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '5f92178606c8ce131d36b37a57c58eb71e55f019',
|
||||
),
|
||||
'topthink/think-image' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.7',
|
||||
'version' => '1.0.7.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '8586cf47f117481c6d415b20f7dedf62e79d5512',
|
||||
),
|
||||
'topthink/think-installer' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.14',
|
||||
'version' => '1.0.14.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'eae1740ac264a55c06134b6685dfb9f837d004d1',
|
||||
),
|
||||
'topthink/think-migration' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.1.1',
|
||||
'version' => '1.1.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '8e489f8d38a39876690c0e00fcf9a54ae92c4d3d',
|
||||
),
|
||||
'topthink/think-mongo' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.8.5',
|
||||
'version' => '1.8.5.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '657cc79bd5f090a58b0cc83776073dd69c83a3d1',
|
||||
),
|
||||
'topthink/think-queue' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.1.6',
|
||||
'version' => '1.1.6.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '250650eb0e8ea5af4cfdc7ae46f3f4e0a24ac245',
|
||||
),
|
||||
'topthink/think-sae' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.1',
|
||||
'version' => '1.1.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'e31ee4ec073c0ffc5dbc7292f8268661e5265091',
|
||||
),
|
||||
'topthink/think-testing' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.6',
|
||||
'version' => '1.0.6.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'e89794e5c58aa5587f7b08038e9468150870b185',
|
||||
),
|
||||
'topthink/think-worker' =>
|
||||
array (
|
||||
'pretty_version' => 'v1.0.0',
|
||||
'version' => '1.0.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'f48ca8e5d8324aace15cdbf0f3de751397ef803f',
|
||||
),
|
||||
'webmozart/assert' =>
|
||||
array (
|
||||
'pretty_version' => '1.10.0',
|
||||
'version' => '1.10.0.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '6964c76c7804814a842473e0c8fd15bab0f18e25',
|
||||
),
|
||||
'workerman/workerman' =>
|
||||
array (
|
||||
'pretty_version' => 'v3.5.31',
|
||||
'version' => '3.5.31.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => 'b73ddc45b3c7299f330923a2bde23ca6e974fd96',
|
||||
),
|
||||
'zendframework/zend-escaper' =>
|
||||
array (
|
||||
'pretty_version' => '2.6.1',
|
||||
'version' => '2.6.1.0',
|
||||
'aliases' =>
|
||||
array (
|
||||
),
|
||||
'reference' => '3801caa21b0ca6aca57fa1c42b08d35c395ebd5f',
|
||||
),
|
||||
),
|
||||
);
|
@ -0,0 +1,26 @@
|
||||
<?php
|
||||
|
||||
// platform_check.php @generated by Composer
|
||||
|
||||
$issues = array();
|
||||
|
||||
if (!(PHP_VERSION_ID >= 70200)) {
|
||||
$issues[] = 'Your Composer dependencies require a PHP version ">= 7.2.0". You are running ' . PHP_VERSION . '.';
|
||||
}
|
||||
|
||||
if ($issues) {
|
||||
if (!headers_sent()) {
|
||||
header('HTTP/1.1 500 Internal Server Error');
|
||||
}
|
||||
if (!ini_get('display_errors')) {
|
||||
if (PHP_SAPI === 'cli' || PHP_SAPI === 'phpdbg') {
|
||||
fwrite(STDERR, 'Composer detected issues in your platform:' . PHP_EOL.PHP_EOL . implode(PHP_EOL, $issues) . PHP_EOL.PHP_EOL);
|
||||
} elseif (!headers_sent()) {
|
||||
echo 'Composer detected issues in your platform:' . PHP_EOL.PHP_EOL . str_replace('You are running '.PHP_VERSION.'.', '', implode(PHP_EOL, $issues)) . PHP_EOL.PHP_EOL;
|
||||
}
|
||||
}
|
||||
trigger_error(
|
||||
'Composer detected issues in your platform: ' . implode(' ', $issues),
|
||||
E_USER_ERROR
|
||||
);
|
||||
}
|
@ -0,0 +1,41 @@
|
||||
{
|
||||
"active": true,
|
||||
"name": "Instantiator",
|
||||
"slug": "instantiator",
|
||||
"docsSlug": "doctrine-instantiator",
|
||||
"codePath": "/src",
|
||||
"versions": [
|
||||
{
|
||||
"name": "1.4",
|
||||
"branchName": "master",
|
||||
"slug": "latest",
|
||||
"upcoming": true
|
||||
},
|
||||
{
|
||||
"name": "1.3",
|
||||
"branchName": "1.3.x",
|
||||
"slug": "1.3",
|
||||
"aliases": [
|
||||
"current",
|
||||
"stable"
|
||||
],
|
||||
"maintained": true,
|
||||
"current": true
|
||||
},
|
||||
{
|
||||
"name": "1.2",
|
||||
"branchName": "1.2.x",
|
||||
"slug": "1.2"
|
||||
},
|
||||
{
|
||||
"name": "1.1",
|
||||
"branchName": "1.1.x",
|
||||
"slug": "1.1"
|
||||
},
|
||||
{
|
||||
"name": "1.0",
|
||||
"branchName": "1.0.x",
|
||||
"slug": "1.0"
|
||||
}
|
||||
]
|
||||
}
|
@ -0,0 +1,3 @@
|
||||
patreon: phpdoctrine
|
||||
tidelift: packagist/doctrine%2Finstantiator
|
||||
custom: https://www.doctrine-project.org/sponsorship.html
|
@ -0,0 +1,48 @@
|
||||
|
||||
name: "Coding Standards"
|
||||
|
||||
on:
|
||||
pull_request:
|
||||
branches:
|
||||
- "*.x"
|
||||
push:
|
||||
branches:
|
||||
- "*.x"
|
||||
|
||||
env:
|
||||
COMPOSER_ROOT_VERSION: "1.4"
|
||||
|
||||
jobs:
|
||||
coding-standards:
|
||||
name: "Coding Standards"
|
||||
runs-on: "ubuntu-20.04"
|
||||
|
||||
strategy:
|
||||
matrix:
|
||||
php-version:
|
||||
- "7.4"
|
||||
|
||||
steps:
|
||||
- name: "Checkout"
|
||||
uses: "actions/checkout@v2"
|
||||
|
||||
- name: "Install PHP"
|
||||
uses: "shivammathur/setup-php@v2"
|
||||
with:
|
||||
coverage: "none"
|
||||
php-version: "${{ matrix.php-version }}"
|
||||
tools: "cs2pr"
|
||||
|
||||
- name: "Cache dependencies installed with Composer"
|
||||
uses: "actions/cache@v2"
|
||||
with:
|
||||
path: "~/.composer/cache"
|
||||
key: "php-${{ matrix.php-version }}-composer-locked-${{ hashFiles('composer.lock') }}"
|
||||
restore-keys: "php-${{ matrix.php-version }}-composer-locked-"
|
||||
|
||||
- name: "Install dependencies with Composer"
|
||||
run: "composer install --no-interaction --no-progress"
|
||||
|
||||
# https://github.com/doctrine/.github/issues/3
|
||||
- name: "Run PHP_CodeSniffer"
|
||||
run: "vendor/bin/phpcs -q --no-colors --report=checkstyle | cs2pr"
|
@ -0,0 +1,91 @@
|
||||
|
||||
name: "Continuous Integration"
|
||||
|
||||
on:
|
||||
pull_request:
|
||||
branches:
|
||||
- "*.x"
|
||||
push:
|
||||
branches:
|
||||
- "*.x"
|
||||
|
||||
env:
|
||||
fail-fast: true
|
||||
COMPOSER_ROOT_VERSION: "1.4"
|
||||
|
||||
jobs:
|
||||
phpunit:
|
||||
name: "PHPUnit with SQLite"
|
||||
runs-on: "ubuntu-20.04"
|
||||
|
||||
strategy:
|
||||
matrix:
|
||||
php-version:
|
||||
- "7.1"
|
||||
- "7.2"
|
||||
- "7.3"
|
||||
- "7.4"
|
||||
- "8.0"
|
||||
|
||||
steps:
|
||||
- name: "Checkout"
|
||||
uses: "actions/checkout@v2"
|
||||
with:
|
||||
fetch-depth: 2
|
||||
|
||||
- name: "Install PHP with XDebug"
|
||||
uses: "shivammathur/setup-php@v2"
|
||||
if: "${{ matrix.php-version == '7.1' }}"
|
||||
with:
|
||||
php-version: "${{ matrix.php-version }}"
|
||||
coverage: "xdebug"
|
||||
ini-values: "zend.assertions=1"
|
||||
|
||||
- name: "Install PHP with PCOV"
|
||||
uses: "shivammathur/setup-php@v2"
|
||||
if: "${{ matrix.php-version != '7.1' }}"
|
||||
with:
|
||||
php-version: "${{ matrix.php-version }}"
|
||||
coverage: "pcov"
|
||||
ini-values: "zend.assertions=1"
|
||||
|
||||
- name: "Cache dependencies installed with composer"
|
||||
uses: "actions/cache@v2"
|
||||
with:
|
||||
path: "~/.composer/cache"
|
||||
key: "php-${{ matrix.php-version }}-composer-locked-${{ hashFiles('composer.lock') }}"
|
||||
restore-keys: "php-${{ matrix.php-version }}-composer-locked-"
|
||||
|
||||
- name: "Install dependencies with composer"
|
||||
run: "composer update --no-interaction --no-progress"
|
||||
|
||||
- name: "Run PHPUnit"
|
||||
run: "vendor/bin/phpunit --coverage-clover=coverage.xml"
|
||||
|
||||
- name: "Upload coverage file"
|
||||
uses: "actions/upload-artifact@v2"
|
||||
with:
|
||||
name: "phpunit-${{ matrix.php-version }}.coverage"
|
||||
path: "coverage.xml"
|
||||
|
||||
upload_coverage:
|
||||
name: "Upload coverage to Codecov"
|
||||
runs-on: "ubuntu-20.04"
|
||||
needs:
|
||||
- "phpunit"
|
||||
|
||||
steps:
|
||||
- name: "Checkout"
|
||||
uses: "actions/checkout@v2"
|
||||
with:
|
||||
fetch-depth: 2
|
||||
|
||||
- name: "Download coverage files"
|
||||
uses: "actions/download-artifact@v2"
|
||||
with:
|
||||
path: "reports"
|
||||
|
||||
- name: "Upload to Codecov"
|
||||
uses: "codecov/codecov-action@v1"
|
||||
with:
|
||||
directory: reports
|
@ -0,0 +1,50 @@
|
||||
|
||||
name: "Performance benchmark"
|
||||
|
||||
on:
|
||||
pull_request:
|
||||
branches:
|
||||
- "*.x"
|
||||
push:
|
||||
branches:
|
||||
- "*.x"
|
||||
|
||||
env:
|
||||
fail-fast: true
|
||||
COMPOSER_ROOT_VERSION: "1.4"
|
||||
|
||||
jobs:
|
||||
phpbench:
|
||||
name: "PHPBench"
|
||||
runs-on: "ubuntu-20.04"
|
||||
|
||||
strategy:
|
||||
matrix:
|
||||
php-version:
|
||||
- "7.4"
|
||||
|
||||
steps:
|
||||
- name: "Checkout"
|
||||
uses: "actions/checkout@v2"
|
||||
with:
|
||||
fetch-depth: 2
|
||||
|
||||
- name: "Install PHP"
|
||||
uses: "shivammathur/setup-php@v2"
|
||||
with:
|
||||
php-version: "${{ matrix.php-version }}"
|
||||
coverage: "pcov"
|
||||
ini-values: "zend.assertions=1"
|
||||
|
||||
- name: "Cache dependencies installed with composer"
|
||||
uses: "actions/cache@v2"
|
||||
with:
|
||||
path: "~/.composer/cache"
|
||||
key: "php-${{ matrix.php-version }}-composer-locked-${{ hashFiles('composer.lock') }}"
|
||||
restore-keys: "php-${{ matrix.php-version }}-composer-locked-"
|
||||
|
||||
- name: "Install dependencies with composer"
|
||||
run: "composer update --no-interaction --no-progress"
|
||||
|
||||
- name: "Run PHPBench"
|
||||
run: "php ./vendor/bin/phpbench run --iterations=3 --warmup=1 --report=aggregate"
|
@ -0,0 +1,45 @@
|
||||
name: "Automatic Releases"
|
||||
|
||||
on:
|
||||
milestone:
|
||||
types:
|
||||
- "closed"
|
||||
|
||||
jobs:
|
||||
release:
|
||||
name: "Git tag, release & create merge-up PR"
|
||||
runs-on: "ubuntu-20.04"
|
||||
|
||||
steps:
|
||||
- name: "Checkout"
|
||||
uses: "actions/checkout@v2"
|
||||
|
||||
- name: "Release"
|
||||
uses: "laminas/automatic-releases@v1"
|
||||
with:
|
||||
command-name: "laminas:automatic-releases:release"
|
||||
env:
|
||||
"GITHUB_TOKEN": ${{ secrets.GITHUB_TOKEN }}
|
||||
"SIGNING_SECRET_KEY": ${{ secrets.SIGNING_SECRET_KEY }}
|
||||
"GIT_AUTHOR_NAME": ${{ secrets.GIT_AUTHOR_NAME }}
|
||||
"GIT_AUTHOR_EMAIL": ${{ secrets.GIT_AUTHOR_EMAIL }}
|
||||
|
||||
- name: "Create Merge-Up Pull Request"
|
||||
uses: "laminas/automatic-releases@v1"
|
||||
with:
|
||||
command-name: "laminas:automatic-releases:create-merge-up-pull-request"
|
||||
env:
|
||||
"GITHUB_TOKEN": ${{ secrets.GITHUB_TOKEN }}
|
||||
"SIGNING_SECRET_KEY": ${{ secrets.SIGNING_SECRET_KEY }}
|
||||
"GIT_AUTHOR_NAME": ${{ secrets.GIT_AUTHOR_NAME }}
|
||||
"GIT_AUTHOR_EMAIL": ${{ secrets.GIT_AUTHOR_EMAIL }}
|
||||
|
||||
- name: "Create new milestones"
|
||||
uses: "laminas/automatic-releases@v1"
|
||||
with:
|
||||
command-name: "laminas:automatic-releases:create-milestones"
|
||||
env:
|
||||
"GITHUB_TOKEN": ${{ secrets.GITHUB_TOKEN }}
|
||||
"SIGNING_SECRET_KEY": ${{ secrets.SIGNING_SECRET_KEY }}
|
||||
"GIT_AUTHOR_NAME": ${{ secrets.GIT_AUTHOR_NAME }}
|
||||
"GIT_AUTHOR_EMAIL": ${{ secrets.GIT_AUTHOR_EMAIL }}
|
@ -0,0 +1,47 @@
|
||||
|
||||
name: "Static Analysis"
|
||||
|
||||
on:
|
||||
pull_request:
|
||||
branches:
|
||||
- "*.x"
|
||||
push:
|
||||
branches:
|
||||
- "*.x"
|
||||
|
||||
env:
|
||||
COMPOSER_ROOT_VERSION: "1.4"
|
||||
|
||||
jobs:
|
||||
static-analysis-phpstan:
|
||||
name: "Static Analysis with PHPStan"
|
||||
runs-on: "ubuntu-20.04"
|
||||
|
||||
strategy:
|
||||
matrix:
|
||||
php-version:
|
||||
- "7.4"
|
||||
|
||||
steps:
|
||||
- name: "Checkout code"
|
||||
uses: "actions/checkout@v2"
|
||||
|
||||
- name: "Install PHP"
|
||||
uses: "shivammathur/setup-php@v2"
|
||||
with:
|
||||
coverage: "none"
|
||||
php-version: "${{ matrix.php-version }}"
|
||||
tools: "cs2pr"
|
||||
|
||||
- name: "Cache dependencies installed with composer"
|
||||
uses: "actions/cache@v2"
|
||||
with:
|
||||
path: "~/.composer/cache"
|
||||
key: "php-${{ matrix.php-version }}-composer-locked-${{ hashFiles('composer.lock') }}"
|
||||
restore-keys: "php-${{ matrix.php-version }}-composer-locked-"
|
||||
|
||||
- name: "Install dependencies with composer"
|
||||
run: "composer install --no-interaction --no-progress"
|
||||
|
||||
- name: "Run a static analysis with phpstan/phpstan"
|
||||
run: "vendor/bin/phpstan analyse --error-format=checkstyle | cs2pr"
|
@ -0,0 +1,35 @@
|
||||
# Contributing
|
||||
|
||||
* Follow the [Doctrine Coding Standard](https://github.com/doctrine/coding-standard)
|
||||
* The project will follow strict [object calisthenics](http://www.slideshare.net/guilhermeblanco/object-calisthenics-applied-to-php)
|
||||
* Any contribution must provide tests for additional introduced conditions
|
||||
* Any un-confirmed issue needs a failing test case before being accepted
|
||||
* Pull requests must be sent from a new hotfix/feature branch, not from `master`.
|
||||
|
||||
## Installation
|
||||
|
||||
To install the project and run the tests, you need to clone it first:
|
||||
|
||||
```sh
|
||||
$ git clone git://github.com/doctrine/instantiator.git
|
||||
```
|
||||
|
||||
You will then need to run a composer installation:
|
||||
|
||||
```sh
|
||||
$ cd Instantiator
|
||||
$ curl -s https://getcomposer.org/installer | php
|
||||
$ php composer.phar update
|
||||
```
|
||||
|
||||
## Testing
|
||||
|
||||
The PHPUnit version to be used is the one installed as a dev- dependency via composer:
|
||||
|
||||
```sh
|
||||
$ ./vendor/bin/phpunit
|
||||
```
|
||||
|
||||
Accepted coverage for new contributions is 80%. Any contribution not satisfying this requirement
|
||||
won't be merged.
|
||||
|
@ -0,0 +1,19 @@
|
||||
Copyright (c) 2014 Doctrine Project
|
||||
|
||||
Permission is hereby granted, free of charge, to any person obtaining a copy of
|
||||
this software and associated documentation files (the "Software"), to deal in
|
||||
the Software without restriction, including without limitation the rights to
|
||||
use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies
|
||||
of the Software, and to permit persons to whom the Software is furnished to do
|
||||
so, subject to the following conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be included in all
|
||||
copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
||||
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
||||
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
||||
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
||||
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
||||
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
||||
SOFTWARE.
|
@ -0,0 +1,38 @@
|
||||
# Instantiator
|
||||
|
||||
This library provides a way of avoiding usage of constructors when instantiating PHP classes.
|
||||
|
||||
[](https://travis-ci.org/doctrine/instantiator)
|
||||
[](https://codecov.io/gh/doctrine/instantiator/branch/master)
|
||||
[](https://www.versioneye.com/package/php--doctrine--instantiator)
|
||||
|
||||
[](https://packagist.org/packages/doctrine/instantiator)
|
||||
[](https://packagist.org/packages/doctrine/instantiator)
|
||||
|
||||
## Installation
|
||||
|
||||
The suggested installation method is via [composer](https://getcomposer.org/):
|
||||
|
||||
```sh
|
||||
php composer.phar require "doctrine/instantiator:~1.0.3"
|
||||
```
|
||||
|
||||
## Usage
|
||||
|
||||
The instantiator is able to create new instances of any class without using the constructor or any API of the class
|
||||
itself:
|
||||
|
||||
```php
|
||||
$instantiator = new \Doctrine\Instantiator\Instantiator();
|
||||
|
||||
$instance = $instantiator->instantiate(\My\ClassName\Here::class);
|
||||
```
|
||||
|
||||
## Contributing
|
||||
|
||||
Please read the [CONTRIBUTING.md](CONTRIBUTING.md) contents if you wish to help out!
|
||||
|
||||
## Credits
|
||||
|
||||
This library was migrated from [ocramius/instantiator](https://github.com/Ocramius/Instantiator), which
|
||||
has been donated to the doctrine organization, and which is now deprecated in favour of this package.
|
@ -0,0 +1,42 @@
|
||||
{
|
||||
"name": "doctrine/instantiator",
|
||||
"description": "A small, lightweight utility to instantiate objects in PHP without invoking their constructors",
|
||||
"type": "library",
|
||||
"license": "MIT",
|
||||
"homepage": "https://www.doctrine-project.org/projects/instantiator.html",
|
||||
"keywords": [
|
||||
"instantiate",
|
||||
"constructor"
|
||||
],
|
||||
"authors": [
|
||||
{
|
||||
"name": "Marco Pivetta",
|
||||
"email": "ocramius@gmail.com",
|
||||
"homepage": "https://ocramius.github.io/"
|
||||
}
|
||||
],
|
||||
"require": {
|
||||
"php": "^7.1 || ^8.0"
|
||||
},
|
||||
"require-dev": {
|
||||
"ext-phar": "*",
|
||||
"ext-pdo": "*",
|
||||
"doctrine/coding-standard": "^8.0",
|
||||
"phpbench/phpbench": "^0.13 || 1.0.0-alpha2",
|
||||
"phpstan/phpstan": "^0.12",
|
||||
"phpstan/phpstan-phpunit": "^0.12",
|
||||
"phpunit/phpunit": "^7.0 || ^8.0 || ^9.0"
|
||||
},
|
||||
"autoload": {
|
||||
"psr-4": {
|
||||
"Doctrine\\Instantiator\\": "src/Doctrine/Instantiator/"
|
||||
}
|
||||
},
|
||||
"autoload-dev": {
|
||||
"psr-0": {
|
||||
"DoctrineTest\\InstantiatorPerformance\\": "tests",
|
||||
"DoctrineTest\\InstantiatorTest\\": "tests",
|
||||
"DoctrineTest\\InstantiatorTestAsset\\": "tests"
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,4 @@
|
||||
.. toctree::
|
||||
:depth: 3
|
||||
|
||||
index
|
@ -0,0 +1,4 @@
|
||||
{
|
||||
"bootstrap": "vendor/autoload.php",
|
||||
"path": "tests/DoctrineTest/InstantiatorPerformance"
|
||||
}
|
@ -0,0 +1,50 @@
|
||||
<?xml version="1.0"?>
|
||||
<ruleset>
|
||||
<arg name="basepath" value="."/>
|
||||
<arg name="extensions" value="php"/>
|
||||
<arg name="parallel" value="80"/>
|
||||
<arg name="cache" value=".phpcs-cache"/>
|
||||
<arg name="colors"/>
|
||||
|
||||
<!-- Ignore warnings, show progress of the run and show sniff names -->
|
||||
<arg value="nps"/>
|
||||
|
||||
<file>src</file>
|
||||
<file>tests</file>
|
||||
|
||||
<rule ref="Doctrine">
|
||||
<exclude name="SlevomatCodingStandard.TypeHints.DeclareStrictTypes"/>
|
||||
<exclude name="SlevomatCodingStandard.TypeHints.TypeHintDeclaration.MissingParameterTypeHint"/>
|
||||
<exclude name="SlevomatCodingStandard.TypeHints.TypeHintDeclaration.MissingReturnTypeHint"/>
|
||||
<exclude name="SlevomatCodingStandard.Exceptions.ReferenceThrowableOnly.ReferencedGeneralException"/>
|
||||
</rule>
|
||||
|
||||
<!-- Disable the rules that will require PHP 7.4 -->
|
||||
<rule ref="SlevomatCodingStandard.TypeHints.PropertyTypeHint">
|
||||
<properties>
|
||||
<property name="enableNativeTypeHint" value="false"/>
|
||||
</properties>
|
||||
</rule>
|
||||
|
||||
<rule ref="SlevomatCodingStandard.TypeHints.ParameterTypeHint.MissingNativeTypeHint">
|
||||
<exclude-pattern>*/src/*</exclude-pattern>
|
||||
</rule>
|
||||
|
||||
<rule ref="SlevomatCodingStandard.TypeHints.ReturnTypeHint.MissingNativeTypeHint">
|
||||
<exclude-pattern>*/src/*</exclude-pattern>
|
||||
</rule>
|
||||
|
||||
<rule ref="SlevomatCodingStandard.Classes.SuperfluousAbstractClassNaming">
|
||||
<exclude-pattern>tests/DoctrineTest/InstantiatorTestAsset/AbstractClassAsset.php</exclude-pattern>
|
||||
</rule>
|
||||
|
||||
<rule ref="SlevomatCodingStandard.Classes.SuperfluousExceptionNaming">
|
||||
<exclude-pattern>src/Doctrine/Instantiator/Exception/UnexpectedValueException.php</exclude-pattern>
|
||||
<exclude-pattern>src/Doctrine/Instantiator/Exception/InvalidArgumentException.php</exclude-pattern>
|
||||
</rule>
|
||||
|
||||
<rule ref="SlevomatCodingStandard.Classes.SuperfluousInterfaceNaming">
|
||||
<exclude-pattern>src/Doctrine/Instantiator/Exception/ExceptionInterface.php</exclude-pattern>
|
||||
<exclude-pattern>src/Doctrine/Instantiator/InstantiatorInterface.php</exclude-pattern>
|
||||
</rule>
|
||||
</ruleset>
|
@ -0,0 +1,15 @@
|
||||
includes:
|
||||
- vendor/phpstan/phpstan-phpunit/extension.neon
|
||||
- vendor/phpstan/phpstan-phpunit/rules.neon
|
||||
|
||||
parameters:
|
||||
level: max
|
||||
paths:
|
||||
- src
|
||||
- tests
|
||||
|
||||
ignoreErrors:
|
||||
# dynamic properties confuse static analysis
|
||||
-
|
||||
message: '#Access to an undefined property object::\$foo\.#'
|
||||
path: '*/tests/DoctrineTest/InstantiatorTest/InstantiatorTest.php'
|
@ -0,0 +1,12 @@
|
||||
<?php
|
||||
|
||||
namespace Doctrine\Instantiator\Exception;
|
||||
|
||||
use Throwable;
|
||||
|
||||
/**
|
||||
* Base exception marker interface for the instantiator component
|
||||
*/
|
||||
interface ExceptionInterface extends Throwable
|
||||
{
|
||||
}
|
@ -0,0 +1,41 @@
|
||||
<?php
|
||||
|
||||
namespace Doctrine\Instantiator\Exception;
|
||||
|
||||
use InvalidArgumentException as BaseInvalidArgumentException;
|
||||
use ReflectionClass;
|
||||
|
||||
use function interface_exists;
|
||||
use function sprintf;
|
||||
use function trait_exists;
|
||||
|
||||
/**
|
||||
* Exception for invalid arguments provided to the instantiator
|
||||
*/
|
||||
class InvalidArgumentException extends BaseInvalidArgumentException implements ExceptionInterface
|
||||
{
|
||||
public static function fromNonExistingClass(string $className): self
|
||||
{
|
||||
if (interface_exists($className)) {
|
||||
return new self(sprintf('The provided type "%s" is an interface, and can not be instantiated', $className));
|
||||
}
|
||||
|
||||
if (trait_exists($className)) {
|
||||
return new self(sprintf('The provided type "%s" is a trait, and can not be instantiated', $className));
|
||||
}
|
||||
|
||||
return new self(sprintf('The provided class "%s" does not exist', $className));
|
||||
}
|
||||
|
||||
/**
|
||||
* @template T of object
|
||||
* @phpstan-param ReflectionClass<T> $reflectionClass
|
||||
*/
|
||||
public static function fromAbstractClass(ReflectionClass $reflectionClass): self
|
||||
{
|
||||
return new self(sprintf(
|
||||
'The provided class "%s" is abstract, and can not be instantiated',
|
||||
$reflectionClass->getName()
|
||||
));
|
||||
}
|
||||
}
|
@ -0,0 +1,57 @@
|
||||
<?php
|
||||
|
||||
namespace Doctrine\Instantiator\Exception;
|
||||
|
||||
use Exception;
|
||||
use ReflectionClass;
|
||||
use UnexpectedValueException as BaseUnexpectedValueException;
|
||||
|
||||
use function sprintf;
|
||||
|
||||
/**
|
||||
* Exception for given parameters causing invalid/unexpected state on instantiation
|
||||
*/
|
||||
class UnexpectedValueException extends BaseUnexpectedValueException implements ExceptionInterface
|
||||
{
|
||||
/**
|
||||
* @template T of object
|
||||
* @phpstan-param ReflectionClass<T> $reflectionClass
|
||||
*/
|
||||
public static function fromSerializationTriggeredException(
|
||||
ReflectionClass $reflectionClass,
|
||||
Exception $exception
|
||||
): self {
|
||||
return new self(
|
||||
sprintf(
|
||||
'An exception was raised while trying to instantiate an instance of "%s" via un-serialization',
|
||||
$reflectionClass->getName()
|
||||
),
|
||||
0,
|
||||
$exception
|
||||
);
|
||||
}
|
||||
|
||||
/**
|
||||
* @template T of object
|
||||
* @phpstan-param ReflectionClass<T> $reflectionClass
|
||||
*/
|
||||
public static function fromUncleanUnSerialization(
|
||||
ReflectionClass $reflectionClass,
|
||||
string $errorString,
|
||||
int $errorCode,
|
||||
string $errorFile,
|
||||
int $errorLine
|
||||
): self {
|
||||
return new self(
|
||||
sprintf(
|
||||
'Could not produce an instance of "%s" via un-serialization, since an error was triggered '
|
||||
. 'in file "%s" at line "%d"',
|
||||
$reflectionClass->getName(),
|
||||
$errorFile,
|
||||
$errorLine
|
||||
),
|
||||
0,
|
||||
new Exception($errorString, $errorCode)
|
||||
);
|
||||
}
|
||||
}
|
@ -0,0 +1,232 @@
|
||||
<?php
|
||||
|
||||
namespace Doctrine\Instantiator;
|
||||
|
||||
use ArrayIterator;
|
||||
use Doctrine\Instantiator\Exception\InvalidArgumentException;
|
||||
use Doctrine\Instantiator\Exception\UnexpectedValueException;
|
||||
use Exception;
|
||||
use ReflectionClass;
|
||||
use ReflectionException;
|
||||
use Serializable;
|
||||
|
||||
use function class_exists;
|
||||
use function is_subclass_of;
|
||||
use function restore_error_handler;
|
||||
use function set_error_handler;
|
||||
use function sprintf;
|
||||
use function strlen;
|
||||
use function unserialize;
|
||||
|
||||
final class Instantiator implements InstantiatorInterface
|
||||
{
|
||||
/**
|
||||
* Markers used internally by PHP to define whether {@see \unserialize} should invoke
|
||||
* the method {@see \Serializable::unserialize()} when dealing with classes implementing
|
||||
* the {@see \Serializable} interface.
|
||||
*/
|
||||
public const SERIALIZATION_FORMAT_USE_UNSERIALIZER = 'C';
|
||||
public const SERIALIZATION_FORMAT_AVOID_UNSERIALIZER = 'O';
|
||||
|
||||
/**
|
||||
* Used to instantiate specific classes, indexed by class name.
|
||||
*
|
||||
* @var callable[]
|
||||
*/
|
||||
private static $cachedInstantiators = [];
|
||||
|
||||
/**
|
||||
* Array of objects that can directly be cloned, indexed by class name.
|
||||
*
|
||||
* @var object[]
|
||||
*/
|
||||
private static $cachedCloneables = [];
|
||||
|
||||
/**
|
||||
* {@inheritDoc}
|
||||
*/
|
||||
public function instantiate($className)
|
||||
{
|
||||
if (isset(self::$cachedCloneables[$className])) {
|
||||
return clone self::$cachedCloneables[$className];
|
||||
}
|
||||
|
||||
if (isset(self::$cachedInstantiators[$className])) {
|
||||
$factory = self::$cachedInstantiators[$className];
|
||||
|
||||
return $factory();
|
||||
}
|
||||
|
||||
return $this->buildAndCacheFromFactory($className);
|
||||
}
|
||||
|
||||
/**
|
||||
* Builds the requested object and caches it in static properties for performance
|
||||
*
|
||||
* @return object
|
||||
*
|
||||
* @template T of object
|
||||
* @phpstan-param class-string<T> $className
|
||||
*
|
||||
* @phpstan-return T
|
||||
*/
|
||||
private function buildAndCacheFromFactory(string $className)
|
||||
{
|
||||
$factory = self::$cachedInstantiators[$className] = $this->buildFactory($className);
|
||||
$instance = $factory();
|
||||
|
||||
if ($this->isSafeToClone(new ReflectionClass($instance))) {
|
||||
self::$cachedCloneables[$className] = clone $instance;
|
||||
}
|
||||
|
||||
return $instance;
|
||||
}
|
||||
|
||||
/**
|
||||
* Builds a callable capable of instantiating the given $className without
|
||||
* invoking its constructor.
|
||||
*
|
||||
* @throws InvalidArgumentException
|
||||
* @throws UnexpectedValueException
|
||||
* @throws ReflectionException
|
||||
*
|
||||
* @template T of object
|
||||
* @phpstan-param class-string<T> $className
|
||||
*
|
||||
* @phpstan-return callable(): T
|
||||
*/
|
||||
private function buildFactory(string $className): callable
|
||||
{
|
||||
$reflectionClass = $this->getReflectionClass($className);
|
||||
|
||||
if ($this->isInstantiableViaReflection($reflectionClass)) {
|
||||
return [$reflectionClass, 'newInstanceWithoutConstructor'];
|
||||
}
|
||||
|
||||
$serializedString = sprintf(
|
||||
'%s:%d:"%s":0:{}',
|
||||
is_subclass_of($className, Serializable::class) ? self::SERIALIZATION_FORMAT_USE_UNSERIALIZER : self::SERIALIZATION_FORMAT_AVOID_UNSERIALIZER,
|
||||
strlen($className),
|
||||
$className
|
||||
);
|
||||
|
||||
$this->checkIfUnSerializationIsSupported($reflectionClass, $serializedString);
|
||||
|
||||
return static function () use ($serializedString) {
|
||||
return unserialize($serializedString);
|
||||
};
|
||||
}
|
||||
|
||||
/**
|
||||
* @throws InvalidArgumentException
|
||||
* @throws ReflectionException
|
||||
*
|
||||
* @template T of object
|
||||
* @phpstan-param class-string<T> $className
|
||||
*
|
||||
* @phpstan-return ReflectionClass<T>
|
||||
*/
|
||||
private function getReflectionClass(string $className): ReflectionClass
|
||||
{
|
||||
if (! class_exists($className)) {
|
||||
throw InvalidArgumentException::fromNonExistingClass($className);
|
||||
}
|
||||
|
||||
$reflection = new ReflectionClass($className);
|
||||
|
||||
if ($reflection->isAbstract()) {
|
||||
throw InvalidArgumentException::fromAbstractClass($reflection);
|
||||
}
|
||||
|
||||
return $reflection;
|
||||
}
|
||||
|
||||
/**
|
||||
* @throws UnexpectedValueException
|
||||
*
|
||||
* @template T of object
|
||||
* @phpstan-param ReflectionClass<T> $reflectionClass
|
||||
*/
|
||||
private function checkIfUnSerializationIsSupported(ReflectionClass $reflectionClass, string $serializedString): void
|
||||
{
|
||||
set_error_handler(static function (int $code, string $message, string $file, int $line) use ($reflectionClass, &$error): bool {
|
||||
$error = UnexpectedValueException::fromUncleanUnSerialization(
|
||||
$reflectionClass,
|
||||
$message,
|
||||
$code,
|
||||
$file,
|
||||
$line
|
||||
);
|
||||
|
||||
return true;
|
||||
});
|
||||
|
||||
try {
|
||||
$this->attemptInstantiationViaUnSerialization($reflectionClass, $serializedString);
|
||||
} finally {
|
||||
restore_error_handler();
|
||||
}
|
||||
|
||||
if ($error) {
|
||||
throw $error;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* @throws UnexpectedValueException
|
||||
*
|
||||
* @template T of object
|
||||
* @phpstan-param ReflectionClass<T> $reflectionClass
|
||||
*/
|
||||
private function attemptInstantiationViaUnSerialization(ReflectionClass $reflectionClass, string $serializedString): void
|
||||
{
|
||||
try {
|
||||
unserialize($serializedString);
|
||||
} catch (Exception $exception) {
|
||||
throw UnexpectedValueException::fromSerializationTriggeredException($reflectionClass, $exception);
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* @template T of object
|
||||
* @phpstan-param ReflectionClass<T> $reflectionClass
|
||||
*/
|
||||
private function isInstantiableViaReflection(ReflectionClass $reflectionClass): bool
|
||||
{
|
||||
return ! ($this->hasInternalAncestors($reflectionClass) && $reflectionClass->isFinal());
|
||||
}
|
||||
|
||||
/**
|
||||
* Verifies whether the given class is to be considered internal
|
||||
*
|
||||
* @template T of object
|
||||
* @phpstan-param ReflectionClass<T> $reflectionClass
|
||||
*/
|
||||
private function hasInternalAncestors(ReflectionClass $reflectionClass): bool
|
||||
{
|
||||
do {
|
||||
if ($reflectionClass->isInternal()) {
|
||||
return true;
|
||||
}
|
||||
|
||||
$reflectionClass = $reflectionClass->getParentClass();
|
||||
} while ($reflectionClass);
|
||||
|
||||
return false;
|
||||
}
|
||||
|
||||
/**
|
||||
* Checks if a class is cloneable
|
||||
*
|
||||
* Classes implementing `__clone` cannot be safely cloned, as that may cause side-effects.
|
||||
*
|
||||
* @template T of object
|
||||
* @phpstan-param ReflectionClass<T> $reflectionClass
|
||||
*/
|
||||
private function isSafeToClone(ReflectionClass $reflectionClass): bool
|
||||
{
|
||||
return $reflectionClass->isCloneable()
|
||||
&& ! $reflectionClass->hasMethod('__clone')
|
||||
&& ! $reflectionClass->isSubclassOf(ArrayIterator::class);
|
||||
}
|
||||
}
|
@ -0,0 +1,23 @@
|
||||
<?php
|
||||
|
||||
namespace Doctrine\Instantiator;
|
||||
|
||||
use Doctrine\Instantiator\Exception\ExceptionInterface;
|
||||
|
||||
/**
|
||||
* Instantiator provides utility methods to build objects without invoking their constructors
|
||||
*/
|
||||
interface InstantiatorInterface
|
||||
{
|
||||
/**
|
||||
* @param string $className
|
||||
*
|
||||
* @return object
|
||||
*
|
||||
* @throws ExceptionInterface
|
||||
*
|
||||
* @template T of object
|
||||
* @phpstan-param class-string<T> $className
|
||||
*/
|
||||
public function instantiate($className);
|
||||
}
|
@ -0,0 +1,156 @@
|
||||
PHPComplex
|
||||
==========
|
||||
|
||||
---
|
||||
|
||||
PHP Class for handling Complex numbers
|
||||
|
||||
Master: [](http://travis-ci.org/MarkBaker/PHPComplex)
|
||||
|
||||
Develop: [](http://travis-ci.org/MarkBaker/PHPComplex)
|
||||
|
||||
[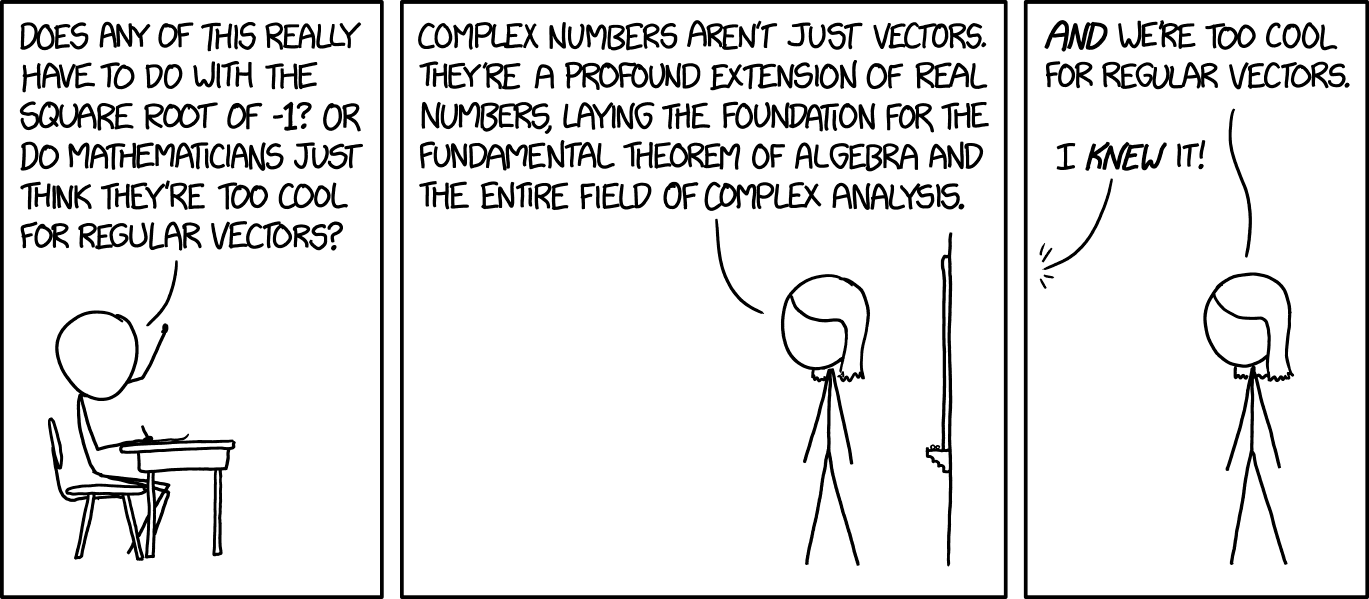](https://xkcd.com/2028/)
|
||||
|
||||
---
|
||||
|
||||
The library currently provides the following operations:
|
||||
|
||||
- addition
|
||||
- subtraction
|
||||
- multiplication
|
||||
- division
|
||||
- division by
|
||||
- division into
|
||||
|
||||
together with functions for
|
||||
|
||||
- theta (polar theta angle)
|
||||
- rho (polar distance/radius)
|
||||
- conjugate
|
||||
* negative
|
||||
- inverse (1 / complex)
|
||||
- cos (cosine)
|
||||
- acos (inverse cosine)
|
||||
- cosh (hyperbolic cosine)
|
||||
- acosh (inverse hyperbolic cosine)
|
||||
- sin (sine)
|
||||
- asin (inverse sine)
|
||||
- sinh (hyperbolic sine)
|
||||
- asinh (inverse hyperbolic sine)
|
||||
- sec (secant)
|
||||
- asec (inverse secant)
|
||||
- sech (hyperbolic secant)
|
||||
- asech (inverse hyperbolic secant)
|
||||
- csc (cosecant)
|
||||
- acsc (inverse cosecant)
|
||||
- csch (hyperbolic secant)
|
||||
- acsch (inverse hyperbolic secant)
|
||||
- tan (tangent)
|
||||
- atan (inverse tangent)
|
||||
- tanh (hyperbolic tangent)
|
||||
- atanh (inverse hyperbolic tangent)
|
||||
- cot (cotangent)
|
||||
- acot (inverse cotangent)
|
||||
- coth (hyperbolic cotangent)
|
||||
- acoth (inverse hyperbolic cotangent)
|
||||
- sqrt (square root)
|
||||
- exp (exponential)
|
||||
- ln (natural log)
|
||||
- log10 (base-10 log)
|
||||
- log2 (base-2 log)
|
||||
- pow (raised to the power of a real number)
|
||||
|
||||
|
||||
---
|
||||
|
||||
# Usage
|
||||
|
||||
To create a new complex object, you can provide either the real, imaginary and suffix parts as individual values, or as an array of values passed passed to the constructor; or a string representing the value. e.g
|
||||
|
||||
```
|
||||
$real = 1.23;
|
||||
$imaginary = -4.56;
|
||||
$suffix = 'i';
|
||||
|
||||
$complexObject = new Complex\Complex($real, $imaginary, $suffix);
|
||||
```
|
||||
or
|
||||
```
|
||||
$real = 1.23;
|
||||
$imaginary = -4.56;
|
||||
$suffix = 'i';
|
||||
|
||||
$arguments = [$real, $imaginary, $suffix];
|
||||
|
||||
$complexObject = new Complex\Complex($arguments);
|
||||
```
|
||||
or
|
||||
```
|
||||
$complexString = '1.23-4.56i';
|
||||
|
||||
$complexObject = new Complex\Complex($complexString);
|
||||
```
|
||||
|
||||
Complex objects are immutable: whenever you call a method or pass a complex value to a function that returns a complex value, a new Complex object will be returned, and the original will remain unchanged.
|
||||
This also allows you to chain multiple methods as you would for a fluent interface (as long as they are methods that will return a Complex result).
|
||||
|
||||
## Performing Mathematical Operations
|
||||
|
||||
To perform mathematical operations with Complex values, you can call the appropriate method against a complex value, passing other values as arguments
|
||||
|
||||
```
|
||||
$complexString1 = '1.23-4.56i';
|
||||
$complexString2 = '2.34+5.67i';
|
||||
|
||||
$complexObject = new Complex\Complex($complexString1);
|
||||
echo $complexObject->add($complexString2);
|
||||
```
|
||||
or pass all values to the appropriate function
|
||||
```
|
||||
$complexString1 = '1.23-4.56i';
|
||||
$complexString2 = '2.34+5.67i';
|
||||
|
||||
echo Complex\add($complexString1, $complexString2);
|
||||
```
|
||||
If you want to perform the same operation against multiple values (e.g. to add three or more complex numbers), then you can pass multiple arguments to any of the operations.
|
||||
|
||||
You can pass these arguments as Complex objects, or as an array or string that will parse to a complex object.
|
||||
|
||||
## Using functions
|
||||
|
||||
When calling any of the available functions for a complex value, you can either call the relevant method for the Complex object
|
||||
```
|
||||
$complexString = '1.23-4.56i';
|
||||
|
||||
$complexObject = new Complex\Complex($complexString);
|
||||
echo $complexObject->sinh();
|
||||
```
|
||||
or you can call the function as you would in procedural code, passing the Complex object as an argument
|
||||
```
|
||||
$complexString = '1.23-4.56i';
|
||||
|
||||
$complexObject = new Complex\Complex($complexString);
|
||||
echo Complex\sinh($complexObject);
|
||||
```
|
||||
When called procedurally using the function, you can pass in the argument as a Complex object, or as an array or string that will parse to a complex object.
|
||||
```
|
||||
$complexString = '1.23-4.56i';
|
||||
|
||||
echo Complex\sinh($complexString);
|
||||
```
|
||||
|
||||
In the case of the `pow()` function (the only implemented function that requires an additional argument) you need to pass both arguments when calling the function procedurally
|
||||
|
||||
```
|
||||
$complexString = '1.23-4.56i';
|
||||
|
||||
$complexObject = new Complex\Complex($complexString);
|
||||
echo Complex\pow($complexObject, 2);
|
||||
```
|
||||
or pass the additional argument when calling the method
|
||||
```
|
||||
$complexString = '1.23-4.56i';
|
||||
|
||||
$complexObject = new Complex\Complex($complexString);
|
||||
echo $complexObject->pow(2);
|
||||
```
|
@ -0,0 +1,53 @@
|
||||
<?php
|
||||
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
*
|
||||
* Autoloader for Complex classes
|
||||
*
|
||||
* @package Complex
|
||||
* @copyright Copyright (c) 2014 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
class Autoloader
|
||||
{
|
||||
/**
|
||||
* Register the Autoloader with SPL
|
||||
*
|
||||
*/
|
||||
public static function Register()
|
||||
{
|
||||
if (function_exists('__autoload')) {
|
||||
// Register any existing autoloader function with SPL, so we don't get any clashes
|
||||
spl_autoload_register('__autoload');
|
||||
}
|
||||
// Register ourselves with SPL
|
||||
return spl_autoload_register(['Complex\\Autoloader', 'Load']);
|
||||
}
|
||||
|
||||
|
||||
/**
|
||||
* Autoload a class identified by name
|
||||
*
|
||||
* @param string $pClassName Name of the object to load
|
||||
*/
|
||||
public static function Load($pClassName)
|
||||
{
|
||||
if ((class_exists($pClassName, false)) || (strpos($pClassName, 'Complex\\') !== 0)) {
|
||||
// Either already loaded, or not a Complex class request
|
||||
return false;
|
||||
}
|
||||
|
||||
$pClassFilePath = __DIR__ . DIRECTORY_SEPARATOR .
|
||||
'src' . DIRECTORY_SEPARATOR .
|
||||
str_replace(['Complex\\', '\\'], ['', '/'], $pClassName) .
|
||||
'.php';
|
||||
|
||||
if ((file_exists($pClassFilePath) === false) || (is_readable($pClassFilePath) === false)) {
|
||||
// Can't load
|
||||
return false;
|
||||
}
|
||||
require($pClassFilePath);
|
||||
}
|
||||
}
|
@ -0,0 +1,38 @@
|
||||
<?php
|
||||
|
||||
include_once __DIR__ . '/Autoloader.php';
|
||||
|
||||
\Complex\Autoloader::Register();
|
||||
|
||||
|
||||
abstract class FilesystemRegexFilter extends RecursiveRegexIterator
|
||||
{
|
||||
protected $regex;
|
||||
public function __construct(RecursiveIterator $it, $regex)
|
||||
{
|
||||
$this->regex = $regex;
|
||||
parent::__construct($it, $regex);
|
||||
}
|
||||
}
|
||||
|
||||
class FilenameFilter extends FilesystemRegexFilter
|
||||
{
|
||||
// Filter files against the regex
|
||||
public function accept()
|
||||
{
|
||||
return (!$this->isFile() || preg_match($this->regex, $this->getFilename()));
|
||||
}
|
||||
}
|
||||
|
||||
|
||||
$srcFolder = __DIR__ . DIRECTORY_SEPARATOR . 'src';
|
||||
$srcDirectory = new RecursiveDirectoryIterator($srcFolder);
|
||||
|
||||
$filteredFileList = new FilenameFilter($srcDirectory, '/(?:php)$/i');
|
||||
$filteredFileList = new FilenameFilter($filteredFileList, '/^(?!.*(Complex|Exception)\.php).*$/i');
|
||||
|
||||
foreach (new RecursiveIteratorIterator($filteredFileList) as $file) {
|
||||
if ($file->isFile()) {
|
||||
include_once $file;
|
||||
}
|
||||
}
|
@ -0,0 +1,390 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Class for the management of Complex numbers
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Complex Number object.
|
||||
*
|
||||
* @package Complex
|
||||
*
|
||||
* @method float abs()
|
||||
* @method Complex acos()
|
||||
* @method Complex acosh()
|
||||
* @method Complex acot()
|
||||
* @method Complex acoth()
|
||||
* @method Complex acsc()
|
||||
* @method Complex acsch()
|
||||
* @method float argument()
|
||||
* @method Complex asec()
|
||||
* @method Complex asech()
|
||||
* @method Complex asin()
|
||||
* @method Complex asinh()
|
||||
* @method Complex atan()
|
||||
* @method Complex atanh()
|
||||
* @method Complex conjugate()
|
||||
* @method Complex cos()
|
||||
* @method Complex cosh()
|
||||
* @method Complex cot()
|
||||
* @method Complex coth()
|
||||
* @method Complex csc()
|
||||
* @method Complex csch()
|
||||
* @method Complex exp()
|
||||
* @method Complex inverse()
|
||||
* @method Complex ln()
|
||||
* @method Complex log2()
|
||||
* @method Complex log10()
|
||||
* @method Complex negative()
|
||||
* @method Complex pow(int|float $power)
|
||||
* @method float rho()
|
||||
* @method Complex sec()
|
||||
* @method Complex sech()
|
||||
* @method Complex sin()
|
||||
* @method Complex sinh()
|
||||
* @method Complex sqrt()
|
||||
* @method Complex tan()
|
||||
* @method Complex tanh()
|
||||
* @method float theta()
|
||||
* @method Complex add(...$complexValues)
|
||||
* @method Complex subtract(...$complexValues)
|
||||
* @method Complex multiply(...$complexValues)
|
||||
* @method Complex divideby(...$complexValues)
|
||||
* @method Complex divideinto(...$complexValues)
|
||||
*/
|
||||
class Complex
|
||||
{
|
||||
/**
|
||||
* @constant Euler's Number.
|
||||
*/
|
||||
const EULER = 2.7182818284590452353602874713526624977572;
|
||||
|
||||
/**
|
||||
* @constant Regexp to split an input string into real and imaginary components and suffix
|
||||
*/
|
||||
const NUMBER_SPLIT_REGEXP =
|
||||
'` ^
|
||||
( # Real part
|
||||
[-+]?(\d+\.?\d*|\d*\.?\d+) # Real value (integer or float)
|
||||
([Ee][-+]?[0-2]?\d{1,3})? # Optional real exponent for scientific format
|
||||
)
|
||||
( # Imaginary part
|
||||
[-+]?(\d+\.?\d*|\d*\.?\d+) # Imaginary value (integer or float)
|
||||
([Ee][-+]?[0-2]?\d{1,3})? # Optional imaginary exponent for scientific format
|
||||
)?
|
||||
( # Imaginary part is optional
|
||||
([-+]?) # Imaginary (implicit 1 or -1) only
|
||||
([ij]?) # Imaginary i or j - depending on whether mathematical or engineering
|
||||
)
|
||||
$`uix';
|
||||
|
||||
/**
|
||||
* @var float $realPart The value of of this complex number on the real plane.
|
||||
*/
|
||||
protected $realPart = 0.0;
|
||||
|
||||
/**
|
||||
* @var float $imaginaryPart The value of of this complex number on the imaginary plane.
|
||||
*/
|
||||
protected $imaginaryPart = 0.0;
|
||||
|
||||
/**
|
||||
* @var string $suffix The suffix for this complex number (i or j).
|
||||
*/
|
||||
protected $suffix;
|
||||
|
||||
|
||||
/**
|
||||
* Validates whether the argument is a valid complex number, converting scalar or array values if possible
|
||||
*
|
||||
* @param mixed $complexNumber The value to parse
|
||||
* @return array
|
||||
* @throws Exception If the argument isn't a Complex number or cannot be converted to one
|
||||
*/
|
||||
private static function parseComplex($complexNumber)
|
||||
{
|
||||
// Test for real number, with no imaginary part
|
||||
if (is_numeric($complexNumber)) {
|
||||
return [$complexNumber, 0, null];
|
||||
}
|
||||
|
||||
// Fix silly human errors
|
||||
$complexNumber = str_replace(
|
||||
['+-', '-+', '++', '--'],
|
||||
['-', '-', '+', '+'],
|
||||
$complexNumber
|
||||
);
|
||||
|
||||
// Basic validation of string, to parse out real and imaginary parts, and any suffix
|
||||
$validComplex = preg_match(
|
||||
self::NUMBER_SPLIT_REGEXP,
|
||||
$complexNumber,
|
||||
$complexParts
|
||||
);
|
||||
|
||||
if (!$validComplex) {
|
||||
// Neither real nor imaginary part, so test to see if we actually have a suffix
|
||||
$validComplex = preg_match('/^([\-\+]?)([ij])$/ui', $complexNumber, $complexParts);
|
||||
if (!$validComplex) {
|
||||
throw new Exception('Invalid complex number');
|
||||
}
|
||||
// We have a suffix, so set the real to 0, the imaginary to either 1 or -1 (as defined by the sign)
|
||||
$imaginary = 1;
|
||||
if ($complexParts[1] === '-') {
|
||||
$imaginary = 0 - $imaginary;
|
||||
}
|
||||
return [0, $imaginary, $complexParts[2]];
|
||||
}
|
||||
|
||||
// If we don't have an imaginary part, identify whether it should be +1 or -1...
|
||||
if (($complexParts[4] === '') && ($complexParts[9] !== '')) {
|
||||
if ($complexParts[7] !== $complexParts[9]) {
|
||||
$complexParts[4] = 1;
|
||||
if ($complexParts[8] === '-') {
|
||||
$complexParts[4] = -1;
|
||||
}
|
||||
} else {
|
||||
// ... or if we have only the real and no imaginary part
|
||||
// (in which case our real should be the imaginary)
|
||||
$complexParts[4] = $complexParts[1];
|
||||
$complexParts[1] = 0;
|
||||
}
|
||||
}
|
||||
|
||||
// Return real and imaginary parts and suffix as an array, and set a default suffix if user input lazily
|
||||
return [
|
||||
$complexParts[1],
|
||||
$complexParts[4],
|
||||
!empty($complexParts[9]) ? $complexParts[9] : 'i'
|
||||
];
|
||||
}
|
||||
|
||||
|
||||
public function __construct($realPart = 0.0, $imaginaryPart = null, $suffix = 'i')
|
||||
{
|
||||
if ($imaginaryPart === null) {
|
||||
if (is_array($realPart)) {
|
||||
// We have an array of (potentially) real and imaginary parts, and any suffix
|
||||
list ($realPart, $imaginaryPart, $suffix) = array_values($realPart) + [0.0, 0.0, 'i'];
|
||||
} elseif ((is_string($realPart)) || (is_numeric($realPart))) {
|
||||
// We've been given a string to parse to extract the real and imaginary parts, and any suffix
|
||||
list($realPart, $imaginaryPart, $suffix) = self::parseComplex($realPart);
|
||||
}
|
||||
}
|
||||
|
||||
if ($imaginaryPart != 0.0 && empty($suffix)) {
|
||||
$suffix = 'i';
|
||||
} elseif ($imaginaryPart == 0.0 && !empty($suffix)) {
|
||||
$suffix = '';
|
||||
}
|
||||
|
||||
// Set parsed values in our properties
|
||||
$this->realPart = (float) $realPart;
|
||||
$this->imaginaryPart = (float) $imaginaryPart;
|
||||
$this->suffix = strtolower($suffix);
|
||||
}
|
||||
|
||||
/**
|
||||
* Gets the real part of this complex number
|
||||
*
|
||||
* @return Float
|
||||
*/
|
||||
public function getReal()
|
||||
{
|
||||
return $this->realPart;
|
||||
}
|
||||
|
||||
/**
|
||||
* Gets the imaginary part of this complex number
|
||||
*
|
||||
* @return Float
|
||||
*/
|
||||
public function getImaginary()
|
||||
{
|
||||
return $this->imaginaryPart;
|
||||
}
|
||||
|
||||
/**
|
||||
* Gets the suffix of this complex number
|
||||
*
|
||||
* @return String
|
||||
*/
|
||||
public function getSuffix()
|
||||
{
|
||||
return $this->suffix;
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns true if this is a real value, false if a complex value
|
||||
*
|
||||
* @return Bool
|
||||
*/
|
||||
public function isReal()
|
||||
{
|
||||
return $this->imaginaryPart == 0.0;
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns true if this is a complex value, false if a real value
|
||||
*
|
||||
* @return Bool
|
||||
*/
|
||||
public function isComplex()
|
||||
{
|
||||
return !$this->isReal();
|
||||
}
|
||||
|
||||
public function format()
|
||||
{
|
||||
$str = "";
|
||||
if ($this->imaginaryPart != 0.0) {
|
||||
if (\abs($this->imaginaryPart) != 1.0) {
|
||||
$str .= $this->imaginaryPart . $this->suffix;
|
||||
} else {
|
||||
$str .= (($this->imaginaryPart < 0.0) ? '-' : '') . $this->suffix;
|
||||
}
|
||||
}
|
||||
if ($this->realPart != 0.0) {
|
||||
if (($str) && ($this->imaginaryPart > 0.0)) {
|
||||
$str = "+" . $str;
|
||||
}
|
||||
$str = $this->realPart . $str;
|
||||
}
|
||||
if (!$str) {
|
||||
$str = "0.0";
|
||||
}
|
||||
|
||||
return $str;
|
||||
}
|
||||
|
||||
public function __toString()
|
||||
{
|
||||
return $this->format();
|
||||
}
|
||||
|
||||
/**
|
||||
* Validates whether the argument is a valid complex number, converting scalar or array values if possible
|
||||
*
|
||||
* @param mixed $complex The value to validate
|
||||
* @return Complex
|
||||
* @throws Exception If the argument isn't a Complex number or cannot be converted to one
|
||||
*/
|
||||
public static function validateComplexArgument($complex)
|
||||
{
|
||||
if (is_scalar($complex) || is_array($complex)) {
|
||||
$complex = new Complex($complex);
|
||||
} elseif (!is_object($complex) || !($complex instanceof Complex)) {
|
||||
throw new Exception('Value is not a valid complex number');
|
||||
}
|
||||
|
||||
return $complex;
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns the reverse of this complex number
|
||||
*
|
||||
* @return Complex
|
||||
*/
|
||||
public function reverse()
|
||||
{
|
||||
return new Complex(
|
||||
$this->imaginaryPart,
|
||||
$this->realPart,
|
||||
($this->realPart == 0.0) ? null : $this->suffix
|
||||
);
|
||||
}
|
||||
|
||||
public function invertImaginary()
|
||||
{
|
||||
return new Complex(
|
||||
$this->realPart,
|
||||
$this->imaginaryPart * -1,
|
||||
($this->imaginaryPart == 0.0) ? null : $this->suffix
|
||||
);
|
||||
}
|
||||
|
||||
public function invertReal()
|
||||
{
|
||||
return new Complex(
|
||||
$this->realPart * -1,
|
||||
$this->imaginaryPart,
|
||||
($this->imaginaryPart == 0.0) ? null : $this->suffix
|
||||
);
|
||||
}
|
||||
|
||||
protected static $functions = [
|
||||
'abs',
|
||||
'acos',
|
||||
'acosh',
|
||||
'acot',
|
||||
'acoth',
|
||||
'acsc',
|
||||
'acsch',
|
||||
'argument',
|
||||
'asec',
|
||||
'asech',
|
||||
'asin',
|
||||
'asinh',
|
||||
'atan',
|
||||
'atanh',
|
||||
'conjugate',
|
||||
'cos',
|
||||
'cosh',
|
||||
'cot',
|
||||
'coth',
|
||||
'csc',
|
||||
'csch',
|
||||
'exp',
|
||||
'inverse',
|
||||
'ln',
|
||||
'log2',
|
||||
'log10',
|
||||
'negative',
|
||||
'pow',
|
||||
'rho',
|
||||
'sec',
|
||||
'sech',
|
||||
'sin',
|
||||
'sinh',
|
||||
'sqrt',
|
||||
'tan',
|
||||
'tanh',
|
||||
'theta',
|
||||
];
|
||||
|
||||
protected static $operations = [
|
||||
'add',
|
||||
'subtract',
|
||||
'multiply',
|
||||
'divideby',
|
||||
'divideinto',
|
||||
];
|
||||
|
||||
/**
|
||||
* Returns the result of the function call or operation
|
||||
*
|
||||
* @return Complex|float
|
||||
* @throws Exception|\InvalidArgumentException
|
||||
*/
|
||||
public function __call($functionName, $arguments)
|
||||
{
|
||||
$functionName = strtolower(str_replace('_', '', $functionName));
|
||||
|
||||
// Test for function calls
|
||||
if (in_array($functionName, self::$functions, true)) {
|
||||
$functionName = "\\" . __NAMESPACE__ . "\\{$functionName}";
|
||||
return $functionName($this, ...$arguments);
|
||||
}
|
||||
// Test for operation calls
|
||||
if (in_array($functionName, self::$operations, true)) {
|
||||
$functionName = "\\" . __NAMESPACE__ . "\\{$functionName}";
|
||||
return $functionName($this, ...$arguments);
|
||||
}
|
||||
throw new Exception('Function or Operation does not exist');
|
||||
}
|
||||
}
|
@ -0,0 +1,13 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
* Exception.
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
class Exception extends \Exception
|
||||
{
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex abs() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the absolute value (modulus) of a complex number.
|
||||
* Also known as the rho of the complex number, i.e. the distance/radius
|
||||
* from the centrepoint to the representation of the number in polar coordinates.
|
||||
*
|
||||
* This function is a synonym for rho()
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return float The absolute (or rho) value of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*
|
||||
* @see rho
|
||||
*
|
||||
*/
|
||||
function abs($complex)
|
||||
{
|
||||
return rho($complex);
|
||||
}
|
@ -0,0 +1,38 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex acos() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse cosine of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse cosine of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function acos($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
$square = clone $complex;
|
||||
$square = multiply($square, $complex);
|
||||
$invsqrt = new Complex(1.0);
|
||||
$invsqrt = subtract($invsqrt, $square);
|
||||
$invsqrt = sqrt($invsqrt);
|
||||
$adjust = new Complex(
|
||||
$complex->getReal() - $invsqrt->getImaginary(),
|
||||
$complex->getImaginary() + $invsqrt->getReal()
|
||||
);
|
||||
$log = ln($adjust);
|
||||
|
||||
return new Complex(
|
||||
$log->getImaginary(),
|
||||
-1 * $log->getReal()
|
||||
);
|
||||
}
|
@ -0,0 +1,34 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex acosh() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse hyperbolic cosine of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse hyperbolic cosine of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function acosh($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->isReal() && ($complex->getReal() > 1)) {
|
||||
return new Complex(\acosh($complex->getReal()));
|
||||
}
|
||||
|
||||
$acosh = acos($complex)
|
||||
->reverse();
|
||||
if ($acosh->getReal() < 0.0) {
|
||||
$acosh = $acosh->invertReal();
|
||||
}
|
||||
|
||||
return $acosh;
|
||||
}
|
@ -0,0 +1,25 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex acot() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse cotangent of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse cotangent of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function acot($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
return atan(inverse($complex));
|
||||
}
|
@ -0,0 +1,25 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex acoth() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse hyperbolic cotangent of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse hyperbolic cotangent of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function acoth($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
return atanh(inverse($complex));
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex acsc() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse cosecant of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse cosecant of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function acsc($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->getReal() == 0.0 && $complex->getImaginary() == 0.0) {
|
||||
return INF;
|
||||
}
|
||||
|
||||
return asin(inverse($complex));
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex acsch() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse hyperbolic cosecant of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse hyperbolic cosecant of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function acsch($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->getReal() == 0.0 && $complex->getImaginary() == 0.0) {
|
||||
return INF;
|
||||
}
|
||||
|
||||
return asinh(inverse($complex));
|
||||
}
|
@ -0,0 +1,28 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex argument() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the argument of a complex number.
|
||||
* Also known as the theta of the complex number, i.e. the angle in radians
|
||||
* from the real axis to the representation of the number in polar coordinates.
|
||||
*
|
||||
* This function is a synonym for theta()
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return float The argument (or theta) value of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*
|
||||
* @see theta
|
||||
*/
|
||||
function argument($complex)
|
||||
{
|
||||
return theta($complex);
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex asec() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse secant of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse secant of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function asec($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->getReal() == 0.0 && $complex->getImaginary() == 0.0) {
|
||||
return INF;
|
||||
}
|
||||
|
||||
return acos(inverse($complex));
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex asech() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse hyperbolic secant of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse hyperbolic secant of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function asech($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->getReal() == 0.0 && $complex->getImaginary() == 0.0) {
|
||||
return INF;
|
||||
}
|
||||
|
||||
return acosh(inverse($complex));
|
||||
}
|
@ -0,0 +1,37 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex asin() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse sine of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse sine of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function asin($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
$square = multiply($complex, $complex);
|
||||
$invsqrt = new Complex(1.0);
|
||||
$invsqrt = subtract($invsqrt, $square);
|
||||
$invsqrt = sqrt($invsqrt);
|
||||
$adjust = new Complex(
|
||||
$invsqrt->getReal() - $complex->getImaginary(),
|
||||
$invsqrt->getImaginary() + $complex->getReal()
|
||||
);
|
||||
$log = ln($adjust);
|
||||
|
||||
return new Complex(
|
||||
$log->getImaginary(),
|
||||
-1 * $log->getReal()
|
||||
);
|
||||
}
|
@ -0,0 +1,33 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex asinh() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse hyperbolic sine of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse hyperbolic sine of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function asinh($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->isReal() && ($complex->getReal() > 1)) {
|
||||
return new Complex(\asinh($complex->getReal()));
|
||||
}
|
||||
|
||||
$asinh = clone $complex;
|
||||
$asinh = $asinh->reverse()
|
||||
->invertReal();
|
||||
$asinh = asin($asinh);
|
||||
return $asinh->reverse()
|
||||
->invertImaginary();
|
||||
}
|
@ -0,0 +1,45 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex atan() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
//include_once 'Math/Complex.php';
|
||||
//include_once 'Math/ComplexOp.php';
|
||||
|
||||
/**
|
||||
* Returns the inverse tangent of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse tangent of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function atan($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->isReal()) {
|
||||
return new Complex(\atan($complex->getReal()));
|
||||
}
|
||||
|
||||
$t1Value = new Complex(-1 * $complex->getImaginary(), $complex->getReal());
|
||||
$uValue = new Complex(1, 0);
|
||||
|
||||
$d1Value = clone $uValue;
|
||||
$d1Value = subtract($d1Value, $t1Value);
|
||||
$d2Value = add($t1Value, $uValue);
|
||||
$uResult = $d1Value->divideBy($d2Value);
|
||||
$uResult = ln($uResult);
|
||||
|
||||
return new Complex(
|
||||
(($uResult->getImaginary() == M_PI) ? -M_PI : $uResult->getImaginary()) * -0.5,
|
||||
$uResult->getReal() * 0.5,
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,38 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex atanh() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse hyperbolic tangent of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse hyperbolic tangent of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function atanh($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->isReal()) {
|
||||
$real = $complex->getReal();
|
||||
if ($real >= -1.0 && $real <= 1.0) {
|
||||
return new Complex(\atanh($real));
|
||||
} else {
|
||||
return new Complex(\atanh(1 / $real), (($real < 0.0) ? M_PI_2 : -1 * M_PI_2));
|
||||
}
|
||||
}
|
||||
|
||||
$iComplex = clone $complex;
|
||||
$iComplex = $iComplex->invertImaginary()
|
||||
->reverse();
|
||||
return atan($iComplex)
|
||||
->invertReal()
|
||||
->reverse();
|
||||
}
|
@ -0,0 +1,28 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex conjugate() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the complex conjugate of a complex number
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The conjugate of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function conjugate($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
return new Complex(
|
||||
$complex->getReal(),
|
||||
-1 * $complex->getImaginary(),
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,34 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex cos() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the cosine of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The cosine of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function cos($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->isReal()) {
|
||||
return new Complex(\cos($complex->getReal()));
|
||||
}
|
||||
|
||||
return conjugate(
|
||||
new Complex(
|
||||
\cos($complex->getReal()) * \cosh($complex->getImaginary()),
|
||||
\sin($complex->getReal()) * \sinh($complex->getImaginary()),
|
||||
$complex->getSuffix()
|
||||
)
|
||||
);
|
||||
}
|
@ -0,0 +1,32 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex cosh() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the hyperbolic cosine of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The hyperbolic cosine of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function cosh($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->isReal()) {
|
||||
return new Complex(\cosh($complex->getReal()));
|
||||
}
|
||||
|
||||
return new Complex(
|
||||
\cosh($complex->getReal()) * \cos($complex->getImaginary()),
|
||||
\sinh($complex->getReal()) * \sin($complex->getImaginary()),
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex cot() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the cotangent of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The cotangent of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function cot($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->getReal() == 0.0 && $complex->getImaginary() == 0.0) {
|
||||
return new Complex(INF);
|
||||
}
|
||||
|
||||
return inverse(tan($complex));
|
||||
}
|
@ -0,0 +1,24 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex coth() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the hyperbolic cotangent of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The hyperbolic cotangent of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function coth($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
return inverse(tanh($complex));
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex csc() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the cosecant of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The cosecant of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function csc($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->getReal() == 0.0 && $complex->getImaginary() == 0.0) {
|
||||
return INF;
|
||||
}
|
||||
|
||||
return inverse(sin($complex));
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex csch() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the hyperbolic cosecant of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The hyperbolic cosecant of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function csch($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->getReal() == 0.0 && $complex->getImaginary() == 0.0) {
|
||||
return INF;
|
||||
}
|
||||
|
||||
return inverse(sinh($complex));
|
||||
}
|
@ -0,0 +1,34 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex exp() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the exponential of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The exponential of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function exp($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if (($complex->getReal() == 0.0) && (\abs($complex->getImaginary()) == M_PI)) {
|
||||
return new Complex(-1.0, 0.0);
|
||||
}
|
||||
|
||||
$rho = \exp($complex->getReal());
|
||||
|
||||
return new Complex(
|
||||
$rho * \cos($complex->getImaginary()),
|
||||
$rho * \sin($complex->getImaginary()),
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex inverse() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the inverse of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The inverse of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function inverse($complex)
|
||||
{
|
||||
$complex = clone Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->getReal() == 0.0 && $complex->getImaginary() == 0.0) {
|
||||
throw new \InvalidArgumentException('Division by zero');
|
||||
}
|
||||
|
||||
return $complex->divideInto(1.0);
|
||||
}
|
@ -0,0 +1,33 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex ln() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the natural logarithm of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The natural logarithm of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If the real and the imaginary parts are both zero
|
||||
*/
|
||||
function ln($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if (($complex->getReal() == 0.0) && ($complex->getImaginary() == 0.0)) {
|
||||
throw new \InvalidArgumentException();
|
||||
}
|
||||
|
||||
return new Complex(
|
||||
\log(rho($complex)),
|
||||
theta($complex),
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,32 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex log10() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the common logarithm (base 10) of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The common logarithm (base 10) of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If the real and the imaginary parts are both zero
|
||||
*/
|
||||
function log10($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if (($complex->getReal() == 0.0) && ($complex->getImaginary() == 0.0)) {
|
||||
throw new \InvalidArgumentException();
|
||||
} elseif (($complex->getReal() > 0.0) && ($complex->getImaginary() == 0.0)) {
|
||||
return new Complex(\log10($complex->getReal()), 0.0, $complex->getSuffix());
|
||||
}
|
||||
|
||||
return ln($complex)
|
||||
->multiply(\log10(Complex::EULER));
|
||||
}
|
@ -0,0 +1,32 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex log2() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the base-2 logarithm of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The base-2 logarithm of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If the real and the imaginary parts are both zero
|
||||
*/
|
||||
function log2($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if (($complex->getReal() == 0.0) && ($complex->getImaginary() == 0.0)) {
|
||||
throw new \InvalidArgumentException();
|
||||
} elseif (($complex->getReal() > 0.0) && ($complex->getImaginary() == 0.0)) {
|
||||
return new Complex(\log($complex->getReal(), 2), 0.0, $complex->getSuffix());
|
||||
}
|
||||
|
||||
return ln($complex)
|
||||
->multiply(\log(Complex::EULER, 2));
|
||||
}
|
@ -0,0 +1,31 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex negative() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the negative of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return float The negative value of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*
|
||||
* @see rho
|
||||
*
|
||||
*/
|
||||
function negative($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
return new Complex(
|
||||
-1 * $complex->getReal(),
|
||||
-1 * $complex->getImaginary(),
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,40 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex pow() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns a complex number raised to a power.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @param float|integer $power The power to raise this value to
|
||||
* @return Complex The complex argument raised to the real power.
|
||||
* @throws Exception If the power argument isn't a valid real
|
||||
*/
|
||||
function pow($complex, $power)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if (!is_numeric($power)) {
|
||||
throw new Exception('Power argument must be a real number');
|
||||
}
|
||||
|
||||
if ($complex->getImaginary() == 0.0 && $complex->getReal() >= 0.0) {
|
||||
return new Complex(\pow($complex->getReal(), $power));
|
||||
}
|
||||
|
||||
$rValue = \sqrt(($complex->getReal() * $complex->getReal()) + ($complex->getImaginary() * $complex->getImaginary()));
|
||||
$rPower = \pow($rValue, $power);
|
||||
$theta = $complex->argument() * $power;
|
||||
if ($theta == 0) {
|
||||
return new Complex(1);
|
||||
}
|
||||
|
||||
return new Complex($rPower * \cos($theta), $rPower * \sin($theta), $complex->getSuffix());
|
||||
}
|
@ -0,0 +1,28 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex rho() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the rho of a complex number.
|
||||
* This is the distance/radius from the centrepoint to the representation of the number in polar coordinates.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return float The rho value of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function rho($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
return \sqrt(
|
||||
($complex->getReal() * $complex->getReal()) +
|
||||
($complex->getImaginary() * $complex->getImaginary())
|
||||
);
|
||||
}
|
@ -0,0 +1,25 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex sec() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the secant of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The secant of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function sec($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
return inverse(cos($complex));
|
||||
}
|
@ -0,0 +1,25 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex sech() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the hyperbolic secant of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The hyperbolic secant of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function sech($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
return inverse(cosh($complex));
|
||||
}
|
@ -0,0 +1,32 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex sin() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the sine of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The sine of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function sin($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->isReal()) {
|
||||
return new Complex(\sin($complex->getReal()));
|
||||
}
|
||||
|
||||
return new Complex(
|
||||
\sin($complex->getReal()) * \cosh($complex->getImaginary()),
|
||||
\cos($complex->getReal()) * \sinh($complex->getImaginary()),
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,32 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex sinh() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the hyperbolic sine of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The hyperbolic sine of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function sinh($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->isReal()) {
|
||||
return new Complex(\sinh($complex->getReal()));
|
||||
}
|
||||
|
||||
return new Complex(
|
||||
\sinh($complex->getReal()) * \cos($complex->getImaginary()),
|
||||
\cosh($complex->getReal()) * \sin($complex->getImaginary()),
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,29 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex sqrt() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the square root of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The Square root of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function sqrt($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
$theta = theta($complex);
|
||||
$delta1 = \cos($theta / 2);
|
||||
$delta2 = \sin($theta / 2);
|
||||
$rho = \sqrt(rho($complex));
|
||||
|
||||
return new Complex($delta1 * $rho, $delta2 * $rho, $complex->getSuffix());
|
||||
}
|
@ -0,0 +1,40 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex tan() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the tangent of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The tangent of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function tan($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->isReal()) {
|
||||
return new Complex(\tan($complex->getReal()));
|
||||
}
|
||||
|
||||
$real = $complex->getReal();
|
||||
$imaginary = $complex->getImaginary();
|
||||
$divisor = 1 + \pow(\tan($real), 2) * \pow(\tanh($imaginary), 2);
|
||||
if ($divisor == 0.0) {
|
||||
throw new \InvalidArgumentException('Division by zero');
|
||||
}
|
||||
|
||||
return new Complex(
|
||||
\pow(sech($imaginary)->getReal(), 2) * \tan($real) / $divisor,
|
||||
\pow(sec($real)->getReal(), 2) * \tanh($imaginary) / $divisor,
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,35 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex tanh() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the hyperbolic tangent of a complex number.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return Complex The hyperbolic tangent of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
* @throws \InvalidArgumentException If function would result in a division by zero
|
||||
*/
|
||||
function tanh($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
$real = $complex->getReal();
|
||||
$imaginary = $complex->getImaginary();
|
||||
$divisor = \cos($imaginary) * \cos($imaginary) + \sinh($real) * \sinh($real);
|
||||
if ($divisor == 0.0) {
|
||||
throw new \InvalidArgumentException('Division by zero');
|
||||
}
|
||||
|
||||
return new Complex(
|
||||
\sinh($real) * \cosh($real) / $divisor,
|
||||
0.5 * \sin(2 * $imaginary) / $divisor,
|
||||
$complex->getSuffix()
|
||||
);
|
||||
}
|
@ -0,0 +1,38 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex theta() function
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Returns the theta of a complex number.
|
||||
* This is the angle in radians from the real axis to the representation of the number in polar coordinates.
|
||||
*
|
||||
* @param Complex|mixed $complex Complex number or a numeric value.
|
||||
* @return float The theta value of the complex argument.
|
||||
* @throws Exception If argument isn't a valid real or complex number.
|
||||
*/
|
||||
function theta($complex)
|
||||
{
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($complex->getReal() == 0.0) {
|
||||
if ($complex->isReal()) {
|
||||
return 0.0;
|
||||
} elseif ($complex->getImaginary() < 0.0) {
|
||||
return M_PI / -2;
|
||||
}
|
||||
return M_PI / 2;
|
||||
} elseif ($complex->getReal() > 0.0) {
|
||||
return \atan($complex->getImaginary() / $complex->getReal());
|
||||
} elseif ($complex->getImaginary() < 0.0) {
|
||||
return -(M_PI - \atan(\abs($complex->getImaginary()) / \abs($complex->getReal())));
|
||||
}
|
||||
|
||||
return M_PI - \atan($complex->getImaginary() / \abs($complex->getReal()));
|
||||
}
|
@ -0,0 +1,46 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex addition operation
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Adds two or more complex numbers
|
||||
*
|
||||
* @param array of string|integer|float|Complex $complexValues The numbers to add
|
||||
* @return Complex
|
||||
*/
|
||||
function add(...$complexValues)
|
||||
{
|
||||
if (count($complexValues) < 2) {
|
||||
throw new \Exception('This function requires at least 2 arguments');
|
||||
}
|
||||
|
||||
$base = array_shift($complexValues);
|
||||
$result = clone Complex::validateComplexArgument($base);
|
||||
|
||||
foreach ($complexValues as $complex) {
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($result->isComplex() && $complex->isComplex() &&
|
||||
$result->getSuffix() !== $complex->getSuffix()) {
|
||||
throw new Exception('Suffix Mismatch');
|
||||
}
|
||||
|
||||
$real = $result->getReal() + $complex->getReal();
|
||||
$imaginary = $result->getImaginary() + $complex->getImaginary();
|
||||
|
||||
$result = new Complex(
|
||||
$real,
|
||||
$imaginary,
|
||||
($imaginary == 0.0) ? null : max($result->getSuffix(), $complex->getSuffix())
|
||||
);
|
||||
}
|
||||
|
||||
return $result;
|
||||
}
|
@ -0,0 +1,56 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex division operation
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Divides two or more complex numbers
|
||||
*
|
||||
* @param array of string|integer|float|Complex $complexValues The numbers to divide
|
||||
* @return Complex
|
||||
*/
|
||||
function divideby(...$complexValues)
|
||||
{
|
||||
if (count($complexValues) < 2) {
|
||||
throw new \Exception('This function requires at least 2 arguments');
|
||||
}
|
||||
|
||||
$base = array_shift($complexValues);
|
||||
$result = clone Complex::validateComplexArgument($base);
|
||||
|
||||
foreach ($complexValues as $complex) {
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($result->isComplex() && $complex->isComplex() &&
|
||||
$result->getSuffix() !== $complex->getSuffix()) {
|
||||
throw new Exception('Suffix Mismatch');
|
||||
}
|
||||
if ($complex->getReal() == 0.0 && $complex->getImaginary() == 0.0) {
|
||||
throw new \InvalidArgumentException('Division by zero');
|
||||
}
|
||||
|
||||
$delta1 = ($result->getReal() * $complex->getReal()) +
|
||||
($result->getImaginary() * $complex->getImaginary());
|
||||
$delta2 = ($result->getImaginary() * $complex->getReal()) -
|
||||
($result->getReal() * $complex->getImaginary());
|
||||
$delta3 = ($complex->getReal() * $complex->getReal()) +
|
||||
($complex->getImaginary() * $complex->getImaginary());
|
||||
|
||||
$real = $delta1 / $delta3;
|
||||
$imaginary = $delta2 / $delta3;
|
||||
|
||||
$result = new Complex(
|
||||
$real,
|
||||
$imaginary,
|
||||
($imaginary == 0.0) ? null : max($result->getSuffix(), $complex->getSuffix())
|
||||
);
|
||||
}
|
||||
|
||||
return $result;
|
||||
}
|
@ -0,0 +1,56 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex division operation
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Divides two or more complex numbers
|
||||
*
|
||||
* @param array of string|integer|float|Complex $complexValues The numbers to divide
|
||||
* @return Complex
|
||||
*/
|
||||
function divideinto(...$complexValues)
|
||||
{
|
||||
if (count($complexValues) < 2) {
|
||||
throw new \Exception('This function requires at least 2 arguments');
|
||||
}
|
||||
|
||||
$base = array_shift($complexValues);
|
||||
$result = clone Complex::validateComplexArgument($base);
|
||||
|
||||
foreach ($complexValues as $complex) {
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($result->isComplex() && $complex->isComplex() &&
|
||||
$result->getSuffix() !== $complex->getSuffix()) {
|
||||
throw new Exception('Suffix Mismatch');
|
||||
}
|
||||
if ($result->getReal() == 0.0 && $result->getImaginary() == 0.0) {
|
||||
throw new \InvalidArgumentException('Division by zero');
|
||||
}
|
||||
|
||||
$delta1 = ($complex->getReal() * $result->getReal()) +
|
||||
($complex->getImaginary() * $result->getImaginary());
|
||||
$delta2 = ($complex->getImaginary() * $result->getReal()) -
|
||||
($complex->getReal() * $result->getImaginary());
|
||||
$delta3 = ($result->getReal() * $result->getReal()) +
|
||||
($result->getImaginary() * $result->getImaginary());
|
||||
|
||||
$real = $delta1 / $delta3;
|
||||
$imaginary = $delta2 / $delta3;
|
||||
|
||||
$result = new Complex(
|
||||
$real,
|
||||
$imaginary,
|
||||
($imaginary == 0.0) ? null : max($result->getSuffix(), $complex->getSuffix())
|
||||
);
|
||||
}
|
||||
|
||||
return $result;
|
||||
}
|
@ -0,0 +1,48 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex multiplication operation
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Multiplies two or more complex numbers
|
||||
*
|
||||
* @param array of string|integer|float|Complex $complexValues The numbers to multiply
|
||||
* @return Complex
|
||||
*/
|
||||
function multiply(...$complexValues)
|
||||
{
|
||||
if (count($complexValues) < 2) {
|
||||
throw new \Exception('This function requires at least 2 arguments');
|
||||
}
|
||||
|
||||
$base = array_shift($complexValues);
|
||||
$result = clone Complex::validateComplexArgument($base);
|
||||
|
||||
foreach ($complexValues as $complex) {
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($result->isComplex() && $complex->isComplex() &&
|
||||
$result->getSuffix() !== $complex->getSuffix()) {
|
||||
throw new Exception('Suffix Mismatch');
|
||||
}
|
||||
|
||||
$real = ($result->getReal() * $complex->getReal()) -
|
||||
($result->getImaginary() * $complex->getImaginary());
|
||||
$imaginary = ($result->getReal() * $complex->getImaginary()) +
|
||||
($result->getImaginary() * $complex->getReal());
|
||||
|
||||
$result = new Complex(
|
||||
$real,
|
||||
$imaginary,
|
||||
($imaginary == 0.0) ? null : max($result->getSuffix(), $complex->getSuffix())
|
||||
);
|
||||
}
|
||||
|
||||
return $result;
|
||||
}
|
@ -0,0 +1,46 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Function code for the complex subtraction operation
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPComplex)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Complex;
|
||||
|
||||
/**
|
||||
* Subtracts two or more complex numbers
|
||||
*
|
||||
* @param array of string|integer|float|Complex $complexValues The numbers to subtract
|
||||
* @return Complex
|
||||
*/
|
||||
function subtract(...$complexValues)
|
||||
{
|
||||
if (count($complexValues) < 2) {
|
||||
throw new \Exception('This function requires at least 2 arguments');
|
||||
}
|
||||
|
||||
$base = array_shift($complexValues);
|
||||
$result = clone Complex::validateComplexArgument($base);
|
||||
|
||||
foreach ($complexValues as $complex) {
|
||||
$complex = Complex::validateComplexArgument($complex);
|
||||
|
||||
if ($result->isComplex() && $complex->isComplex() &&
|
||||
$result->getSuffix() !== $complex->getSuffix()) {
|
||||
throw new Exception('Suffix Mismatch');
|
||||
}
|
||||
|
||||
$real = $result->getReal() - $complex->getReal();
|
||||
$imaginary = $result->getImaginary() - $complex->getImaginary();
|
||||
|
||||
$result = new Complex(
|
||||
$real,
|
||||
$imaginary,
|
||||
($imaginary == 0.0) ? null : max($result->getSuffix(), $complex->getSuffix())
|
||||
);
|
||||
}
|
||||
|
||||
return $result;
|
||||
}
|
@ -0,0 +1,94 @@
|
||||
{
|
||||
"name": "markbaker/complex",
|
||||
"type": "library",
|
||||
"description": "PHP Class for working with complex numbers",
|
||||
"keywords": ["complex", "mathematics"],
|
||||
"homepage": "https://github.com/MarkBaker/PHPComplex",
|
||||
"license": "MIT",
|
||||
"authors": [
|
||||
{
|
||||
"name": "Mark Baker",
|
||||
"email": "mark@lange.demon.co.uk"
|
||||
}
|
||||
],
|
||||
"require": {
|
||||
"php": "^5.6.0|^7.0"
|
||||
},
|
||||
"require-dev": {
|
||||
"phpunit/phpunit": "^4.8.35|^5.0|^6.0|^7.0",
|
||||
"phpdocumentor/phpdocumentor":"2.*",
|
||||
"phpmd/phpmd": "2.*",
|
||||
"sebastian/phpcpd": "2.*",
|
||||
"phploc/phploc": "^4.0|^5.0|^6.0|^7.0",
|
||||
"squizlabs/php_codesniffer": "^3.4.0",
|
||||
"phpcompatibility/php-compatibility": "^9.0",
|
||||
"dealerdirect/phpcodesniffer-composer-installer": "^0.5.0"
|
||||
},
|
||||
"autoload": {
|
||||
"psr-4": {
|
||||
"Complex\\": "classes/src/"
|
||||
},
|
||||
"files": [
|
||||
"classes/src/functions/abs.php",
|
||||
"classes/src/functions/acos.php",
|
||||
"classes/src/functions/acosh.php",
|
||||
"classes/src/functions/acot.php",
|
||||
"classes/src/functions/acoth.php",
|
||||
"classes/src/functions/acsc.php",
|
||||
"classes/src/functions/acsch.php",
|
||||
"classes/src/functions/argument.php",
|
||||
"classes/src/functions/asec.php",
|
||||
"classes/src/functions/asech.php",
|
||||
"classes/src/functions/asin.php",
|
||||
"classes/src/functions/asinh.php",
|
||||
"classes/src/functions/atan.php",
|
||||
"classes/src/functions/atanh.php",
|
||||
"classes/src/functions/conjugate.php",
|
||||
"classes/src/functions/cos.php",
|
||||
"classes/src/functions/cosh.php",
|
||||
"classes/src/functions/cot.php",
|
||||
"classes/src/functions/coth.php",
|
||||
"classes/src/functions/csc.php",
|
||||
"classes/src/functions/csch.php",
|
||||
"classes/src/functions/exp.php",
|
||||
"classes/src/functions/inverse.php",
|
||||
"classes/src/functions/ln.php",
|
||||
"classes/src/functions/log2.php",
|
||||
"classes/src/functions/log10.php",
|
||||
"classes/src/functions/negative.php",
|
||||
"classes/src/functions/pow.php",
|
||||
"classes/src/functions/rho.php",
|
||||
"classes/src/functions/sec.php",
|
||||
"classes/src/functions/sech.php",
|
||||
"classes/src/functions/sin.php",
|
||||
"classes/src/functions/sinh.php",
|
||||
"classes/src/functions/sqrt.php",
|
||||
"classes/src/functions/tan.php",
|
||||
"classes/src/functions/tanh.php",
|
||||
"classes/src/functions/theta.php",
|
||||
"classes/src/operations/add.php",
|
||||
"classes/src/operations/subtract.php",
|
||||
"classes/src/operations/multiply.php",
|
||||
"classes/src/operations/divideby.php",
|
||||
"classes/src/operations/divideinto.php"
|
||||
]
|
||||
},
|
||||
"scripts": {
|
||||
"style": [
|
||||
"phpcs --report-width=200 --report=summary,full -n"
|
||||
],
|
||||
"mess": [
|
||||
"phpmd classes/src/ xml codesize,unusedcode,design,naming -n"
|
||||
],
|
||||
"lines": [
|
||||
"phploc classes/src/ -n"
|
||||
],
|
||||
"cpd": [
|
||||
"phpcpd classes/src/ -n"
|
||||
],
|
||||
"versions": [
|
||||
"phpcs --report-width=200 --report=summary,full classes/src/ --standard=PHPCompatibility --runtime-set testVersion 5.6- -n"
|
||||
]
|
||||
},
|
||||
"minimum-stability": "dev"
|
||||
}
|
@ -0,0 +1,154 @@
|
||||
<?php
|
||||
|
||||
use Complex\Complex as Complex;
|
||||
|
||||
include('../classes/Bootstrap.php');
|
||||
|
||||
echo 'Create', PHP_EOL;
|
||||
|
||||
$x = new Complex(123);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123, 456);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(array(123,456,'j'));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex('1.23e-4--2.34e-5i');
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
|
||||
echo PHP_EOL, 'Add', PHP_EOL;
|
||||
|
||||
$x = new Complex(123);
|
||||
$x->add(456);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456);
|
||||
$x->add(789.012);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->add(new Complex(-987.654, -32.1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->add(-987.654);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->add(new Complex(0, 1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->add(new Complex(0, -1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
|
||||
echo PHP_EOL, 'Subtract', PHP_EOL;
|
||||
|
||||
$x = new Complex(123);
|
||||
$x->subtract(456);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456);
|
||||
$x->subtract(789.012);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->subtract(new Complex(-987.654, -32.1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->subtract(-987.654);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->subtract(new Complex(0, 1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->subtract(new Complex(0, -1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
|
||||
echo PHP_EOL, 'Multiply', PHP_EOL;
|
||||
|
||||
$x = new Complex(123);
|
||||
$x->multiply(456);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456);
|
||||
$x->multiply(789.012);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->multiply(new Complex(-987.654, -32.1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->multiply(-987.654);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->multiply(new Complex(0, 1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->multiply(new Complex(0, -1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
|
||||
echo PHP_EOL, 'Divide By', PHP_EOL;
|
||||
|
||||
$x = new Complex(123);
|
||||
$x->divideBy(456);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456);
|
||||
$x->divideBy(789.012);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->divideBy(new Complex(-987.654, -32.1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->divideBy(-987.654);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->divideBy(new Complex(0, 1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->divideBy(new Complex(0, -1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
|
||||
echo PHP_EOL, 'Divide Into', PHP_EOL;
|
||||
|
||||
$x = new Complex(123);
|
||||
$x->divideInto(456);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456);
|
||||
$x->divideInto(789.012);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->divideInto(new Complex(-987.654, -32.1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(123.456, 78.90);
|
||||
$x->divideInto(-987.654);
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->divideInto(new Complex(0, 1));
|
||||
echo $x, PHP_EOL;
|
||||
|
||||
$x = new Complex(-987.654, -32.1);
|
||||
$x->divideInto(new Complex(0, -1));
|
||||
echo $x, PHP_EOL;
|
@ -0,0 +1,52 @@
|
||||
<?php
|
||||
|
||||
namespace Complex;
|
||||
|
||||
include('../classes/Bootstrap.php');
|
||||
|
||||
echo 'Function Examples', PHP_EOL;
|
||||
|
||||
$functions = array(
|
||||
'abs',
|
||||
'acos',
|
||||
'acosh',
|
||||
'acsc',
|
||||
'acsch',
|
||||
'argument',
|
||||
'asec',
|
||||
'asech',
|
||||
'asin',
|
||||
'asinh',
|
||||
'conjugate',
|
||||
'cos',
|
||||
'cosh',
|
||||
'csc',
|
||||
'csch',
|
||||
'exp',
|
||||
'inverse',
|
||||
'ln',
|
||||
'log2',
|
||||
'log10',
|
||||
'rho',
|
||||
'sec',
|
||||
'sech',
|
||||
'sin',
|
||||
'sinh',
|
||||
'sqrt',
|
||||
'theta'
|
||||
);
|
||||
|
||||
for ($real = -3.5; $real <= 3.5; $real += 0.5) {
|
||||
for ($imaginary = -3.5; $imaginary <= 3.5; $imaginary += 0.5) {
|
||||
foreach ($functions as $function) {
|
||||
$complexFunction = __NAMESPACE__ . '\\' . $function;
|
||||
$complex = new Complex($real, $imaginary);
|
||||
try {
|
||||
echo $function, '(', $complex, ') = ', $complexFunction($complex), PHP_EOL;
|
||||
} catch (\Exception $e) {
|
||||
echo $function, '(', $complex, ') ERROR: ', $e->getMessage(), PHP_EOL;
|
||||
}
|
||||
}
|
||||
echo PHP_EOL;
|
||||
}
|
||||
}
|
@ -0,0 +1,34 @@
|
||||
<?php
|
||||
|
||||
use Complex\Complex as Complex;
|
||||
|
||||
include('../classes/Bootstrap.php');
|
||||
|
||||
$values = [
|
||||
new Complex(123),
|
||||
new Complex(456, 123),
|
||||
new Complex(0.0, 456),
|
||||
];
|
||||
|
||||
foreach ($values as $value) {
|
||||
echo $value, PHP_EOL;
|
||||
}
|
||||
|
||||
echo 'Addition', PHP_EOL;
|
||||
|
||||
$result = \Complex\add(...$values);
|
||||
echo '=> ', $result, PHP_EOL;
|
||||
|
||||
echo PHP_EOL;
|
||||
|
||||
echo 'Subtraction', PHP_EOL;
|
||||
|
||||
$result = \Complex\subtract(...$values);
|
||||
echo '=> ', $result, PHP_EOL;
|
||||
|
||||
echo PHP_EOL;
|
||||
|
||||
echo 'Multiplication', PHP_EOL;
|
||||
|
||||
$result = \Complex\multiply(...$values);
|
||||
echo '=> ', $result, PHP_EOL;
|
@ -0,0 +1,25 @@
|
||||
The MIT License (MIT)
|
||||
=====================
|
||||
|
||||
Copyright © `2017` `Mark Baker`
|
||||
|
||||
Permission is hereby granted, free of charge, to any person
|
||||
obtaining a copy of this software and associated documentation
|
||||
files (the “Software”), to deal in the Software without
|
||||
restriction, including without limitation the rights to use,
|
||||
copy, modify, merge, publish, distribute, sublicense, and/or sell
|
||||
copies of the Software, and to permit persons to whom the
|
||||
Software is furnished to do so, subject to the following
|
||||
conditions:
|
||||
|
||||
The above copyright notice and this permission notice shall be
|
||||
included in all copies or substantial portions of the Software.
|
||||
|
||||
THE SOFTWARE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND,
|
||||
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES
|
||||
OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
||||
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT
|
||||
HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY,
|
||||
WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING
|
||||
FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR
|
||||
OTHER DEALINGS IN THE SOFTWARE.
|
@ -0,0 +1,112 @@
|
||||
name: main
|
||||
on: [ push, pull_request ]
|
||||
jobs:
|
||||
test:
|
||||
runs-on: ubuntu-latest
|
||||
strategy:
|
||||
matrix:
|
||||
php-version:
|
||||
- '5.6'
|
||||
- '7.0'
|
||||
- '7.1'
|
||||
- '7.2'
|
||||
- '7.3'
|
||||
- '7.4'
|
||||
|
||||
name: PHP ${{ matrix.php-version }}
|
||||
|
||||
steps:
|
||||
- name: Checkout
|
||||
uses: actions/checkout@v2
|
||||
|
||||
- name: Setup PHP, with composer and extensions
|
||||
uses: shivammathur/setup-php@v2
|
||||
with:
|
||||
php-version: ${{ matrix.php-version }}
|
||||
extensions: ctype, dom, gd, iconv, fileinfo, libxml, mbstring, simplexml, xml, xmlreader, xmlwriter, zip, zlib
|
||||
coverage: none
|
||||
|
||||
- name: Get composer cache directory
|
||||
id: composer-cache
|
||||
run: echo "::set-output name=dir::$(composer config cache-files-dir)"
|
||||
|
||||
- name: Cache composer dependencies
|
||||
uses: actions/cache@v2
|
||||
with:
|
||||
path: ${{ steps.composer-cache.outputs.dir }}
|
||||
key: ${{ runner.os }}-composer-${{ hashFiles('**/composer.lock') }}
|
||||
restore-keys: ${{ runner.os }}-composer-
|
||||
|
||||
- name: Install dependencies
|
||||
run: composer install --no-progress --prefer-dist --optimize-autoloader ${{ steps.composer-lock.outputs.flags }}
|
||||
|
||||
- name: Setup problem matchers for PHP
|
||||
run: echo "::add-matcher::${{ runner.tool_cache }}/php.json"
|
||||
|
||||
- name: Setup problem matchers for PHPUnit
|
||||
run: echo "::add-matcher::${{ runner.tool_cache }}/phpunit.json"
|
||||
|
||||
- name: Test with PHPUnit
|
||||
run: ./vendor/bin/phpunit
|
||||
|
||||
phpcs:
|
||||
runs-on: ubuntu-latest
|
||||
steps:
|
||||
- name: Checkout
|
||||
uses: actions/checkout@v2
|
||||
|
||||
- name: Setup PHP, with composer and extensions
|
||||
uses: shivammathur/setup-php@v2
|
||||
with:
|
||||
php-version: 7.4
|
||||
extensions: ctype, dom, gd, iconv, fileinfo, libxml, mbstring, simplexml, xml, xmlreader, xmlwriter, zip, zlib
|
||||
coverage: none
|
||||
tools: cs2pr
|
||||
|
||||
- name: Get composer cache directory
|
||||
id: composer-cache
|
||||
run: echo "::set-output name=dir::$(composer config cache-files-dir)"
|
||||
|
||||
- name: Cache composer dependencies
|
||||
uses: actions/cache@v2
|
||||
with:
|
||||
path: ${{ steps.composer-cache.outputs.dir }}
|
||||
key: ${{ runner.os }}-composer-${{ hashFiles('**/composer.lock') }}
|
||||
restore-keys: ${{ runner.os }}-composer-
|
||||
|
||||
- name: Install dependencies
|
||||
run: composer install --no-progress --prefer-dist --optimize-autoloader
|
||||
|
||||
- name: Code style with PHP_CodeSniffer
|
||||
run: ./vendor/bin/phpcs -q --report=checkstyle | cs2pr --graceful-warnings --colorize
|
||||
|
||||
coverage:
|
||||
runs-on: ubuntu-latest
|
||||
steps:
|
||||
- name: Checkout
|
||||
uses: actions/checkout@v2
|
||||
|
||||
- name: Setup PHP, with composer and extensions
|
||||
uses: shivammathur/setup-php@v2
|
||||
with:
|
||||
php-version: 7.4
|
||||
extensions: ctype, dom, gd, iconv, fileinfo, libxml, mbstring, simplexml, xml, xmlreader, xmlwriter, zip, zlib
|
||||
coverage: pcov
|
||||
|
||||
- name: Get composer cache directory
|
||||
id: composer-cache
|
||||
run: echo "::set-output name=dir::$(composer config cache-files-dir)"
|
||||
|
||||
- name: Cache composer dependencies
|
||||
uses: actions/cache@v2
|
||||
with:
|
||||
path: ${{ steps.composer-cache.outputs.dir }}
|
||||
key: ${{ runner.os }}-composer-${{ hashFiles('**/composer.lock') }}
|
||||
restore-keys: ${{ runner.os }}-composer-
|
||||
|
||||
- name: Install dependencies
|
||||
run: composer install --no-progress --prefer-dist --optimize-autoloader
|
||||
|
||||
- name: Coverage
|
||||
run: |
|
||||
./vendor/bin/phpunit --coverage-text
|
@ -0,0 +1,207 @@
|
||||
PHPMatrix
|
||||
==========
|
||||
|
||||
---
|
||||
|
||||
PHP Class for handling Matrices
|
||||
|
||||
[](http://travis-ci.org/MarkBaker/PHPMatrix)
|
||||
|
||||
[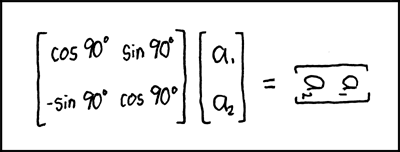](https://xkcd.com/184/)
|
||||
|
||||
Matrix Transform
|
||||
|
||||
---
|
||||
|
||||
This library currently provides the following operations:
|
||||
|
||||
- addition
|
||||
- direct sum
|
||||
- subtraction
|
||||
- multiplication
|
||||
- division (using [A].[B]<sup>-1</sup>)
|
||||
- division by
|
||||
- division into
|
||||
|
||||
together with functions for
|
||||
|
||||
- adjoint
|
||||
- antidiagonal
|
||||
- cofactors
|
||||
- determinant
|
||||
- diagonal
|
||||
- identity
|
||||
- inverse
|
||||
- minors
|
||||
- trace
|
||||
- transpose
|
||||
- solve
|
||||
|
||||
Given Matrices A and B, calculate X for A.X = B
|
||||
|
||||
and classes for
|
||||
|
||||
- Decomposition
|
||||
- LU Decomposition with partial row pivoting,
|
||||
|
||||
such that [P].[A] = [L].[U] and [A] = [P]<sup>|</sup>.[L].[U]
|
||||
- QR Decomposition
|
||||
|
||||
such that [A] = [Q].[R]
|
||||
|
||||
## TO DO
|
||||
|
||||
- power() function
|
||||
- Decomposition
|
||||
- Cholesky Decomposition
|
||||
- EigenValue Decomposition
|
||||
- EigenValues
|
||||
- EigenVectors
|
||||
|
||||
---
|
||||
|
||||
# Usage
|
||||
|
||||
To create a new Matrix object, provide an array as the constructor argument
|
||||
|
||||
```php
|
||||
$grid = [
|
||||
[16, 3, 2, 13],
|
||||
[ 5, 10, 11, 8],
|
||||
[ 9, 6, 7, 12],
|
||||
[ 4, 15, 14, 1],
|
||||
];
|
||||
|
||||
$matrix = new Matrix\Matrix($grid);
|
||||
```
|
||||
The `Builder` class provides helper methods for creating specific matrices, specifically an identity matrix of a specified size; or a matrix of a specified dimensions, with every cell containing a set value.
|
||||
```php
|
||||
$matrix = Matrix\Builder::createFilledMatrix(1, 5, 3);
|
||||
```
|
||||
Will create a matrix of 5 rows and 3 columns, filled with a `1` in every cell; while
|
||||
```php
|
||||
$matrix = Matrix\Builder::createIdentityMatrix(3);
|
||||
```
|
||||
will create a 3x3 identity matrix.
|
||||
|
||||
|
||||
Matrix objects are immutable: whenever you call a method or pass a grid to a function that returns a matrix value, a new Matrix object will be returned, and the original will remain unchanged. This also allows you to chain multiple methods as you would for a fluent interface (as long as they are methods that will return a Matrix result).
|
||||
|
||||
## Performing Mathematical Operations
|
||||
|
||||
To perform mathematical operations with Matrices, you can call the appropriate method against a matrix value, passing other values as arguments
|
||||
|
||||
```php
|
||||
$matrix1 = new Matrix\Matrix([
|
||||
[2, 7, 6],
|
||||
[9, 5, 1],
|
||||
[4, 3, 8],
|
||||
]);
|
||||
$matrix2 = new Matrix\Matrix([
|
||||
[1, 2, 3],
|
||||
[4, 5, 6],
|
||||
[7, 8, 9],
|
||||
]);
|
||||
|
||||
var_dump($matrix1->multiply($matrix2)->toArray());
|
||||
```
|
||||
or pass all values to the appropriate function
|
||||
```php
|
||||
$matrix1 = new Matrix\Matrix([
|
||||
[2, 7, 6],
|
||||
[9, 5, 1],
|
||||
[4, 3, 8],
|
||||
]);
|
||||
$matrix2 = new Matrix\Matrix([
|
||||
[1, 2, 3],
|
||||
[4, 5, 6],
|
||||
[7, 8, 9],
|
||||
]);
|
||||
|
||||
var_dump(Matrix\multiply($matrix1, $matrix2)->toArray());
|
||||
```
|
||||
You can pass in the arguments as Matrix objects, or as arrays.
|
||||
|
||||
If you want to perform the same operation against multiple values (e.g. to add three or more matrices), then you can pass multiple arguments to any of the operations.
|
||||
|
||||
## Using functions
|
||||
|
||||
When calling any of the available functions for a matrix value, you can either call the relevant method for the Matrix object
|
||||
```php
|
||||
$grid = [
|
||||
[16, 3, 2, 13],
|
||||
[ 5, 10, 11, 8],
|
||||
[ 9, 6, 7, 12],
|
||||
[ 4, 15, 14, 1],
|
||||
];
|
||||
|
||||
$matrix = new Matrix\Matrix($grid);
|
||||
|
||||
echo $matrix->trace();
|
||||
```
|
||||
or you can call the function as you would in procedural code, passing the Matrix object as an argument
|
||||
```php
|
||||
$grid = [
|
||||
[16, 3, 2, 13],
|
||||
[ 5, 10, 11, 8],
|
||||
[ 9, 6, 7, 12],
|
||||
[ 4, 15, 14, 1],
|
||||
];
|
||||
|
||||
$matrix = new Matrix\Matrix($grid);
|
||||
echo Matrix\trace($matrix);
|
||||
```
|
||||
When called procedurally using the function, you can pass in the argument as a Matrix object, or as an array.
|
||||
```php
|
||||
$grid = [
|
||||
[16, 3, 2, 13],
|
||||
[ 5, 10, 11, 8],
|
||||
[ 9, 6, 7, 12],
|
||||
[ 4, 15, 14, 1],
|
||||
];
|
||||
|
||||
echo Matrix\trace($grid);
|
||||
```
|
||||
As an alternative, it is also possible to call the method directly from the `Functions` class.
|
||||
```php
|
||||
$grid = [
|
||||
[16, 3, 2, 13],
|
||||
[ 5, 10, 11, 8],
|
||||
[ 9, 6, 7, 12],
|
||||
[ 4, 15, 14, 1],
|
||||
];
|
||||
|
||||
$matrix = new Matrix\Matrix($grid);
|
||||
echo Matrix\Functions::trace($matrix);
|
||||
```
|
||||
Used this way, methods must be called statically, and the argument must be the Matrix object, and cannot be an array.
|
||||
|
||||
## Decomposition
|
||||
|
||||
The library also provides classes for matrix decomposition. You can access these using
|
||||
```php
|
||||
$grid = [
|
||||
[1, 2],
|
||||
[3, 4],
|
||||
];
|
||||
|
||||
$matrix = new Matrix\Matrix($grid);
|
||||
|
||||
$decomposition = new Matrix\Decomposition\QR($matrix);
|
||||
$Q = $decomposition->getQ();
|
||||
$R = $decomposition->getR();
|
||||
```
|
||||
|
||||
or alternatively us the `Decomposition` factory, identifying which form of decomposition you want to use
|
||||
```php
|
||||
$grid = [
|
||||
[1, 2],
|
||||
[3, 4],
|
||||
];
|
||||
|
||||
$matrix = new Matrix\Matrix($grid);
|
||||
|
||||
$decomposition = Matrix\Decomposition\Decomposition::decomposition(Matrix\Decomposition\Decomposition::QR, $matrix);
|
||||
$Q = $decomposition->getQ();
|
||||
$R = $decomposition->getR();
|
||||
```
|
@ -0,0 +1,62 @@
|
||||
<?php
|
||||
|
||||
# required: PHP 5.3+ and zlib extension
|
||||
|
||||
// ini option check
|
||||
if (ini_get('phar.readonly')) {
|
||||
echo "php.ini: set the 'phar.readonly' option to 0 to enable phar creation\n";
|
||||
exit(1);
|
||||
}
|
||||
|
||||
// output name
|
||||
$pharName = 'Matrix.phar';
|
||||
|
||||
// target folder
|
||||
$sourceDir = __DIR__ . DIRECTORY_SEPARATOR . 'classes' . DIRECTORY_SEPARATOR . 'src' . DIRECTORY_SEPARATOR;
|
||||
|
||||
// default meta information
|
||||
$metaData = array(
|
||||
'Author' => 'Mark Baker <mark@lange.demon.co.uk>',
|
||||
'Description' => 'PHP Class for working with Matrix numbers',
|
||||
'Copyright' => 'Mark Baker (c) 2013-' . date('Y'),
|
||||
'Timestamp' => time(),
|
||||
'Version' => '0.1.0',
|
||||
'Date' => date('Y-m-d')
|
||||
);
|
||||
|
||||
// cleanup
|
||||
if (file_exists($pharName)) {
|
||||
echo "Removed: {$pharName}\n";
|
||||
unlink($pharName);
|
||||
}
|
||||
|
||||
echo "Building phar file...\n";
|
||||
|
||||
// the phar object
|
||||
$phar = new Phar($pharName, null, 'Matrix');
|
||||
$phar->buildFromDirectory($sourceDir);
|
||||
$phar->setStub(
|
||||
<<<'EOT'
|
||||
<?php
|
||||
spl_autoload_register(function ($className) {
|
||||
include 'phar://' . $className . '.php';
|
||||
});
|
||||
|
||||
try {
|
||||
Phar::mapPhar();
|
||||
} catch (PharException $e) {
|
||||
error_log($e->getMessage());
|
||||
exit(1);
|
||||
}
|
||||
|
||||
include 'phar://functions/sqrt.php';
|
||||
|
||||
__HALT_COMPILER();
|
||||
EOT
|
||||
);
|
||||
$phar->setMetadata($metaData);
|
||||
$phar->compressFiles(Phar::GZ);
|
||||
|
||||
echo "Complete.\n";
|
||||
|
||||
exit();
|
@ -0,0 +1,70 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Class for the creating "special" Matrices
|
||||
*
|
||||
* @copyright Copyright (c) 2018 Mark Baker (https://github.com/MarkBaker/PHPMatrix)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
|
||||
namespace Matrix;
|
||||
|
||||
/**
|
||||
* Matrix Builder class.
|
||||
*
|
||||
* @package Matrix
|
||||
*/
|
||||
class Builder
|
||||
{
|
||||
/**
|
||||
* Create a new matrix of specified dimensions, and filled with a specified value
|
||||
* If the column argument isn't provided, then a square matrix will be created
|
||||
*
|
||||
* @param mixed $value
|
||||
* @param int $rows
|
||||
* @param int|null $columns
|
||||
* @return Matrix
|
||||
* @throws Exception
|
||||
*/
|
||||
public static function createFilledMatrix($value, $rows, $columns = null)
|
||||
{
|
||||
if ($columns === null) {
|
||||
$columns = $rows;
|
||||
}
|
||||
|
||||
$rows = Matrix::validateRow($rows);
|
||||
$columns = Matrix::validateColumn($columns);
|
||||
|
||||
return new Matrix(
|
||||
array_fill(
|
||||
0,
|
||||
$rows,
|
||||
array_fill(
|
||||
0,
|
||||
$columns,
|
||||
$value
|
||||
)
|
||||
)
|
||||
);
|
||||
}
|
||||
|
||||
/**
|
||||
* Create a new identity matrix of specified dimensions
|
||||
* This will always be a square matrix, with the number of rows and columns matching the provided dimension
|
||||
*
|
||||
* @param int $dimensions
|
||||
* @return Matrix
|
||||
* @throws Exception
|
||||
*/
|
||||
public static function createIdentityMatrix($dimensions)
|
||||
{
|
||||
$grid = static::createFilledMatrix(null, $dimensions)->toArray();
|
||||
|
||||
for ($x = 0; $x < $dimensions; ++$x) {
|
||||
$grid[$x][$x] = 1;
|
||||
}
|
||||
|
||||
return new Matrix($grid);
|
||||
}
|
||||
}
|
@ -0,0 +1,27 @@
|
||||
<?php
|
||||
|
||||
namespace Matrix\Decomposition;
|
||||
|
||||
use Matrix\Exception;
|
||||
use Matrix\Matrix;
|
||||
|
||||
class Decomposition
|
||||
{
|
||||
const LU = 'LU';
|
||||
const QR = 'QR';
|
||||
|
||||
/**
|
||||
* @throws Exception
|
||||
*/
|
||||
public static function decomposition($type, Matrix $matrix)
|
||||
{
|
||||
switch (strtoupper($type)) {
|
||||
case self::LU:
|
||||
return new LU($matrix);
|
||||
case self::QR:
|
||||
return new QR($matrix);
|
||||
default:
|
||||
throw new Exception('Invalid Decomposition');
|
||||
}
|
||||
}
|
||||
}
|
@ -0,0 +1,260 @@
|
||||
<?php
|
||||
|
||||
namespace Matrix\Decomposition;
|
||||
|
||||
use Matrix\Exception;
|
||||
use Matrix\Matrix;
|
||||
|
||||
class LU
|
||||
{
|
||||
private $luMatrix;
|
||||
private $rows;
|
||||
private $columns;
|
||||
|
||||
private $pivot = [];
|
||||
|
||||
public function __construct(Matrix $matrix)
|
||||
{
|
||||
$this->luMatrix = $matrix->toArray();
|
||||
$this->rows = $matrix->rows;
|
||||
$this->columns = $matrix->columns;
|
||||
|
||||
$this->buildPivot();
|
||||
}
|
||||
|
||||
/**
|
||||
* Get lower triangular factor.
|
||||
*
|
||||
* @return Matrix Lower triangular factor
|
||||
*/
|
||||
public function getL()
|
||||
{
|
||||
$lower = [];
|
||||
|
||||
$columns = min($this->rows, $this->columns);
|
||||
for ($row = 0; $row < $this->rows; ++$row) {
|
||||
for ($column = 0; $column < $columns; ++$column) {
|
||||
if ($row > $column) {
|
||||
$lower[$row][$column] = $this->luMatrix[$row][$column];
|
||||
} elseif ($row === $column) {
|
||||
$lower[$row][$column] = 1.0;
|
||||
} else {
|
||||
$lower[$row][$column] = 0.0;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
return new Matrix($lower);
|
||||
}
|
||||
|
||||
/**
|
||||
* Get upper triangular factor.
|
||||
*
|
||||
* @return Matrix Upper triangular factor
|
||||
*/
|
||||
public function getU()
|
||||
{
|
||||
$upper = [];
|
||||
|
||||
$rows = min($this->rows, $this->columns);
|
||||
for ($row = 0; $row < $rows; ++$row) {
|
||||
for ($column = 0; $column < $this->columns; ++$column) {
|
||||
if ($row <= $column) {
|
||||
$upper[$row][$column] = $this->luMatrix[$row][$column];
|
||||
} else {
|
||||
$upper[$row][$column] = 0.0;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
return new Matrix($upper);
|
||||
}
|
||||
|
||||
/**
|
||||
* Return pivot permutation vector.
|
||||
*
|
||||
* @return Matrix Pivot matrix
|
||||
*/
|
||||
public function getP()
|
||||
{
|
||||
$pMatrix = [];
|
||||
|
||||
$pivots = $this->pivot;
|
||||
$pivotCount = count($pivots);
|
||||
foreach ($pivots as $row => $pivot) {
|
||||
$pMatrix[$row] = array_fill(0, $pivotCount, 0);
|
||||
$pMatrix[$row][$pivot] = 1;
|
||||
}
|
||||
|
||||
return new Matrix($pMatrix);
|
||||
}
|
||||
|
||||
/**
|
||||
* Return pivot permutation vector.
|
||||
*
|
||||
* @return array Pivot vector
|
||||
*/
|
||||
public function getPivot()
|
||||
{
|
||||
return $this->pivot;
|
||||
}
|
||||
|
||||
/**
|
||||
* Is the matrix nonsingular?
|
||||
*
|
||||
* @return bool true if U, and hence A, is nonsingular
|
||||
*/
|
||||
public function isNonsingular()
|
||||
{
|
||||
for ($diagonal = 0; $diagonal < $this->columns; ++$diagonal) {
|
||||
if ($this->luMatrix[$diagonal][$diagonal] === 0.0) {
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
return true;
|
||||
}
|
||||
|
||||
private function buildPivot()
|
||||
{
|
||||
for ($row = 0; $row < $this->rows; ++$row) {
|
||||
$this->pivot[$row] = $row;
|
||||
}
|
||||
|
||||
for ($column = 0; $column < $this->columns; ++$column) {
|
||||
$luColumn = $this->localisedReferenceColumn($column);
|
||||
|
||||
$this->applyTransformations($column, $luColumn);
|
||||
|
||||
$pivot = $this->findPivot($column, $luColumn);
|
||||
if ($pivot !== $column) {
|
||||
$this->pivotExchange($pivot, $column);
|
||||
}
|
||||
|
||||
$this->computeMultipliers($column);
|
||||
|
||||
unset($luColumn);
|
||||
}
|
||||
}
|
||||
|
||||
private function localisedReferenceColumn($column)
|
||||
{
|
||||
$luColumn = [];
|
||||
|
||||
for ($row = 0; $row < $this->rows; ++$row) {
|
||||
$luColumn[$row] = &$this->luMatrix[$row][$column];
|
||||
}
|
||||
|
||||
return $luColumn;
|
||||
}
|
||||
|
||||
private function applyTransformations($column, array $luColumn)
|
||||
{
|
||||
for ($row = 0; $row < $this->rows; ++$row) {
|
||||
$luRow = $this->luMatrix[$row];
|
||||
// Most of the time is spent in the following dot product.
|
||||
$kmax = min($row, $column);
|
||||
$sValue = 0.0;
|
||||
for ($kValue = 0; $kValue < $kmax; ++$kValue) {
|
||||
$sValue += $luRow[$kValue] * $luColumn[$kValue];
|
||||
}
|
||||
$luRow[$column] = $luColumn[$row] -= $sValue;
|
||||
}
|
||||
}
|
||||
|
||||
private function findPivot($column, array $luColumn)
|
||||
{
|
||||
$pivot = $column;
|
||||
for ($row = $column + 1; $row < $this->rows; ++$row) {
|
||||
if (abs($luColumn[$row]) > abs($luColumn[$pivot])) {
|
||||
$pivot = $row;
|
||||
}
|
||||
}
|
||||
|
||||
return $pivot;
|
||||
}
|
||||
|
||||
private function pivotExchange($pivot, $column)
|
||||
{
|
||||
for ($kValue = 0; $kValue < $this->columns; ++$kValue) {
|
||||
$tValue = $this->luMatrix[$pivot][$kValue];
|
||||
$this->luMatrix[$pivot][$kValue] = $this->luMatrix[$column][$kValue];
|
||||
$this->luMatrix[$column][$kValue] = $tValue;
|
||||
}
|
||||
|
||||
$lValue = $this->pivot[$pivot];
|
||||
$this->pivot[$pivot] = $this->pivot[$column];
|
||||
$this->pivot[$column] = $lValue;
|
||||
}
|
||||
|
||||
private function computeMultipliers($diagonal)
|
||||
{
|
||||
if (($diagonal < $this->rows) && ($this->luMatrix[$diagonal][$diagonal] != 0.0)) {
|
||||
for ($row = $diagonal + 1; $row < $this->rows; ++$row) {
|
||||
$this->luMatrix[$row][$diagonal] /= $this->luMatrix[$diagonal][$diagonal];
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
private function pivotB(Matrix $B)
|
||||
{
|
||||
$X = [];
|
||||
foreach ($this->pivot as $rowId) {
|
||||
$row = $B->getRows($rowId + 1)->toArray();
|
||||
$X[] = array_pop($row);
|
||||
}
|
||||
|
||||
return $X;
|
||||
}
|
||||
|
||||
/**
|
||||
* Solve A*X = B.
|
||||
*
|
||||
* @param Matrix $B a Matrix with as many rows as A and any number of columns
|
||||
*
|
||||
* @throws Exception
|
||||
*
|
||||
* @return Matrix X so that L*U*X = B(piv,:)
|
||||
*/
|
||||
public function solve(Matrix $B)
|
||||
{
|
||||
if ($B->rows !== $this->rows) {
|
||||
throw new Exception('Matrix row dimensions are not equal');
|
||||
}
|
||||
|
||||
if ($this->rows !== $this->columns) {
|
||||
throw new Exception('LU solve() only works on square matrices');
|
||||
}
|
||||
|
||||
if (!$this->isNonsingular()) {
|
||||
throw new Exception('Can only perform operation on singular matrix');
|
||||
}
|
||||
|
||||
// Copy right hand side with pivoting
|
||||
$nx = $B->columns;
|
||||
$X = $this->pivotB($B);
|
||||
|
||||
// Solve L*Y = B(piv,:)
|
||||
for ($k = 0; $k < $this->columns; ++$k) {
|
||||
for ($i = $k + 1; $i < $this->columns; ++$i) {
|
||||
for ($j = 0; $j < $nx; ++$j) {
|
||||
$X[$i][$j] -= $X[$k][$j] * $this->luMatrix[$i][$k];
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
// Solve U*X = Y;
|
||||
for ($k = $this->columns - 1; $k >= 0; --$k) {
|
||||
for ($j = 0; $j < $nx; ++$j) {
|
||||
$X[$k][$j] /= $this->luMatrix[$k][$k];
|
||||
}
|
||||
for ($i = 0; $i < $k; ++$i) {
|
||||
for ($j = 0; $j < $nx; ++$j) {
|
||||
$X[$i][$j] -= $X[$k][$j] * $this->luMatrix[$i][$k];
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
return new Matrix($X);
|
||||
}
|
||||
}
|
@ -0,0 +1,194 @@
|
||||
<?php
|
||||
|
||||
namespace Matrix\Decomposition;
|
||||
|
||||
use Matrix\Exception;
|
||||
use Matrix\Matrix;
|
||||
|
||||
class QR
|
||||
{
|
||||
private $qrMatrix;
|
||||
private $rows;
|
||||
private $columns;
|
||||
|
||||
private $rDiagonal = [];
|
||||
|
||||
public function __construct(Matrix $matrix)
|
||||
{
|
||||
$this->qrMatrix = $matrix->toArray();
|
||||
$this->rows = $matrix->rows;
|
||||
$this->columns = $matrix->columns;
|
||||
|
||||
$this->decompose();
|
||||
}
|
||||
|
||||
public function getHouseholdVectors()
|
||||
{
|
||||
$householdVectors = [];
|
||||
for ($row = 0; $row < $this->rows; ++$row) {
|
||||
for ($column = 0; $column < $this->columns; ++$column) {
|
||||
if ($row >= $column) {
|
||||
$householdVectors[$row][$column] = $this->qrMatrix[$row][$column];
|
||||
} else {
|
||||
$householdVectors[$row][$column] = 0.0;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
return new Matrix($householdVectors);
|
||||
}
|
||||
|
||||
public function getQ()
|
||||
{
|
||||
$qGrid = [];
|
||||
|
||||
$rowCount = $this->rows;
|
||||
for ($k = $this->columns - 1; $k >= 0; --$k) {
|
||||
for ($i = 0; $i < $this->rows; ++$i) {
|
||||
$qGrid[$i][$k] = 0.0;
|
||||
}
|
||||
$qGrid[$k][$k] = 1.0;
|
||||
if ($this->columns > $this->rows) {
|
||||
$qGrid = array_slice($qGrid, 0, $this->rows);
|
||||
}
|
||||
|
||||
for ($j = $k; $j < $this->columns; ++$j) {
|
||||
if (isset($this->qrMatrix[$k], $this->qrMatrix[$k][$k]) && $this->qrMatrix[$k][$k] != 0.0) {
|
||||
$s = 0.0;
|
||||
for ($i = $k; $i < $this->rows; ++$i) {
|
||||
$s += $this->qrMatrix[$i][$k] * $qGrid[$i][$j];
|
||||
}
|
||||
$s = -$s / $this->qrMatrix[$k][$k];
|
||||
for ($i = $k; $i < $this->rows; ++$i) {
|
||||
$qGrid[$i][$j] += $s * $this->qrMatrix[$i][$k];
|
||||
}
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
array_walk(
|
||||
$qGrid,
|
||||
function (&$row) use ($rowCount) {
|
||||
$row = array_reverse($row);
|
||||
$row = array_slice($row, 0, $rowCount);
|
||||
}
|
||||
);
|
||||
|
||||
return new Matrix($qGrid);
|
||||
}
|
||||
|
||||
public function getR()
|
||||
{
|
||||
$rGrid = [];
|
||||
|
||||
for ($row = 0; $row < $this->columns; ++$row) {
|
||||
for ($column = 0; $column < $this->columns; ++$column) {
|
||||
if ($row < $column) {
|
||||
$rGrid[$row][$column] = isset($this->qrMatrix[$row][$column]) ? $this->qrMatrix[$row][$column] : 0.0;
|
||||
} elseif ($row === $column) {
|
||||
$rGrid[$row][$column] = isset($this->rDiagonal[$row]) ? $this->rDiagonal[$row] : 0.0;
|
||||
} else {
|
||||
$rGrid[$row][$column] = 0.0;
|
||||
}
|
||||
}
|
||||
}
|
||||
|
||||
if ($this->columns > $this->rows) {
|
||||
$rGrid = array_slice($rGrid, 0, $this->rows);
|
||||
}
|
||||
|
||||
return new Matrix($rGrid);
|
||||
}
|
||||
|
||||
private function hypo($a, $b)
|
||||
{
|
||||
if (abs($a) > abs($b)) {
|
||||
$r = $b / $a;
|
||||
$r = abs($a) * sqrt(1 + $r * $r);
|
||||
} elseif ($b != 0.0) {
|
||||
$r = $a / $b;
|
||||
$r = abs($b) * sqrt(1 + $r * $r);
|
||||
} else {
|
||||
$r = 0.0;
|
||||
}
|
||||
|
||||
return $r;
|
||||
}
|
||||
|
||||
/**
|
||||
* QR Decomposition computed by Householder reflections.
|
||||
*/
|
||||
private function decompose()
|
||||
{
|
||||
for ($k = 0; $k < $this->columns; ++$k) {
|
||||
// Compute 2-norm of k-th column without under/overflow.
|
||||
$norm = 0.0;
|
||||
for ($i = $k; $i < $this->rows; ++$i) {
|
||||
$norm = $this->hypo($norm, $this->qrMatrix[$i][$k]);
|
||||
}
|
||||
if ($norm != 0.0) {
|
||||
// Form k-th Householder vector.
|
||||
if ($this->qrMatrix[$k][$k] < 0.0) {
|
||||
$norm = -$norm;
|
||||
}
|
||||
for ($i = $k; $i < $this->rows; ++$i) {
|
||||
$this->qrMatrix[$i][$k] /= $norm;
|
||||
}
|
||||
$this->qrMatrix[$k][$k] += 1.0;
|
||||
// Apply transformation to remaining columns.
|
||||
for ($j = $k + 1; $j < $this->columns; ++$j) {
|
||||
$s = 0.0;
|
||||
for ($i = $k; $i < $this->rows; ++$i) {
|
||||
$s += $this->qrMatrix[$i][$k] * $this->qrMatrix[$i][$j];
|
||||
}
|
||||
$s = -$s / $this->qrMatrix[$k][$k];
|
||||
for ($i = $k; $i < $this->rows; ++$i) {
|
||||
$this->qrMatrix[$i][$j] += $s * $this->qrMatrix[$i][$k];
|
||||
}
|
||||
}
|
||||
}
|
||||
$this->rDiagonal[$k] = -$norm;
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* @return bool
|
||||
*/
|
||||
public function isFullRank()
|
||||
{
|
||||
for ($j = 0; $j < $this->columns; ++$j) {
|
||||
if ($this->rDiagonal[$j] == 0.0) {
|
||||
return false;
|
||||
}
|
||||
}
|
||||
|
||||
return true;
|
||||
}
|
||||
|
||||
/**
|
||||
* Least squares solution of A*X = B.
|
||||
*
|
||||
* @param Matrix $B a Matrix with as many rows as A and any number of columns
|
||||
*
|
||||
* @throws Exception
|
||||
*
|
||||
* @return Matrix matrix that minimizes the two norm of Q*R*X-B
|
||||
*/
|
||||
public function solve(Matrix $B)
|
||||
{
|
||||
if ($B->rows !== $this->rows) {
|
||||
throw new Exception('Matrix row dimensions are not equal');
|
||||
}
|
||||
|
||||
if (!$this->isFullRank()) {
|
||||
throw new Exception('Can only perform this operation on a full-rank matrix');
|
||||
}
|
||||
|
||||
// Compute Y = transpose(Q)*B
|
||||
$Y = $this->getQ()->transpose()
|
||||
->multiply($B);
|
||||
// Solve R*X = Y;
|
||||
return $this->getR()->inverse()
|
||||
->multiply($Y);
|
||||
}
|
||||
}
|
@ -0,0 +1,13 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
* Exception.
|
||||
*
|
||||
* @copyright Copyright (c) 2013-2018 Mark Baker (https://github.com/MarkBaker/PHPMatrix)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
namespace Matrix;
|
||||
|
||||
class Exception extends \Exception
|
||||
{
|
||||
}
|
@ -0,0 +1,423 @@
|
||||
<?php
|
||||
|
||||
/**
|
||||
*
|
||||
* Class for the management of Matrices
|
||||
*
|
||||
* @copyright Copyright (c) 2018 Mark Baker (https://github.com/MarkBaker/PHPMatrix)
|
||||
* @license https://opensource.org/licenses/MIT MIT
|
||||
*/
|
||||
|
||||
namespace Matrix;
|
||||
|
||||
use Generator;
|
||||
use Matrix\Decomposition\LU;
|
||||
use Matrix\Decomposition\QR;
|
||||
|
||||
/**
|
||||
* Matrix object.
|
||||
*
|
||||
* @package Matrix
|
||||
*
|
||||
* @property-read int $rows The number of rows in the matrix
|
||||
* @property-read int $columns The number of columns in the matrix
|
||||
* @method Matrix antidiagonal()
|
||||
* @method Matrix adjoint()
|
||||
* @method Matrix cofactors()
|
||||
* @method float determinant()
|
||||
* @method Matrix diagonal()
|
||||
* @method Matrix identity()
|
||||
* @method Matrix inverse()
|
||||
* @method Matrix pseudoInverse()
|
||||
* @method Matrix minors()
|
||||
* @method float trace()
|
||||
* @method Matrix transpose()
|
||||
* @method Matrix add(...$matrices)
|
||||
* @method Matrix subtract(...$matrices)
|
||||
* @method Matrix multiply(...$matrices)
|
||||
* @method Matrix divideby(...$matrices)
|
||||
* @method Matrix divideinto(...$matrices)
|
||||
* @method Matrix directsum(...$matrices)
|
||||
*/
|
||||
class Matrix
|
||||
{
|
||||
protected $rows;
|
||||
protected $columns;
|
||||
protected $grid = [];
|
||||
|
||||
/*
|
||||
* Create a new Matrix object from an array of values
|
||||
*
|
||||
* @param array $grid
|
||||
*/
|
||||
final public function __construct(array $grid)
|
||||
{
|
||||
$this->buildFromArray(array_values($grid));
|
||||
}
|
||||
|
||||
/*
|
||||
* Create a new Matrix object from an array of values
|
||||
*
|
||||
* @param array $grid
|
||||
*/
|
||||
protected function buildFromArray(array $grid)
|
||||
{
|
||||
$this->rows = count($grid);
|
||||
$columns = array_reduce(
|
||||
$grid,
|
||||
function ($carry, $value) {
|
||||
return max($carry, is_array($value) ? count($value) : 1);
|
||||
}
|
||||
);
|
||||
$this->columns = $columns;
|
||||
|
||||
array_walk(
|
||||
$grid,
|
||||
function (&$value) use ($columns) {
|
||||
if (!is_array($value)) {
|
||||
$value = [$value];
|
||||
}
|
||||
$value = array_pad(array_values($value), $columns, null);
|
||||
}
|
||||
);
|
||||
|
||||
$this->grid = $grid;
|
||||
}
|
||||
|
||||
/**
|
||||
* Validate that a row number is a positive integer
|
||||
*
|
||||
* @param int $row
|
||||
* @return int
|
||||
* @throws Exception
|
||||
*/
|
||||
public static function validateRow($row)
|
||||
{
|
||||
if ((!is_numeric($row)) || (intval($row) < 1)) {
|
||||
throw new Exception('Invalid Row');
|
||||
}
|
||||
|
||||
return (int)$row;
|
||||
}
|
||||
|
||||
/**
|
||||
* Validate that a column number is a positive integer
|
||||
*
|
||||
* @param int $column
|
||||
* @return int
|
||||
* @throws Exception
|
||||
*/
|
||||
public static function validateColumn($column)
|
||||
{
|
||||
if ((!is_numeric($column)) || (intval($column) < 1)) {
|
||||
throw new Exception('Invalid Column');
|
||||
}
|
||||
|
||||
return (int)$column;
|
||||
}
|
||||
|
||||
/**
|
||||
* Validate that a row number falls within the set of rows for this matrix
|
||||
*
|
||||
* @param int $row
|
||||
* @return int
|
||||
* @throws Exception
|
||||
*/
|
||||
protected function validateRowInRange($row)
|
||||
{
|
||||
$row = static::validateRow($row);
|
||||
if ($row > $this->rows) {
|
||||
throw new Exception('Requested Row exceeds matrix size');
|
||||
}
|
||||
|
||||
return $row;
|
||||
}
|
||||
|
||||
/**
|
||||
* Validate that a column number falls within the set of columns for this matrix
|
||||
*
|
||||
* @param int $column
|
||||
* @return int
|
||||
* @throws Exception
|
||||
*/
|
||||
protected function validateColumnInRange($column)
|
||||
{
|
||||
$column = static::validateColumn($column);
|
||||
if ($column > $this->columns) {
|
||||
throw new Exception('Requested Column exceeds matrix size');
|
||||
}
|
||||
|
||||
return $column;
|
||||
}
|
||||
|
||||
/**
|
||||
* Return a new matrix as a subset of rows from this matrix, starting at row number $row, and $rowCount rows
|
||||
* A $rowCount value of 0 will return all rows of the matrix from $row
|
||||
* A negative $rowCount value will return rows until that many rows from the end of the matrix
|
||||
*
|
||||
* Note that row numbers start from 1, not from 0
|
||||
*
|
||||
* @param int $row
|
||||
* @param int $rowCount
|
||||
* @return static
|
||||
* @throws Exception
|
||||
*/
|
||||
public function getRows($row, $rowCount = 1)
|
||||
{
|
||||
$row = $this->validateRowInRange($row);
|
||||
if ($rowCount === 0) {
|
||||
$rowCount = $this->rows - $row + 1;
|
||||
}
|
||||
|
||||
return new static(array_slice($this->grid, $row - 1, (int)$rowCount));
|
||||
}
|
||||
|
||||
/**
|
||||
* Return a new matrix as a subset of columns from this matrix, starting at column number $column, and $columnCount columns
|
||||
* A $columnCount value of 0 will return all columns of the matrix from $column
|
||||
* A negative $columnCount value will return columns until that many columns from the end of the matrix
|
||||
*
|
||||
* Note that column numbers start from 1, not from 0
|
||||
*
|
||||
* @param int $column
|
||||
* @param int $columnCount
|
||||
* @return Matrix
|
||||
* @throws Exception
|
||||
*/
|
||||
public function getColumns($column, $columnCount = 1)
|
||||
{
|
||||
$column = $this->validateColumnInRange($column);
|
||||
if ($columnCount < 1) {
|
||||
$columnCount = $this->columns + $columnCount - $column + 1;
|
||||
}
|
||||
|
||||
$grid = [];
|
||||
for ($i = $column - 1; $i < $column + $columnCount - 1; ++$i) {
|
||||
$grid[] = array_column($this->grid, $i);
|
||||
}
|
||||
|
||||
return (new static($grid))->transpose();
|
||||
}
|
||||
|
||||
/**
|
||||
* Return a new matrix as a subset of rows from this matrix, dropping rows starting at row number $row,
|
||||
* and $rowCount rows
|
||||
* A negative $rowCount value will drop rows until that many rows from the end of the matrix
|
||||
* A $rowCount value of 0 will remove all rows of the matrix from $row
|
||||
*
|
||||
* Note that row numbers start from 1, not from 0
|
||||
*
|
||||
* @param int $row
|
||||
* @param int $rowCount
|
||||
* @return static
|
||||
* @throws Exception
|
||||
*/
|
||||
public function dropRows($row, $rowCount = 1)
|
||||
{
|
||||
$this->validateRowInRange($row);
|
||||
if ($rowCount === 0) {
|
||||
$rowCount = $this->rows - $row + 1;
|
||||
}
|
||||
|
||||
$grid = $this->grid;
|
||||
array_splice($grid, $row - 1, (int)$rowCount);
|
||||
|
||||
return new static($grid);
|
||||
}
|
||||
|
||||
/**
|
||||
* Return a new matrix as a subset of columns from this matrix, dropping columns starting at column number $column,
|
||||
* and $columnCount columns
|
||||
* A negative $columnCount value will drop columns until that many columns from the end of the matrix
|
||||
* A $columnCount value of 0 will remove all columns of the matrix from $column
|
||||
*
|
||||
* Note that column numbers start from 1, not from 0
|
||||
*
|
||||
* @param int $column
|
||||
* @param int $columnCount
|
||||
* @return static
|
||||
* @throws Exception
|
||||
*/
|
||||
public function dropColumns($column, $columnCount = 1)
|
||||
{
|
||||
$this->validateColumnInRange($column);
|
||||
if ($columnCount < 1) {
|
||||
$columnCount = $this->columns + $columnCount - $column + 1;
|
||||
}
|
||||
|
||||
$grid = $this->grid;
|
||||
array_walk(
|
||||
$grid,
|
||||
function (&$row) use ($column, $columnCount) {
|
||||
array_splice($row, $column - 1, (int)$columnCount);
|
||||
}
|
||||
);
|
||||
|
||||
return new static($grid);
|
||||
}
|
||||
|
||||
/**
|
||||
* Return a value from this matrix, from the "cell" identified by the row and column numbers
|
||||
* Note that row and column numbers start from 1, not from 0
|
||||
*
|
||||
* @param int $row
|
||||
* @param int $column
|
||||
* @return mixed
|
||||
* @throws Exception
|
||||
*/
|
||||
public function getValue($row, $column)
|
||||
{
|
||||
$row = $this->validateRowInRange($row);
|
||||
$column = $this->validateColumnInRange($column);
|
||||
|
||||
return $this->grid[$row - 1][$column - 1];
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns a Generator that will yield each row of the matrix in turn as a vector matrix
|
||||
* or the value of each cell if the matrix is a column vector
|
||||
*
|
||||
* @return Generator|Matrix[]|mixed[]
|
||||
*/
|
||||
public function rows()
|
||||
{
|
||||
foreach ($this->grid as $i => $row) {
|
||||
yield $i + 1 => ($this->columns == 1)
|
||||
? $row[0]
|
||||
: new static([$row]);
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Returns a Generator that will yield each column of the matrix in turn as a vector matrix
|
||||
* or the value of each cell if the matrix is a row vector
|
||||
*
|
||||
* @return Generator|Matrix[]|mixed[]
|
||||
*/
|
||||
public function columns()
|
||||
{
|
||||
for ($i = 0; $i < $this->columns; ++$i) {
|
||||
yield $i + 1 => ($this->rows == 1)
|
||||
? $this->grid[0][$i]
|
||||
: new static(array_column($this->grid, $i));
|
||||
}
|
||||
}
|
||||
|
||||
/**
|
||||
* Identify if the row and column dimensions of this matrix are equal,
|
||||
* i.e. if it is a "square" matrix
|
||||
*
|
||||
* @return bool
|
||||
*/
|
||||
public function isSquare()
|
||||
{
|
||||
return $this->rows == $this->columns;
|
||||
}
|
||||
|
||||
/**
|
||||
* Identify if this matrix is a vector
|
||||
* i.e. if it comprises only a single row or a single column
|
||||
*
|
||||
* @return bool
|
||||
*/
|
||||
public function isVector()
|
||||
{
|
||||
return $this->rows == 1 || $this->columns == 1;
|
||||
}
|
||||
|
||||
/**
|
||||
* Return the matrix as a 2-dimensional array
|
||||
*
|
||||
* @return array
|
||||
*/
|
||||
public function toArray()
|
||||
{
|
||||
return $this->grid;
|
||||
}
|
||||
|
||||
/**
|
||||
* Solve A*X = B.
|
||||
*
|
||||
* @param Matrix $B Right hand side
|
||||
*
|
||||
* @throws Exception
|
||||
*
|
||||
* @return Matrix ... Solution if A is square, least squares solution otherwise
|
||||
*/
|
||||
public function solve(Matrix $B)
|
||||
{
|
||||
if ($this->columns === $this->rows) {
|
||||
return (new LU($this))->solve($B);
|
||||
}
|
||||
|
||||
return (new QR($this))->solve($B);
|
||||
}
|
||||
|
||||
protected static $getters = [
|
||||
'rows',
|
||||
'columns',
|
||||
];
|
||||
|
||||
/**
|
||||
* Access specific properties as read-only (no setters)
|
||||
*
|
||||
* @param string $propertyName
|
||||
* @return mixed
|
||||
* @throws Exception
|
||||
*/
|
||||
public function __get($propertyName)
|
||||
{
|
||||
$propertyName = strtolower($propertyName);
|
||||
|
||||
// Test for function calls
|
||||
if (in_array($propertyName, self::$getters)) {
|
||||
return $this->$propertyName;
|
||||
}
|
||||
|
||||
throw new Exception('Property does not exist');
|
||||
}
|
||||
|
||||
protected static $functions = [
|
||||
'antidiagonal',
|
||||
'adjoint',
|
||||
'cofactors',
|
||||
'determinant',
|
||||
'diagonal',
|
||||
'identity',
|
||||
'inverse',
|
||||
'minors',
|
||||
'trace',
|
||||
'transpose',
|
||||
];
|
||||
|
||||
protected static $operations = [
|
||||
'add',
|
||||
'subtract',
|
||||
'multiply',
|
||||
'divideby',
|
||||
'divideinto',
|
||||
'directsum',
|
||||
];
|
||||
|
||||
/**
|
||||
* Returns the result of the function call or operation
|
||||
*
|
||||
* @param string $functionName
|
||||
* @param mixed[] $arguments
|
||||
* @return Matrix|float
|
||||
* @throws Exception
|
||||
*/
|
||||
public function __call($functionName, $arguments)
|
||||
{
|
||||
$functionName = strtolower(str_replace('_', '', $functionName));
|
||||
|
||||
if (in_array($functionName, self::$functions, true) || in_array($functionName, self::$operations, true)) {
|
||||
$functionName = "\\" . __NAMESPACE__ . "\\{$functionName}";
|
||||
if (is_callable($functionName)) {
|
||||
$arguments = array_values(array_merge([$this], $arguments));
|
||||
return call_user_func_array($functionName, $arguments);
|
||||
}
|
||||
}
|
||||
throw new Exception('Function or Operation does not exist');
|
||||
}
|
||||
}
|
@ -0,0 +1,68 @@
|
||||
<?php
|
||||
|
||||
namespace Matrix\Operators;
|
||||
|
||||
use Matrix\Matrix;
|
||||
use Matrix\Exception;
|
||||
|
||||
class Addition extends Operator
|
||||
{
|
||||
/**
|
||||
* Execute the addition
|
||||
*
|
||||
* @param mixed $value The matrix or numeric value to add to the current base value
|
||||
* @throws Exception If the provided argument is not appropriate for the operation
|
||||
* @return $this The operation object, allowing multiple additions to be chained
|
||||
**/
|
||||
public function execute($value)
|
||||
{
|
||||
if (is_array($value)) {
|
||||
$value = new Matrix($value);
|
||||
}
|
||||
|
||||
if (is_object($value) && ($value instanceof Matrix)) {
|
||||
return $this->addMatrix($value);
|
||||
} elseif (is_numeric($value)) {
|
||||
return $this->addScalar($value);
|
||||
}
|
||||
|
||||
throw new Exception('Invalid argument for addition');
|
||||
}
|
||||
|
||||
/**
|
||||
* Execute the addition for a scalar
|
||||
*
|
||||
* @param mixed $value The numeric value to add to the current base value
|
||||
* @return $this The operation object, allowing multiple additions to be chained
|
||||
**/
|
||||
protected function addScalar($value)
|
||||
{
|
||||
for ($row = 0; $row < $this->rows; ++$row) {
|
||||
for ($column = 0; $column < $this->columns; ++$column) {
|
||||
$this->matrix[$row][$column] += $value;
|
||||
}
|
||||
}
|
||||
|
||||
return $this;
|
||||
}
|
||||
|
||||
/**
|
||||
* Execute the addition for a matrix
|
||||
*
|
||||
* @param Matrix $value The numeric value to add to the current base value
|
||||
* @return $this The operation object, allowing multiple additions to be chained
|
||||
* @throws Exception If the provided argument is not appropriate for the operation
|
||||
**/
|
||||
protected function addMatrix(Matrix $value)
|
||||
{
|
||||
$this->validateMatchingDimensions($value);
|
||||
|
||||
for ($row = 0; $row < $this->rows; ++$row) {
|
||||
for ($column = 0; $column < $this->columns; ++$column) {
|
||||
$this->matrix[$row][$column] += $value->getValue($row + 1, $column + 1);
|
||||
}
|
||||
}
|
||||
|
||||
return $this;
|
||||
}
|
||||
}
|
@ -0,0 +1,64 @@
|
||||
<?php
|
||||
|
||||
namespace Matrix\Operators;
|
||||
|
||||
use Matrix\Matrix;
|
||||
use Matrix\Exception;
|
||||
|
||||
class DirectSum extends Operator
|
||||
{
|
||||
/**
|
||||
* Execute the addition
|
||||
*
|
||||
* @param mixed $value The matrix or numeric value to add to the current base value
|
||||
* @return $this The operation object, allowing multiple additions to be chained
|
||||
* @throws Exception If the provided argument is not appropriate for the operation
|
||||
*/
|
||||
public function execute($value)
|
||||
{
|
||||
if (is_array($value)) {
|
||||
$value = new Matrix($value);
|
||||
}
|
||||
|
||||
if ($value instanceof Matrix) {
|
||||
return $this->directSumMatrix($value);
|
||||
}
|
||||
|
||||
throw new Exception('Invalid argument for addition');
|
||||
}
|
||||
|
||||
/**
|
||||
* Execute the direct sum for a matrix
|
||||
*
|
||||
* @param Matrix $value The numeric value to concatenate/direct sum with the current base value
|
||||
* @return $this The operation object, allowing multiple additions to be chained
|
||||
**/
|
||||
private function directSumMatrix($value)
|
||||
{
|
||||
$originalColumnCount = count($this->matrix[0]);
|
||||
$originalRowCount = count($this->matrix);
|
||||
$valColumnCount = $value->columns;
|
||||
$valRowCount = $value->rows;
|
||||
$value = $value->toArray();
|
||||
|
||||
for ($row = 0; $row < $this->rows; ++$row) {
|
||||
$this->matrix[$row] = array_merge($this->matrix[$row], array_fill(0, $valColumnCount, 0));
|
||||
}
|
||||
|
||||
$this->matrix = array_merge(
|
||||
$this->matrix,
|
||||
array_fill(0, $valRowCount, array_fill(0, $originalColumnCount, 0))
|
||||
);
|
||||
|
||||
for ($row = $originalRowCount; $row < $originalRowCount + $valRowCount; ++$row) {
|
||||
array_splice(
|
||||
$this->matrix[$row],
|
||||
$originalColumnCount,
|
||||
$valColumnCount,
|
||||
$value[$row - $originalRowCount]
|
||||
);
|
||||
}
|
||||
|
||||
return $this;
|
||||
}
|
||||
}
|
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in new issue